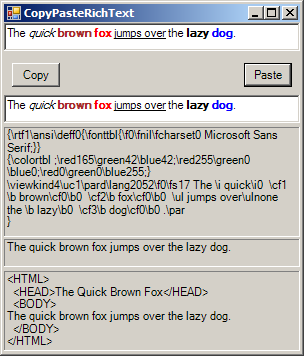
Imports System.Drawing
Imports System.Windows.Forms
public class CopyPasteRichText
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Private Sub frmDragRichText_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Dim txt As String = "The quick brown fox jumps over the lazy dog."
rchSource.Text = txt
rchSource.Select(txt.IndexOf("quick"), Len("quick"))
rchSource.SelectionFont = New Font(rchSource.SelectionFont, FontStyle.Italic)
rchSource.Select(txt.IndexOf("brown"), Len("brown"))
rchSource.SelectionFont = New Font(rchSource.SelectionFont, FontStyle.Bold)
rchSource.SelectionColor = Color.Brown
rchSource.Select(txt.IndexOf("fox"), Len("fox"))
rchSource.SelectionFont = New Font(rchSource.SelectionFont, FontStyle.Bold)
rchSource.SelectionColor = Color.Red
rchSource.Select(txt.IndexOf("jumps over"), Len("jumps over"))
rchSource.SelectionFont = New Font(rchSource.SelectionFont, FontStyle.Underline)
rchSource.Select(txt.IndexOf("lazy"), Len("lazy"))
rchSource.SelectionFont = New Font(rchSource.SelectionFont, FontStyle.Bold)
rchSource.Select(txt.IndexOf("dog"), Len("dog"))
rchSource.SelectionFont = New Font(rchSource.SelectionFont, FontStyle.Bold)
rchSource.SelectionColor = Color.Blue
rchSource.Select(0, 0)
End Sub
Private Sub btnCopy_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnCopy.Click
Dim data_object As New DataObject
data_object.SetData(DataFormats.Rtf, rchSource.Rtf)
data_object.SetData(DataFormats.Text, rchSource.Text)
Dim html_text As String
html_text = "<HTML>" & vbCrLf
html_text &= " <HEAD>The Quick Brown Fox</HEAD>" & vbCrLf
html_text &= " <BODY>" & vbCrLf
html_text &= rchSource.Text & vbCrLf
html_text &= " </BODY>" & vbCrLf & "</HTML>"
data_object.SetData(DataFormats.Html, html_text)
Clipboard.SetDataObject(data_object)
End Sub
Private Sub btnPaste_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnPaste.Click
Dim data_object As IDataObject = Clipboard.GetDataObject()
If data_object.GetDataPresent(DataFormats.Rtf) Then
rchTarget.Rtf = data_object.GetData(DataFormats.Rtf).ToString
lblRtf.Text = data_object.GetData(DataFormats.Rtf).ToString
Else
rchTarget.Text = ""
lblRtf.Text = ""
End If
If data_object.GetDataPresent(DataFormats.Text) Then
lblTarget.Text = data_object.GetData(DataFormats.Text).ToString
Else
lblTarget.Text = ""
End If
If data_object.GetDataPresent(DataFormats.Html) Then
lblHtml.Text = data_object.GetData(DataFormats.Html).ToString
Else
lblHtml.Text = ""
End If
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Public Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.btnPaste = New System.Windows.Forms.Button
Me.btnCopy = New System.Windows.Forms.Button
Me.lblRtf = New System.Windows.Forms.Label
Me.lblHtml = New System.Windows.Forms.Label
Me.lblTarget = New System.Windows.Forms.Label
Me.rchTarget = New System.Windows.Forms.RichTextBox
Me.rchSource = New System.Windows.Forms.RichTextBox
Me.SuspendLayout()
'
'btnPaste
'
Me.btnPaste.Anchor = CType((System.Windows.Forms.AnchorStyles.Top Or System.Windows.Forms.AnchorStyles.Right), System.Windows.Forms.AnchorStyles)
Me.btnPaste.Location = New System.Drawing.Point(240, 40)
Me.btnPaste.Name = "btnPaste"
Me.btnPaste.Size = New System.Drawing.Size(48, 24)
Me.btnPaste.TabIndex = 16
Me.btnPaste.Text = "Paste"
'
'btnCopy
'
Me.btnCopy.Location = New System.Drawing.Point(8, 40)
Me.btnCopy.Name = "btnCopy"
Me.btnCopy.Size = New System.Drawing.Size(48, 24)
Me.btnCopy.TabIndex = 15
Me.btnCopy.Text = "Copy"
'
'lblRtf
'
Me.lblRtf.Anchor = CType(((System.Windows.Forms.AnchorStyles.Top Or System.Windows.Forms.AnchorStyles.Left) _
Or System.Windows.Forms.AnchorStyles.Right), System.Windows.Forms.AnchorStyles)
Me.lblRtf.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D
Me.lblRtf.Location = New System.Drawing.Point(0, 104)
Me.lblRtf.Margin = New System.Windows.Forms.Padding(3, 3, 3, 1)
Me.lblRtf.Name = "lblRtf"
Me.lblRtf.Size = New System.Drawing.Size(296, 110)
Me.lblRtf.TabIndex = 14
'
'lblHtml
'
Me.lblHtml.Anchor = CType(((System.Windows.Forms.AnchorStyles.Top Or System.Windows.Forms.AnchorStyles.Left) _
Or System.Windows.Forms.AnchorStyles.Right), System.Windows.Forms.AnchorStyles)
Me.lblHtml.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D
Me.lblHtml.Location = New System.Drawing.Point(0, 248)
Me.lblHtml.Margin = New System.Windows.Forms.Padding(3, 2, 3, 3)
Me.lblHtml.Name = "lblHtml"
Me.lblHtml.Size = New System.Drawing.Size(296, 80)
Me.lblHtml.TabIndex = 13
'
'lblTarget
'
Me.lblTarget.Anchor = CType(((System.Windows.Forms.AnchorStyles.Top Or System.Windows.Forms.AnchorStyles.Left) _
Or System.Windows.Forms.AnchorStyles.Right), System.Windows.Forms.AnchorStyles)
Me.lblTarget.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D
Me.lblTarget.Location = New System.Drawing.Point(0, 216)
Me.lblTarget.Margin = New System.Windows.Forms.Padding(3, 1, 3, 2)
Me.lblTarget.Name = "lblTarget"
Me.lblTarget.Size = New System.Drawing.Size(296, 28)
Me.lblTarget.TabIndex = 12
'
'rchTarget
'
Me.rchTarget.AllowDrop = True
Me.rchTarget.Anchor = CType(((System.Windows.Forms.AnchorStyles.Top Or System.Windows.Forms.AnchorStyles.Left) _
Or System.Windows.Forms.AnchorStyles.Right), System.Windows.Forms.AnchorStyles)
Me.rchTarget.Location = New System.Drawing.Point(0, 72)
Me.rchTarget.Name = "rchTarget"
Me.rchTarget.Size = New System.Drawing.Size(296, 28)
Me.rchTarget.TabIndex = 11
Me.rchTarget.Text = ""
'
'rchSource
'
Me.rchSource.AllowDrop = True
Me.rchSource.Anchor = CType(((System.Windows.Forms.AnchorStyles.Top Or System.Windows.Forms.AnchorStyles.Left) _
Or System.Windows.Forms.AnchorStyles.Right), System.Windows.Forms.AnchorStyles)
Me.rchSource.Location = New System.Drawing.Point(0, 0)
Me.rchSource.Name = "rchSource"
Me.rchSource.Size = New System.Drawing.Size(296, 28)
Me.rchSource.TabIndex = 10
Me.rchSource.Text = ""
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 13.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(296, 329)
Me.Controls.Add(Me.btnPaste)
Me.Controls.Add(Me.btnCopy)
Me.Controls.Add(Me.lblRtf)
Me.Controls.Add(Me.lblHtml)
Me.Controls.Add(Me.lblTarget)
Me.Controls.Add(Me.rchTarget)
Me.Controls.Add(Me.rchSource)
Me.Name = "Form1"
Me.Text = "CopyPasteRichText"
Me.ResumeLayout(False)
End Sub
Friend WithEvents btnPaste As System.Windows.Forms.Button
Friend WithEvents btnCopy As System.Windows.Forms.Button
Friend WithEvents lblRtf As System.Windows.Forms.Label
Friend WithEvents lblHtml As System.Windows.Forms.Label
Friend WithEvents lblTarget As System.Windows.Forms.Label
Friend WithEvents rchTarget As System.Windows.Forms.RichTextBox
Friend WithEvents rchSource As System.Windows.Forms.RichTextBox
End Class