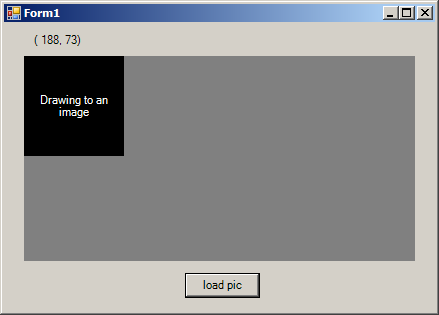
Imports System.Drawing
Imports System.Drawing.Drawing2D
Imports System.Windows.Forms
public class PictureBoxSingleDoubleClicked
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Private oldcolor As Color
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
PictureBox1.Image = System.Drawing.Image.FromFile("yourfile.jpg")
Label1.Text = "Loaded"
End Sub
Private Sub Button1_MouseDown(ByVal sender As System.Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles Button1.MouseDown
If (e.Button <> MouseButtons.Left) Then
Label1.Text = "Left button"
End If
End Sub
Private Sub PictureBox1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles PictureBox1.Click
Label1.Text = "single cliked"
End Sub
Private Sub PictureBox1_DoubleClick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles PictureBox1.DoubleClick
Label1.Text = "double clicked"
End Sub
Private Sub PictureBox1_MouseMove(ByVal sender As System.Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles PictureBox1.MouseMove
PictureBox1.BackColor = System.Drawing.Color.Gray
PictureBox1.Image = System.Drawing.Image.FromFile("yourfile.jpg")
Label1.Text = "(" + Str(e.X) + "," + Str(e.Y) + ")"
End Sub
Private Sub PictureBox1_MouseLeave(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles PictureBox1.MouseLeave
PictureBox1.BackColor = oldcolor
PictureBox1.Image = System.Drawing.Image.FromFile("yourfile.jpg")
Label1.Text = "Exit"
End Sub
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
oldcolor = PictureBox1.BackColor
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.Button1 = New System.Windows.Forms.Button
Me.Label1 = New System.Windows.Forms.Label
Me.PictureBox1 = New System.Windows.Forms.PictureBox
CType(Me.PictureBox1, System.ComponentModel.ISupportInitialize).BeginInit()
Me.SuspendLayout()
'
'Button1
'
Me.Button1.Location = New System.Drawing.Point(181, 231)
Me.Button1.Name = "Button1"
Me.Button1.Size = New System.Drawing.Size(75, 23)
Me.Button1.TabIndex = 0
Me.Button1.Text = "load pic"
Me.Button1.UseVisualStyleBackColor = True
'
'Label1
'
Me.Label1.AutoSize = True
Me.Label1.Location = New System.Drawing.Point(28, 9)
Me.Label1.Name = "Label1"
Me.Label1.Size = New System.Drawing.Size(41, 12)
Me.Label1.TabIndex = 1
Me.Label1.Text = "Label1"
'
'PictureBox1
'
Me.PictureBox1.Location = New System.Drawing.Point(20, 30)
Me.PictureBox1.Name = "PictureBox1"
Me.PictureBox1.Size = New System.Drawing.Size(391, 189)
Me.PictureBox1.TabIndex = 2
Me.PictureBox1.TabStop = False
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 12.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(431, 266)
Me.Controls.Add(Me.PictureBox1)
Me.Controls.Add(Me.Label1)
Me.Controls.Add(Me.Button1)
Me.Name = "Form1"
Me.Text = "Form1"
CType(Me.PictureBox1, System.ComponentModel.ISupportInitialize).EndInit()
Me.ResumeLayout(False)
Me.PerformLayout()
End Sub
Friend WithEvents Button1 As System.Windows.Forms.Button
Friend WithEvents Label1 As System.Windows.Forms.Label
Friend WithEvents PictureBox1 As System.Windows.Forms.PictureBox
End Class