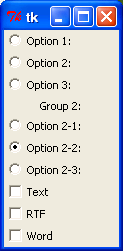
from Tkinter import *
class SelectionTest :
def __init__(self) :
self.root = Tk()
self.group_1 = IntVar()
Radiobutton(self.root, text="Option 1:", variable=self.group_1, value=1).pack(anchor=W)
Radiobutton(self.root, text="Option 2:", variable=self.group_1, value=2).pack(anchor=W)
Radiobutton(self.root, text="Option 3:", variable=self.group_1, value=3).pack(anchor=W)
Label(self.root, text="Group 2:").pack()
self.group_2 = IntVar()
Radiobutton(self.root, text="Option 2-1:", variable=self.group_2, value=1).pack(anchor=W)
Radiobutton(self.root, text="Option 2-2:", variable=self.group_2, value=2).pack(anchor=W)
Radiobutton(self.root, text="Option 2-3:", variable=self.group_2, value=3).pack(anchor=W)
self.check_1 = IntVar()
self.check_2 = IntVar()
self.check_3 = IntVar()
Checkbutton(self.root, text="Text", variable=self.check_1).pack(anchor=W)
Checkbutton(self.root, text="RTF", variable=self.check_2).pack(anchor=W)
Checkbutton(self.root, text="Word", variable=self.check_3).pack(anchor=W)
self.root.mainloop()
st = SelectionTest()
print "Group 1 selection: "+str(st.group_1.get())
print "Group 2 selection: "+str(st.group_2.get())
print "Check Box 1: " + str(st.check_1.get())
print "Check Box 2: " + str(st.check_2.get())
print "Check Box 3: " + str(st.check_3.get())