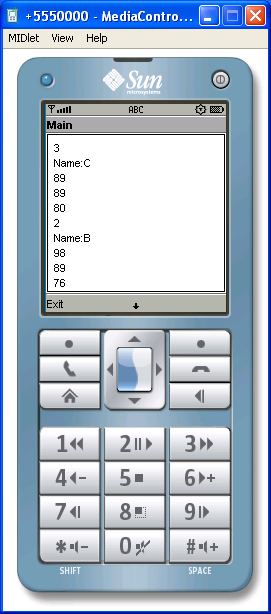
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.TextBox;
import javax.microedition.lcdui.TextField;
import javax.microedition.midlet.MIDlet;
import javax.microedition.rms.RecordEnumeration;
import javax.microedition.rms.RecordFilter;
import javax.microedition.rms.RecordStore;
import javax.microedition.rms.RecordStoreNotFoundException;
public class FilterFieldsMIDlet extends MIDlet implements CommandListener {
private Command exitCommand;
private Display display;
private String[] names = { "A", "B", "C", "D" };
private int[] chineseScore = { 74, 98, 89, 76 };
private int[] englishScore = { 67, 89, 89, 78 };
private int[] mathScore = { 80, 76, 80, 78 };
public FilterFieldsMIDlet() {
display = Display.getDisplay(this);
exitCommand = new Command("Exit", Command.EXIT, 1);
}
public void startApp() {
TextBox aTextBox = new TextBox("Main", null, 256, TextField.ANY);
RecordStore rs = null;
byte[] nameEmail = null;
boolean existingOrNot = false;
boolean OK = true;
existingOrNot = existing("aRS3");
if (existingOrNot) {
try {
rs = RecordStore.openRecordStore("aRS3", false);
} catch (Exception e) {
OK = false;
} finally {
if (OK) {
aTextBox.setString("Ok");
} else {
aTextBox.setString("not tOk");
}
}
} else {
try {
rs = RecordStore.openRecordStore("aRS3", true);
} catch (Exception e) {
OK = false;
} finally {
if (OK) {
aTextBox.setString("Ok");
} else {
aTextBox.setString("Not Ok");
}
}
}
Student aStudent = null;
if (OK)
try {
for (int i = 0; i < names.length; i++) {
aStudent = new Student();
aStudent.write(names[i], chineseScore[i], englishScore[i], mathScore[i]);
byte[] data = aStudent.changeToByteArray();
int recordID = aStudent.getRecordID();
if (recordID != -1) {
rs.setRecord(recordID, data, 0, data.length);
} else {
recordID = rs.addRecord(data, 0, data.length);
aStudent.setRecordID(recordID);
}
aStudent = null;
}
aTextBox.setString("Added");
} catch (Exception e) {
aTextBox.setString("Failed");
}
String result = "";
aStudent = new Student();
if (OK)
try {
byte[] data;
int number = 0;
RecordFilter rf = new averageFilter();
RecordEnumeration re = rs.enumerateRecords(rf, null, false);
while (re.hasNextElement()) {
int recordID = re.nextRecordId();
data = rs.getRecord(recordID);
aStudent.changeFromByteArray(data);
result += recordID + "\n" + "Name:" + aStudent.getName() + "\n"
+ aStudent.getChineseScore() + "\n" + aStudent.getEnglishScore() + "\n"
+ aStudent.getMathScore() + "\n";
number++;
}
result += number;
aTextBox.setString(result);
} catch (Exception e) {
aTextBox.setString("Failed");
try {
rs.closeRecordStore();
System.out.println("1.Closed.");
RecordStore.deleteRecordStore("aRS3");
System.out.println("delete OK");
} catch (Exception x) {
}
} finally {
try {
if (rs != null)
rs.closeRecordStore();
rs.deleteRecordStore("aRS3");
} catch (Exception e) {
}
}
aTextBox.setString(result);
aTextBox.addCommand(exitCommand);
aTextBox.setCommandListener(this);
display.setCurrent(aTextBox);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
public boolean existing(String recordStoreName) {
boolean existingOrNot = false;
RecordStore rs = null;
if (recordStoreName.length() > 32)
return false;
try {
rs = RecordStore.openRecordStore(recordStoreName, false);
} catch (RecordStoreNotFoundException e) {
existingOrNot = false;
} catch (Exception e) {
} finally {
try {
rs.closeRecordStore();
} catch (Exception e) {
}
}
return existingOrNot;
}
public void commandAction(Command c, Displayable s) {
destroyApp(false);
notifyDestroyed();
}
}
class averageFilter implements RecordFilter {
public boolean matches(byte[] candidate) {
DataInputStream student = new DataInputStream(new ByteArrayInputStream(candidate));
int average = 0;
try {
String dummy = student.readUTF();
average = (student.readInt() + student.readInt() + student.readInt()) / 3;
} catch (Exception e) {
}
if (average >= 80)
return true;
else
return false;
}
}
class Student {
private int ID = -1;
private String name;
private int chineseScore;
private int englishScore;
private int mathScore;
public void write(String name, int chineseScore, int englishScore, int mathScore) {
this.name = name;
this.chineseScore = chineseScore;
this.englishScore = englishScore;
this.mathScore = mathScore;
}
public void setRecordID(int ID) {
this.ID = ID;
}
public int getRecordID() {
return ID;
}
public byte[] changeToByteArray() {
byte[] data = null;
try {
ByteArrayOutputStream baos = new ByteArrayOutputStream();
DataOutputStream dos = new DataOutputStream(baos);
dos.writeUTF(name);
dos.writeInt(chineseScore);
dos.writeInt(englishScore);
dos.writeInt(mathScore);
data = baos.toByteArray();
baos.close();
dos.close();
} catch (Exception e) {
}
return data;
}
public void changeFromByteArray(byte[] data) {
try {
ByteArrayInputStream bais = new ByteArrayInputStream(data);
DataInputStream dis = new DataInputStream(bais);
name = dis.readUTF();
chineseScore = dis.readInt();
englishScore = dis.readInt();
mathScore = dis.readInt();
bais.close();
dis.close();
} catch (Exception e) {
}
}
public String getName() {
return name;
}
public int getChineseScore() {
return chineseScore;
}
public int getEnglishScore() {
return englishScore;
}
public int getMathScore() {
return mathScore;
}
}