Specifies whether controls will be wrapped to the next row or column if there is not enough space left on the current row or column.
The default value is true.
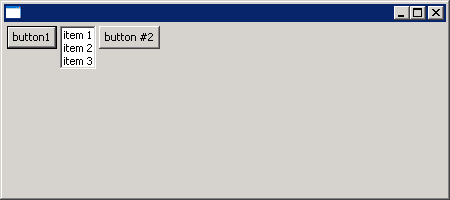
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.List;
import org.eclipse.swt.widgets.Shell;
public class RowLayoutWrap {
public static void main(String[] args) {
Display display = new Display();
final Shell shell = new Shell(display);
RowLayout rowLayout = new RowLayout();
rowLayout.wrap = false;
shell.setLayout(rowLayout);
Button button1 = new Button(shell, SWT.PUSH);
button1.setText("button1");
List list = new List(shell, SWT.BORDER);
list.add("item 1");
list.add("item 2");
list.add("item 3");
Button button2 = new Button(shell, SWT.PUSH);
button2.setText("button #2");
shell.setSize(450, 200);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
}