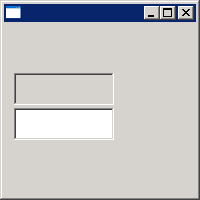
import org.eclipse.swt.SWT;
import org.eclipse.swt.widgets.Canvas;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Event;
import org.eclipse.swt.widgets.Listener;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;
public class CanvasTranverseEvent {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
final Canvas c = new Canvas(shell, SWT.BORDER);
c.setBounds(10, 50, 100, 32);
c.addListener(SWT.Traverse, new Listener() {
public void handleEvent(Event e) {
switch (e.detail) {
/* Do tab group traversal */
case SWT.TRAVERSE_ESCAPE:
System.out.println("SWT.TRAVERSE_ESCAPE");
break;
case SWT.TRAVERSE_RETURN:
case SWT.TRAVERSE_TAB_NEXT:
System.out.println("SWT.TRAVERSE_TAB_NEXT");
break;
case SWT.TRAVERSE_TAB_PREVIOUS:
case SWT.TRAVERSE_PAGE_NEXT:
case SWT.TRAVERSE_PAGE_PREVIOUS:
e.doit = true;
break;
}
}
});
Text t = new Text(shell, SWT.SINGLE | SWT.BORDER);
t.setBounds(10, 85, 100, 32);
c.setFocus();
shell.setSize(200, 200);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}