Modes | Description |
AUTO_RESIZE_ALL_COLUMNS | Adjusts all column widths proportionally. |
AUTO_RESIZE_LAST_COLUMN | Adjusts the rightmost column width only to give or take space as required by the column currently being altered. |
AUTO_RESIZE_NEXT_COLUMN | If you're reducing the width of a neighboring column, the neighboring column will grow to fill the unused space. If you're increasing the width of a column, the neighboring column will shrink. |
AUTO_RESIZE_OFF | Turns off the user's ability to resize columns. The columns can still be resized programmatically. |
AUTO_RESIZE_SUBSEQUENT_COLUMNS | Adjusts the width by proportionally altering (default) columns displayed to the right of the column being changed. |
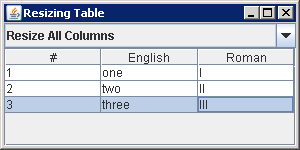
import java.awt.BorderLayout;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTable;
public class ResizeTable {
public static void main(String args[]) {
final Object rowData[][] = {
{ "1", "one", "I" },
{ "2", "two", "II" },
{ "3", "three", "III" }};
final String columnNames[] = { "#", "English", "Roman" };
final JTable table = new JTable(rowData, columnNames);
JScrollPane scrollPane = new JScrollPane(table);
String modes[] = { "Resize All Columns", "Resize Last Column", "Resize Next Column",
"Resize Off", "Resize Subsequent Columns" };
final int modeKey[] = { JTable.AUTO_RESIZE_ALL_COLUMNS, JTable.AUTO_RESIZE_LAST_COLUMN,
JTable.AUTO_RESIZE_NEXT_COLUMN, JTable.AUTO_RESIZE_OFF,
JTable.AUTO_RESIZE_SUBSEQUENT_COLUMNS };
JComboBox resizeModeComboBox = new JComboBox(modes);
ItemListener itemListener = new ItemListener() {
public void itemStateChanged(ItemEvent e) {
JComboBox source = (JComboBox) e.getSource();
int index = source.getSelectedIndex();
table.setAutoResizeMode(modeKey[index]);
}
};
resizeModeComboBox.addItemListener(itemListener);
JFrame frame = new JFrame("Resizing Table");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(resizeModeComboBox, BorderLayout.NORTH);
frame.add(scrollPane, BorderLayout.CENTER);
frame.setSize(300, 150);
frame.setVisible(true);
}
}