- The empty border leaves a transparent margin without any associated drawing.
- It's an alternative to using the insets in AWT.
- The class requires that fields such as top, left, bottom, and right be specified.
- You can also use an insets object to create an empty border.
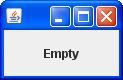
import javax.swing.BorderFactory;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
public class EmptyBorderForLabel extends JFrame {
public EmptyBorderForLabel() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel();
JLabel label;
label = new JLabel("Empty");
label.setBorder(BorderFactory.createEmptyBorder(10, 20, 10, 20));
panel.add(label);
getContentPane().add(panel);
pack();
}
public static void main(String[] args) {
EmptyBorderForLabel s = new EmptyBorderForLabel();
s.setVisible(true);
}
}