
/*
*
* Copyright (c) 1998 Sun Microsystems, Inc. All Rights Reserved.
*
* Sun grants you ("Licensee") a non-exclusive, royalty free, license to use,
* modify and redistribute this software in source and binary code form,
* provided that i) this copyright notice and license appear on all copies of
* the software; and ii) Licensee does not utilize the software in a manner
* which is disparaging to Sun.
*
* This software is provided "AS IS," without a warranty of any kind. ALL
* EXPRESS OR IMPLIED CONDITIONS, REPRESENTATIONS AND WARRANTIES, INCLUDING ANY
* IMPLIED WARRANTY OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE OR
* NON-INFRINGEMENT, ARE HEREBY EXCLUDED. SUN AND ITS LICENSORS SHALL NOT BE
* LIABLE FOR ANY DAMAGES SUFFERED BY LICENSEE AS A RESULT OF USING, MODIFYING
* OR DISTRIBUTING THE SOFTWARE OR ITS DERIVATIVES. IN NO EVENT WILL SUN OR ITS
* LICENSORS BE LIABLE FOR ANY LOST REVENUE, PROFIT OR DATA, OR FOR DIRECT,
* INDIRECT, SPECIAL, CONSEQUENTIAL, INCIDENTAL OR PUNITIVE DAMAGES, HOWEVER
* CAUSED AND REGARDLESS OF THE THEORY OF LIABILITY, ARISING OUT OF THE USE OF
* OR INABILITY TO USE SOFTWARE, EVEN IF SUN HAS BEEN ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGES.
*
* This software is not designed or intended for use in on-line control of
* aircraft, air traffic, aircraft navigation or aircraft communications; or in
* the design, construction, operation or maintenance of any nuclear
* facility. Licensee represents and warrants that it will not use or
* redistribute the Software for such purposes.
*/
import java.awt.Component;
import java.awt.Dimension;
import java.awt.GridLayout;
import javax.swing.DefaultCellEditor;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.table.AbstractTableModel;
import javax.swing.table.DefaultTableCellRenderer;
import javax.swing.table.TableCellRenderer;
import javax.swing.table.TableColumn;
public class TableRenderDemo extends JPanel {
private boolean DEBUG = false;
public TableRenderDemo() {
super(new GridLayout(1, 0));
JTable table = new JTable(new MyTableModel());
table.setPreferredScrollableViewportSize(new Dimension(500, 70));
// Create the scroll pane and add the table to it.
JScrollPane scrollPane = new JScrollPane(table);
// Set up column sizes.
initColumnSizes(table);
// Fiddle with the Sport column's cell editors/renderers.
setUpSportColumn(table, table.getColumnModel().getColumn(2));
// Add the scroll pane to this panel.
add(scrollPane);
}
/*
* This method picks good column sizes. If all column heads are wider than the
* column's cells' contents, then you can just use column.sizeWidthToFit().
*/
private void initColumnSizes(JTable table) {
MyTableModel model = (MyTableModel) table.getModel();
TableColumn column = null;
Component comp = null;
int headerWidth = 0;
int cellWidth = 0;
Object[] longValues = model.longValues;
TableCellRenderer headerRenderer = table.getTableHeader().getDefaultRenderer();
for (int i = 0; i < 5; i++) {
column = table.getColumnModel().getColumn(i);
comp = headerRenderer.getTableCellRendererComponent(null, column.getHeaderValue(), false,
false, 0, 0);
headerWidth = comp.getPreferredSize().width;
comp = table.getDefaultRenderer(model.getColumnClass(i)).getTableCellRendererComponent(table,
longValues[i], false, false, 0, i);
cellWidth = comp.getPreferredSize().width;
if (DEBUG) {
System.out.println("Initializing width of column " + i + ". " + "headerWidth = "
+ headerWidth + "; cellWidth = " + cellWidth);
}
// XXX: Before Swing 1.1 Beta 2, use setMinWidth instead.
column.setPreferredWidth(Math.max(headerWidth, cellWidth));
}
}
public void setUpSportColumn(JTable table, TableColumn sportColumn) {
// Set up the editor for the sport cells.
JComboBox comboBox = new JComboBox();
comboBox.addItem("Snowboarding");
comboBox.addItem("Rowing");
comboBox.addItem("Knitting");
comboBox.addItem("Speed reading");
comboBox.addItem("Pool");
comboBox.addItem("None of the above");
sportColumn.setCellEditor(new DefaultCellEditor(comboBox));
// Set up tool tips for the sport cells.
DefaultTableCellRenderer renderer = new DefaultTableCellRenderer();
renderer.setToolTipText("Click for combo box");
sportColumn.setCellRenderer(renderer);
}
class MyTableModel extends AbstractTableModel {
private String[] columnNames = { "First Name", "Last Name", "Sport", "# of Years", "Vegetarian" };
private Object[][] data = {
{ "Mary", "Campione", "Snowboarding", new Integer(5), new Boolean(false) },
{ "Alison", "Huml", "Rowing", new Integer(3), new Boolean(true) },
{ "Kathy", "Walrath", "Knitting", new Integer(2), new Boolean(false) },
{ "Sharon", "Zakhour", "Speed reading", new Integer(20), new Boolean(true) },
{ "Philip", "Milne", "Pool", new Integer(10), new Boolean(false) } };
public final Object[] longValues = { "Sharon", "Campione", "None of the above",
new Integer(20), Boolean.TRUE };
public int getColumnCount() {
return columnNames.length;
}
public int getRowCount() {
return data.length;
}
public String getColumnName(int col) {
return columnNames[col];
}
public Object getValueAt(int row, int col) {
return data[row][col];
}
/*
* JTable uses this method to determine the default renderer/ editor for
* each cell. If we didn't implement this method, then the last column would
* contain text ("true"/"false"), rather than a check box.
*/
public Class getColumnClass(int c) {
return getValueAt(0, c).getClass();
}
/*
* Don't need to implement this method unless your table's editable.
*/
public boolean isCellEditable(int row, int col) {
// Note that the data/cell address is constant,
// no matter where the cell appears onscreen.
if (col < 2) {
return false;
} else {
return true;
}
}
/*
* Don't need to implement this method unless your table's data can change.
*/
public void setValueAt(Object value, int row, int col) {
if (DEBUG) {
System.out.println("Setting value at " + row + "," + col + " to " + value
+ " (an instance of " + value.getClass() + ")");
}
data[row][col] = value;
fireTableCellUpdated(row, col);
if (DEBUG) {
System.out.println("New value of data:");
printDebugData();
}
}
private void printDebugData() {
int numRows = getRowCount();
int numCols = getColumnCount();
for (int i = 0; i < numRows; i++) {
System.out.print(" row " + i + ":");
for (int j = 0; j < numCols; j++) {
System.out.print(" " + data[i][j]);
}
System.out.println();
}
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("TableRenderDemo");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Create and set up the content pane.
TableRenderDemo newContentPane = new TableRenderDemo();
newContentPane.setOpaque(true); // content panes must be opaque
frame.setContentPane(newContentPane);
// Display the window.
frame.pack();
frame.setVisible(true);
}
}
14.51.JTabbedPane |
| 14.51.1. | First usage of JTabbedPane |
| 14.51.2. | Adding and Removing Tabs | 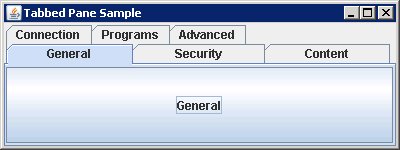 |
| 14.51.3. | Get the index of the first tab that matches an icon |
| 14.51.4. | Changing tab's title, icon, mnemonic, tooltip, or component on a particular tab with one of the setXXXAt() methods | 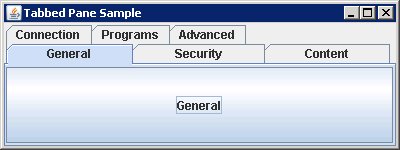 |
| 14.51.5. | Adds tool tips to a table using a renderer |  |
| 14.51.6. | Specifying a tab's location: TOP, BOTTOM, LEFT, or RIGHT | 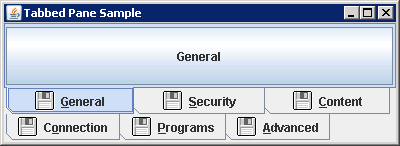 |
| 14.51.7. | Changing background, foreground and icon | 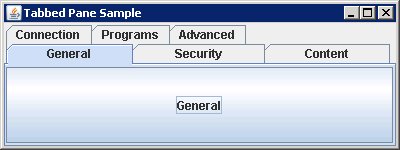 |
| 14.51.8. | To remove a tab, you can remove a specific tab with removeTabAt(int index), remove(int index), or remove(Component component) | 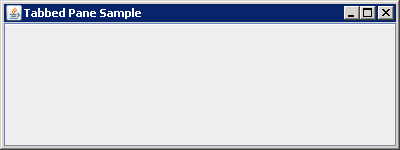 |
| 14.51.9. | Listening for Selected Tab Changes | 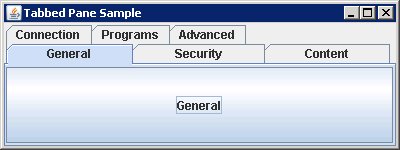 |
| 14.51.10. | TabLayout Policy: SCROLL_TAB_LAYOUT or WRAP_TAP_LAYOUT | 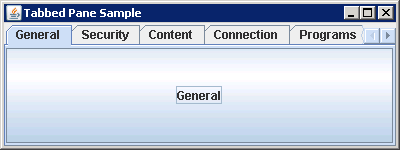 |
| 14.51.11. | Add Components to JTabbedPane |
| 14.51.12. | New Methods in the JTabPane Component (Add component to JTabPane) |
| 14.51.13. | JTabPane with TextField in the tab | 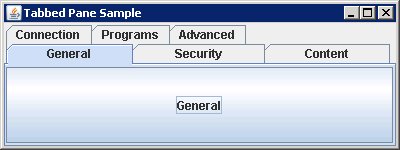 |
| 14.51.14. | Add user icon to tab panel | 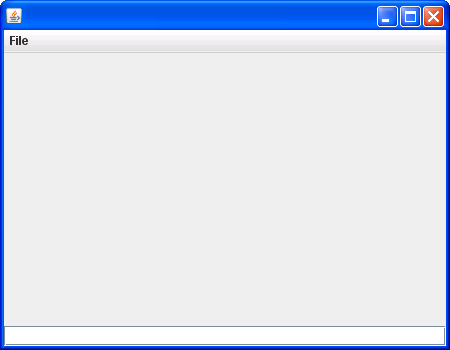 |
| 14.51.15. | Add Button to tab bar | 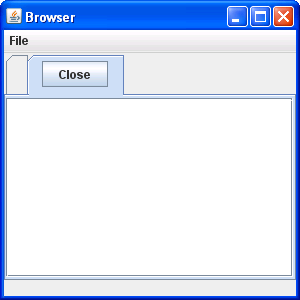 |
| 14.51.16. | Add a tab with a label taken from the name of the component |
| 14.51.17. | Add a tab with a label at the end of all tabs |
| 14.51.18. | Add a tab with a label and icon at the end of all tabs |
| 14.51.19. | Add a tab with a label, icon, and tool tip at the end of all tabs |
| 14.51.20. | Getting the Tabs in a JTabbedPane Container |
| 14.51.21. | Enable Scrolling Tabs in a JTabbedPane Container |
| 14.51.22. | Enabling the Selection of a Tab in a JTabbedPane Container Using a Keystroke |
| 14.51.23. | Enabling and Disabling a Tab in a JTabbedPane Container |
| 14.51.24. | Setting the Color of a Tab in a JTabbedPane Container |
| 14.51.25. | Setting the Tool Tip for a Tab in a JTabbedPane Container |
| 14.51.26. | Setting the Location of the Tabs in a JTabbedPane Container |
| 14.51.27. | Determining When the Selected Tab Changes in a JTabbedPane Container |
| 14.51.28. | Moving a Tab in a JTabbedPane Container |
| 14.51.29. | Insert a tab after the first tab |
| 14.51.30. | Get the index of the tab by matching the child component |
| 14.51.31. | Getting and Setting the Selected Tab in a JTabbedPane Container |
| 14.51.32. | Setting the Size of the Divider in a JSplitPane Container |
| 14.51.33. | Customizing a JTabbedPane Look and Feel |