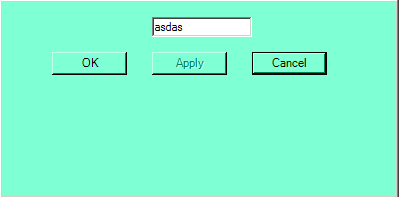
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class DialogApplyEvent : System.Windows.Forms.Form
{
private System.Windows.Forms.Button btnCreate;
private System.Windows.Forms.Label lblReturn;
public DialogApplyEvent()
{
InitializeComponent();
}
private void InitializeComponent()
{
this.btnCreate = new System.Windows.Forms.Button();
this.lblReturn = new System.Windows.Forms.Label();
this.SuspendLayout();
//
// btnCreate
//
this.btnCreate.Location = new System.Drawing.Point(72, 176);
this.btnCreate.Name = "btnCreate";
this.btnCreate.Size = new System.Drawing.Size(144, 23);
this.btnCreate.TabIndex = 0;
this.btnCreate.Text = "Create Dialog Box";
this.btnCreate.Click += new System.EventHandler(this.btnCreate_Click);
//
// lblReturn
//
this.lblReturn.Location = new System.Drawing.Point(88, 88);
this.lblReturn.Name = "lblReturn";
this.lblReturn.TabIndex = 1;
//
// DialogApplyEvent
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 273);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.lblReturn,
this.btnCreate});
this.ResumeLayout(false);
}
[STAThread]
static void Main()
{
Application.Run(new DialogApplyEvent());
}
private void btnCreate_Click(object sender, System.EventArgs e)
{
DialogDemo dlg = new DialogDemo();
dlg.EnableApplyButton = false;
dlg.ClickApply += new EventHandler(DialogDemoOnApply);
dlg.ShowDialog();
if (dlg.DialogResult == DialogResult.OK)
{lblReturn.Text = dlg.TextOut;}
else
{lblReturn.Text = dlg.DialogResult.ToString();}
}
private void DialogDemoOnApply(object sender, System.EventArgs e)
{
DialogDemo dlg = (DialogDemo)sender;
lblReturn.Text = dlg.TextOut;
dlg.EnableApplyButton = false;
}
}
class DialogDemo : Form
{
private Button btnApply;
private TextBox txt;
public event EventHandler ClickApply;
public DialogDemo()
{
FormBorderStyle = FormBorderStyle.FixedDialog;
BackColor = System.Drawing.Color.Aquamarine;
ControlBox = false;
MaximizeBox = false;
MinimizeBox = false;
ShowInTaskbar = false;
Size = new Size(400,200);
StartPosition = FormStartPosition.CenterScreen;
Button btnOK = new Button();
btnOK.Text = "OK";
btnOK.DialogResult = DialogResult.OK;
btnOK.Location = new Point(50,50);
btnOK.TabIndex = 0;
btnOK.Click += new EventHandler(ApplyButtonOnClick);
Controls.Add(btnOK);
btnApply = new Button();
btnApply.Text = "Apply";
btnApply.Location = new Point(150,50);
btnApply.TabIndex = 1;
btnApply.Enabled = false;
btnApply.Click += new EventHandler(ApplyButtonOnClick);
Controls.Add(btnApply);
Button btnCancel = new Button();
btnCancel.Text = "Cancel";
btnCancel.DialogResult = DialogResult.Cancel;
btnCancel.Location = new Point(250,50);
btnCancel.TabIndex = 2;
Controls.Add(btnCancel);
txt = new TextBox();
txt.Size = new Size(100,15);
txt.Location = new Point(150,15);
txt.TextChanged += new EventHandler(TextBoxChanged);
Controls.Add(txt);
}
private void TextBoxChanged(object sender, EventArgs e)
{
this.EnableApplyButton = true;
}
public bool EnableApplyButton
{
get {return btnApply.Enabled; }
set {btnApply.Enabled = value; }
}
public string TextOut
{
get {return txt.Text; }
}
private void ApplyButtonOnClick (object sender, System.EventArgs e)
{
if (ClickApply != null)
ClickApply(this, new EventArgs());
}
}