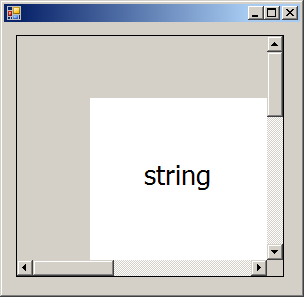
using System;
using System.Drawing;
using System.Windows.Forms;
public class BitmapCreateDrawing : Form
{
public BitmapCreateDrawing()
{
this.panel1 = new System.Windows.Forms.Panel();
this.pictureBox1 = new System.Windows.Forms.PictureBox();
this.panel1.SuspendLayout();
((System.ComponentModel.ISupportInitialize)(this.pictureBox1)).BeginInit();
this.SuspendLayout();
//
// panel1
//
this.panel1.AutoScroll = true;
this.panel1.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle;
this.panel1.Controls.Add(this.pictureBox1);
this.panel1.Location = new System.Drawing.Point(12, 12);
this.panel1.Size = new System.Drawing.Size(268, 242);
this.pictureBox1.Location = new System.Drawing.Point(73, 62);
this.pictureBox1.Size = new System.Drawing.Size(111, 144);
this.pictureBox1.TabStop = false;
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(296, 270);
this.Controls.Add(this.panel1);
this.panel1.ResumeLayout(false);
((System.ComponentModel.ISupportInitialize)(this.pictureBox1)).EndInit();
this.ResumeLayout(false);
string text = "string";
Font font = new Font("Tahoma", 20);
Bitmap b = new Bitmap(600, 600);
using (Graphics g = Graphics.FromImage(b))
{
g.FillRectangle(Brushes.White, new Rectangle(0, 0,
b.Width, b.Height));
g.DrawString(text, font, Brushes.Black, 50, 60);
}
pictureBox1.BackgroundImage = b;
pictureBox1.Size = b.Size;
}
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new BitmapCreateDrawing());
}
private System.Windows.Forms.Panel panel1;
private System.Windows.Forms.PictureBox pictureBox1;
}