Path Animation with DoubleAnimation Using Path, AutoReverse
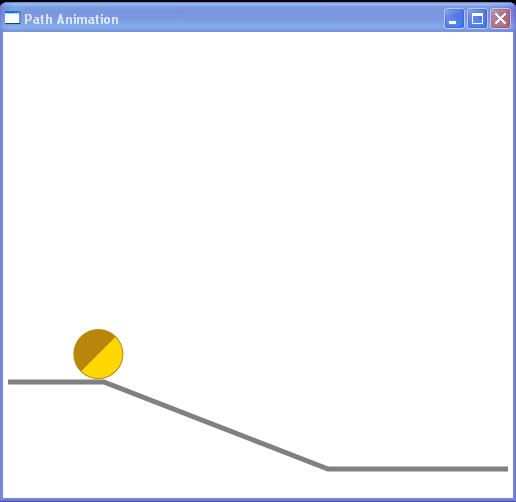
<Window x:Class="WpfApplication1.PathAnimationExample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Path Animation" Height="500" Width="518">
<Canvas Margin="5">
<Polyline Points="0,345,96,345,320,432,500,432" Stroke="Gray"
StrokeThickness="5" />
<Path>
<Path.Data>
<PathGeometry x:Name="path2" Figures="M0,292 L75,292 300,380,449,380" />
</Path.Data>
</Path>
<Ellipse Name="circle2" Stroke="DarkGoldenrod" Canvas.Left="0"
Canvas.Top="293" Width="50" Height="50">
<Ellipse.Fill>
<LinearGradientBrush>
<GradientStop Color="DarkGoldenrod" Offset="0.5" />
<GradientStop Color="Gold" Offset="0.5" />
</LinearGradientBrush>
</Ellipse.Fill>
<Ellipse.RenderTransform>
<RotateTransform x:Name="circle2Rotate" CenterX="25" CenterY="25" />
</Ellipse.RenderTransform>
</Ellipse>
</Canvas>
</Window>
//File:Window.xaml.vb
Imports System
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Input
Imports System.Windows.Media
Imports System.Windows.Media.Animation
Imports System.Windows.Shapes
Namespace WpfApplication1
Public Partial Class PathAnimationExample
Inherits Window
Public Sub New()
InitializeComponent()
path2.Freeze()
' For performance benefits.
Dim daPath As New DoubleAnimationUsingPath()
daPath.Duration = TimeSpan.FromSeconds(5)
daPath.RepeatBehavior = RepeatBehavior.Forever
daPath.AccelerationRatio = 0.6
daPath.DecelerationRatio = 0.4
daPath.AutoReverse = True
daPath.PathGeometry = path2
daPath.Source = PathAnimationSource.X
circle2.BeginAnimation(Canvas.LeftProperty, daPath)
daPath = New DoubleAnimationUsingPath()
daPath.Duration = TimeSpan.FromSeconds(5)
daPath.RepeatBehavior = RepeatBehavior.Forever
daPath.AccelerationRatio = 0.6
daPath.DecelerationRatio = 0.4
daPath.AutoReverse = True
daPath.PathGeometry = path2
daPath.Source = PathAnimationSource.Y
circle2.BeginAnimation(Canvas.TopProperty, daPath)
End Sub
End Class
End Namespace
Related examples in the same category