Bind to an Existing Object Instance
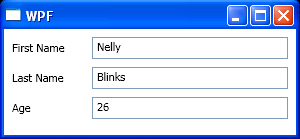
<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="WPF" Height="140" Width="300">
<Grid Margin="4">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="80"/>
<ColumnDefinition Width="*"/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="30"/>
<RowDefinition Height="30"/>
<RowDefinition Height="30"/>
</Grid.RowDefinitions>
<TextBlock Margin="4" Text="First Name" VerticalAlignment="Center"/>
<TextBox Margin="4" Text="{Binding Path=FirstName}"
Grid.Column="1"/>
<TextBlock Margin="4" Text="Last Name" Grid.Row="1" VerticalAlignment="Center"/>
<TextBox Margin="4" Text="{Binding Path=LastName}"
Grid.Column="1"
Grid.Row="1"/>
<TextBlock Margin="4" Text="Age" Grid.Row="2" VerticalAlignment="Center"/>
<TextBox Margin="4" Text="{Binding Path=Age}"
Grid.Column="1"
Grid.Row="2"/>
</Grid>
</Window>
//File:Window.xaml.vb
Imports System.Windows
Namespace WpfApplication1
Public Partial Class Window1
Inherits Window
Public Sub New()
InitializeComponent()
Me.DataContext = New Employee() With { _
.FirstName = "Nelly", _
.LastName = "Blinks", _
.Age = 26 _
}
End Sub
End Class
Public Class Employee
Public Property FirstName() As String
Get
Return m_FirstName
End Get
Set
m_FirstName = Value
End Set
End Property
Private m_FirstName As String
Public Property LastName() As String
Get
Return m_LastName
End Get
Set
m_LastName = Value
End Set
End Property
Private m_LastName As String
Public Property Age() As Integer
Get
Return m_Age
End Get
Set
m_Age = Value
End Set
End Property
Private m_Age As Integer
End Class
End Namespace
Related examples in the same category