Put time-consuming Task in Thread
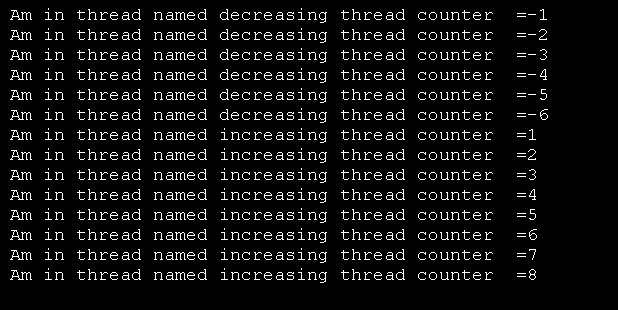
Imports System
Imports System.Threading
Public Class MainClass
Public Shared Sub Main()
Dim aThreadStart As New Threading.ThreadStart(AddressOf Adding)
Dim aThread As New Thread(aThreadStart)
aThread.Name = "increasing thread"
Dim bThreadStart As New Threading.ThreadStart(AddressOf Substracting)
Dim bThread As New Thread(AddressOf Substracting)
bThread.Name = "decreasing thread"
aThread.Start()
bThread.Start()
End Sub
Shared Public Sub Adding()
Dim count As Integer
Do While True
count += 1
Console.WriteLine("Am in thread named " & Thread.CurrentThread.Name & " counter =" & count)
If count Mod 100 = 0 Then
Try
Thread.Sleep(1000)
Catch e As ThreadInterruptedException
End Try
End If
Loop
End Sub
Shared Public Sub Substracting()
Dim count As Integer
Do
count -= 1
Console.WriteLine("Am in thread named " & Thread.CurrentThread.Name & " counter =" & count)
If count Mod 200 = 0 Then
Try
Thread.Sleep(1000)
Catch e As ThreadInterruptedException
'thread interrupted from sleeping
End Try
End If
Loop
End Sub
End Class
Related examples in the same category