Define and use a generic Tree data structure
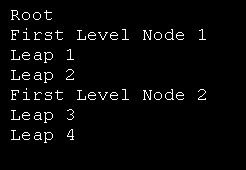
Imports System
Imports System.Windows.Forms
Imports System.Collections.Generic
Imports System.ComponentModel
Public Class MainClass
Shared Sub Main()
Dim family_tree As New Tree(Of Person)
Dim root As TreeNode(Of Person) = _
family_tree.MakeRoot(New Person("Root"))
Dim child1 As TreeNode(Of Person) = _
root.AddChild(New Person("First Level Node 1"))
Dim child2 As TreeNode(Of Person) = _
root.AddChild(New Person("First Level Node 2"))
child1.AddChild(New Person("Leap 1"))
child1.AddChild(New Person("Leap 2"))
child2.AddChild(New Person("Leap 3"))
child2.AddChild(New Person("Leap 4"))
' Display the result.
Console.WriteLine(family_tree.ToString())
End Sub
End Class
Public Class Person
Public Name As String
Public Sub New(ByVal new_name As String)
Name = new_name
End Sub
Public Overrides Function ToString() As String
Return Name
End Function
End Class
Public Class Tree(Of data_type)
Private m_Root As TreeNode(Of data_type) = Nothing
<Description("The tree's root node."), _
Category("Data")> _
Public Property Root() As TreeNode(Of data_type)
Get
Return m_Root
End Get
Set(ByVal value As TreeNode(Of data_type))
m_Root = value
End Set
End Property
Public Sub Clear()
m_Root = Nothing
End Sub
Public Function MakeRoot(ByVal node_item As data_type) As TreeNode(Of data_type)
m_Root = New TreeNode(Of data_type)(node_item)
Return m_Root
End Function
Public Overrides Function ToString() As String
Return m_Root.ToString()
End Function
End Class
Public Class TreeNode(Of data_type)
Public NodeObject As data_type
Public Children As New List(Of TreeNode(Of data_type))
Public Sub New(ByVal node_object As data_type)
NodeObject = node_object
End Sub
Public Function AddChild(ByVal node_item As data_type) As TreeNode(Of data_type)
Dim child_node As New TreeNode(Of data_type)(node_item)
Children.Add(child_node)
Return child_node
End Function
Public Shadows Function ToString(Optional ByVal indent As Integer = 0) As String
Dim txt As String
txt = New String(" "c, indent) & NodeObject.ToString & vbCrLf
For Each child As TreeNode(Of data_type) In Children
txt &= child.ToString(indent + 2)
Next child
Return txt
End Function
End Class
Related examples in the same category