Using menus to change font colors and styles
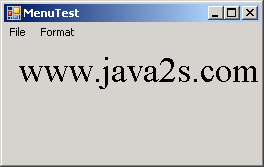
Imports System
Imports System.Drawing
Imports System.Windows.Forms
Public Class MainClass
Shared Sub Main()
Dim myform As Form = New FrmMenu()
Application.Run(myform)
End Sub ' Main
End Class
Public Class FrmMenu
Inherits Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
' display label
Friend WithEvents lblDisplay As Label
' main menu (contains file and format menus)
Friend WithEvents mnuMainMenu As MainMenu
' file menu
Friend WithEvents mnuFile As MenuItem
Friend WithEvents mnuitmAbout As MenuItem
Friend WithEvents mnuitmExit As MenuItem
' format menu (contains format and font submenus)
Friend WithEvents mnuFormat As MenuItem
' color submenu
Friend WithEvents mnuitmColor As MenuItem
Friend WithEvents mnuitmBlack As MenuItem
Friend WithEvents mnuitmBlue As MenuItem
Friend WithEvents mnuitmRed As MenuItem
Friend WithEvents mnuitmGreen As MenuItem
' font submenu
Friend WithEvents mnuitmFont As MenuItem
Friend WithEvents mnuitmTimes As MenuItem
Friend WithEvents mnuitmCourier As MenuItem
Friend WithEvents mnuitmComic As MenuItem
Friend WithEvents mnuitmDash As MenuItem
Friend WithEvents mnuitmBold As MenuItem
Friend WithEvents mnuitmItalic As MenuItem
'Required by the Windows Form Designer
Private components As System.ComponentModel.Container
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.mnuitmItalic = New System.Windows.Forms.MenuItem()
Me.mnuitmExit = New System.Windows.Forms.MenuItem()
Me.mnuitmCourier = New System.Windows.Forms.MenuItem()
Me.mnuitmBlue = New System.Windows.Forms.MenuItem()
Me.mnuitmGreen = New System.Windows.Forms.MenuItem()
Me.mnuMainMenu = New System.Windows.Forms.MainMenu()
Me.mnuFile = New System.Windows.Forms.MenuItem()
Me.mnuitmAbout = New System.Windows.Forms.MenuItem()
Me.mnuFormat = New System.Windows.Forms.MenuItem()
Me.mnuitmColor = New System.Windows.Forms.MenuItem()
Me.mnuitmBlack = New System.Windows.Forms.MenuItem()
Me.mnuitmRed = New System.Windows.Forms.MenuItem()
Me.mnuitmFont = New System.Windows.Forms.MenuItem()
Me.mnuitmTimes = New System.Windows.Forms.MenuItem()
Me.mnuitmComic = New System.Windows.Forms.MenuItem()
Me.mnuitmDash = New System.Windows.Forms.MenuItem()
Me.mnuitmBold = New System.Windows.Forms.MenuItem()
Me.lblDisplay = New System.Windows.Forms.Label()
Me.SuspendLayout()
'
'mnuitmItalic
'
Me.mnuitmItalic.Index = 5
Me.mnuitmItalic.Text = "Italic"
'
'mnuitmExit
'
Me.mnuitmExit.Index = 1
Me.mnuitmExit.Text = "Exit"
'
'mnuitmCourier
'
Me.mnuitmCourier.Index = 1
Me.mnuitmCourier.Text = "Courier"
'
'mnuitmBlue
'
Me.mnuitmBlue.Index = 1
Me.mnuitmBlue.RadioCheck = True
Me.mnuitmBlue.Text = "Blue"
'
'mnuitmGreen
'
Me.mnuitmGreen.Index = 3
Me.mnuitmGreen.RadioCheck = True
Me.mnuitmGreen.Text = "Green"
'
'mnuMainMenu
'
Me.mnuMainMenu.MenuItems.AddRange(New System.Windows.Forms.MenuItem() {Me.mnuFile, Me.mnuFormat})
'
'mnuFile
'
Me.mnuFile.Index = 0
Me.mnuFile.MenuItems.AddRange(New System.Windows.Forms.MenuItem() {Me.mnuitmAbout, Me.mnuitmExit})
Me.mnuFile.Text = "File "
'
'mnuitmAbout
'
Me.mnuitmAbout.Index = 0
Me.mnuitmAbout.Text = "About"
'
'mnuFormat
'
Me.mnuFormat.Index = 1
Me.mnuFormat.MenuItems.AddRange(New System.Windows.Forms.MenuItem() {Me.mnuitmColor, Me.mnuitmFont})
Me.mnuFormat.Text = "Format"
'
'mnuitmColor
'
Me.mnuitmColor.Index = 0
Me.mnuitmColor.MenuItems.AddRange(New System.Windows.Forms.MenuItem() {Me.mnuitmBlack, Me.mnuitmBlue, Me.mnuitmRed, Me.mnuitmGreen})
Me.mnuitmColor.Text = "Color"
'
'mnuitmBlack
'
Me.mnuitmBlack.Index = 0
Me.mnuitmBlack.RadioCheck = True
Me.mnuitmBlack.Text = "Black"
'
'mnuitmRed
'
Me.mnuitmRed.Index = 2
Me.mnuitmRed.RadioCheck = True
Me.mnuitmRed.Text = "Red"
'
'mnuitmFont
'
Me.mnuitmFont.Index = 1
Me.mnuitmFont.MenuItems.AddRange(New System.Windows.Forms.MenuItem() {Me.mnuitmTimes, Me.mnuitmCourier, Me.mnuitmComic, Me.mnuitmDash, Me.mnuitmBold, Me.mnuitmItalic})
Me.mnuitmFont.Text = "Font"
'
'mnuitmTimes
'
Me.mnuitmTimes.Index = 0
Me.mnuitmTimes.Text = "Times New Roman"
'
'mnuitmComic
'
Me.mnuitmComic.Index = 2
Me.mnuitmComic.Text = "Comic Sans"
'
'mnuitmDash
'
Me.mnuitmDash.Index = 3
Me.mnuitmDash.Text = "-"
'
'mnuitmBold
'
Me.mnuitmBold.Index = 4
Me.mnuitmBold.Text = "Bold"
'
'lblDisplay
'
Me.lblDisplay.Font = New System.Drawing.Font("Times New Roman", 26.25!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.lblDisplay.Location = New System.Drawing.Point(8, 8)
Me.lblDisplay.Name = "lblDisplay"
Me.lblDisplay.Size = New System.Drawing.Size(290, 48)
Me.lblDisplay.TabIndex = 0
Me.lblDisplay.Text = "www.java2s.com"
'
'FrmMenu
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(256, 121)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.lblDisplay})
Me.Menu = Me.mnuMainMenu
Me.Name = "FrmMenu"
Me.Text = "MenuTest"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub mnuitmAbout_Click _
(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmAbout.Click
MessageBox.Show("This is an example" & vbCrLf & _
"of using menus.", "About", MessageBoxButtons.OK, _
MessageBoxIcon.Information)
End Sub
' exit program
Private Sub mnuitmExit_Click _
(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmExit.Click
Application.Exit()
End Sub ' mnuitmExit_Click
Private Sub ClearColor()
mnuitmBlack.Checked = False
mnuitmBlue.Checked = False
mnuitmRed.Checked = False
mnuitmGreen.Checked = False
End Sub ' ClearColor
Private Sub mnuitmBlack_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmBlack.Click
ClearColor()
lblDisplay.ForeColor = Color.Black
mnuitmBlack.Checked = True
End Sub ' mnuitmBlack_Click
Private Sub mnuitmBlue_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmBlue.Click
ClearColor()
lblDisplay.ForeColor = Color.Blue
mnuitmBlue.Checked = True
End Sub
Private Sub mnuitmRed_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmRed.Click
ClearColor()
lblDisplay.ForeColor = Color.Red
mnuitmRed.Checked = True
End Sub ' mnuitmRed_Click
Private Sub mnuitmGreen_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmGreen.Click
ClearColor()
lblDisplay.ForeColor = Color.Green
mnuitmGreen.Checked = True
End Sub ' mnuitmGreen_Click
Private Sub ClearFont()
mnuitmTimes.Checked = False
mnuitmCourier.Checked = False
mnuitmComic.Checked = False
End Sub ' ClearFont
Private Sub mnuitmTimes_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmTimes.Click
ClearFont()
mnuitmTimes.Checked = True
lblDisplay.Font = New Font("Times New Roman", 30, _
lblDisplay.Font.Style)
End Sub ' mnuitmTimes_Click
Private Sub mnuitmCourier_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmCourier.Click
ClearFont()
mnuitmCourier.Checked = True
lblDisplay.Font = New Font("Courier New", 30, _
lblDisplay.Font.Style)
End Sub ' mnuitmCourier_Click
Private Sub mnuitmComic_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmComic.Click
ClearFont()
mnuitmComic.Checked = True
lblDisplay.Font = New Font("Comic Sans MS", 30, _
lblDisplay.Font.Style)
End Sub ' mnuitmComic_Click
Private Sub mnuitmBold_Click _
(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmBold.Click
mnuitmBold.Checked = Not mnuitmBold.Checked
lblDisplay.Font = New Font _
(lblDisplay.Font.FontFamily, 30, _
lblDisplay.Font.Style Xor FontStyle.Bold)
End Sub ' mnuitmBold_Click
Private Sub mnuitmItalic_Click _
(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmItalic.Click
mnuitmItalic.Checked = Not mnuitmItalic.Checked
lblDisplay.Font = New Font _
(lblDisplay.Font.FontFamily, 30, _
lblDisplay.Font.Style Xor FontStyle.Italic)
End Sub
End Class
Related examples in the same category