Property Grid Demo
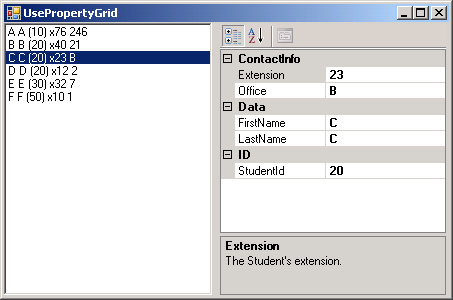
Imports System
Imports System.Data
Imports System.Windows.Forms
Imports System.Drawing
Imports System.Diagnostics
Imports System.Drawing.Printing
Imports System.ComponentModel
Public Class MainClass
Shared Sub Main()
Dim form1 As Form = New Form1
Application.Run(form1)
End Sub
End Class
Public Class Form1
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
lstStudents.Items.Add(New Student("A", "A", 10, 76, "246"))
lstStudents.Items.Add(New Student("B", "B", 20, 40, "21"))
lstStudents.Items.Add(New Student("C", "C", 20, 23, "B"))
lstStudents.Items.Add(New Student("D", "D", 20, 12, "2"))
lstStudents.Items.Add(New Student("E", "E", 30, 32, "7"))
lstStudents.Items.Add(New Student("F", "F", 50, 10, "1"))
End Sub
Private Sub lstStudents_SelectedIndexChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles lstStudents.SelectedIndexChanged
Dim s As Student = DirectCast(lstStudents.SelectedItem, Student)
PropertyGrid1.SelectedObject = s
End Sub
End Class
Public Class Student
' The FirstName property.
Private m_FirstName As String = ""
<Description("The Student's first name."), _
Category("Data"), Browsable(True)> _
Public Property FirstName() As String
Get
Return m_FirstName
End Get
Set(ByVal value As String)
m_FirstName = value
End Set
End Property
' The LastName property.
Private m_LastName As String = ""
<Description("The Student's last name."), _
Category("Data"), Browsable(True)> _
Public Property LastName() As String
Get
Return m_LastName
End Get
Set(ByVal value As String)
m_LastName = value
End Set
End Property
' The StudentId property.
Private m_StudentId As Integer = 0
<Description("The Student's ID number."), _
Category("ID")> _
Public Property StudentId() As Integer
Get
Return m_StudentId
End Get
Set(ByVal value As Integer)
m_StudentId = value
End Set
End Property
' The Extension property.
Private m_Extension As Integer = 0
<Description("The Student's extension."), _
Category("ContactInfo")> _
Public Property Extension() As Integer
Get
Return m_Extension
End Get
Set(ByVal value As Integer)
m_Extension = value
End Set
End Property
' The Office property.
Private m_Office As String = ""
<Description("The Student's building and office number."), _
Category("ContactInfo")> _
Public Property Office() As String
Get
Return m_Office
End Get
Set(ByVal value As String)
m_Office = value
End Set
End Property
Public Sub New(ByVal first_name As String, ByVal last_name As String, ByVal id As Integer, ByVal ext As Integer, ByVal the_office As String)
FirstName = first_name
LastName = last_name
StudentId = id
Extension = ext
Office = the_office
End Sub
Public Overrides Function ToString() As String
Return _
FirstName & " " & _
LastName & " (" & _
StudentId.ToString() & ") x" & _
Extension.ToString & " " & _
Office
End Function
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Public Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.PropertyGrid1 = New System.Windows.Forms.PropertyGrid
Me.lstStudents = New System.Windows.Forms.ListBox
Me.SuspendLayout()
'
'PropertyGrid1
'
Me.PropertyGrid1.Anchor = CType(((System.Windows.Forms.AnchorStyles.Top Or System.Windows.Forms.AnchorStyles.Bottom) _
Or System.Windows.Forms.AnchorStyles.Right), System.Windows.Forms.AnchorStyles)
Me.PropertyGrid1.Location = New System.Drawing.Point(216, 0)
Me.PropertyGrid1.Name = "PropertyGrid1"
Me.PropertyGrid1.Size = New System.Drawing.Size(226, 272)
Me.PropertyGrid1.TabIndex = 0
'
'lstStudents
'
Me.lstStudents.Anchor = CType(((System.Windows.Forms.AnchorStyles.Top Or System.Windows.Forms.AnchorStyles.Bottom) _
Or System.Windows.Forms.AnchorStyles.Left), System.Windows.Forms.AnchorStyles)
Me.lstStudents.FormattingEnabled = True
Me.lstStudents.IntegralHeight = False
Me.lstStudents.Location = New System.Drawing.Point(0, 0)
Me.lstStudents.Name = "lstStudents"
Me.lstStudents.Size = New System.Drawing.Size(208, 272)
Me.lstStudents.TabIndex = 1
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 13.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(445, 273)
Me.Controls.Add(Me.lstStudents)
Me.Controls.Add(Me.PropertyGrid1)
Me.Name = "Form1"
Me.Text = "UsePropertyGrid"
Me.ResumeLayout(False)
End Sub
Friend WithEvents PropertyGrid1 As System.Windows.Forms.PropertyGrid
Friend WithEvents lstStudents As System.Windows.Forms.ListBox
End Class
Related examples in the same category