Read from a binary file
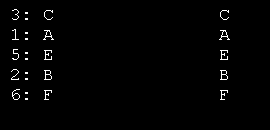
Imports System
Imports System.IO
Imports System.Windows.Forms
Public Class MainClass
Shared Sub Main()
Dim emp As New Employee
Dim file_num As Integer = FreeFile()
FileOpen(file_num, "MYFILE.DAT", OpenMode.Random, _
OpenAccess.ReadWrite, OpenShare.Shared, _
Len(emp))
FilePut(file_num, New Employee(1, "A", "A"))
FilePut(file_num, New Employee(2, "B", "B"))
FilePut(file_num, New Employee(3, "C", "C"))
FilePut(file_num, New Employee(4, "D", "D"))
FilePut(file_num, New Employee(5, "E", "E"))
FilePut(file_num, New Employee(6, "F", "F"))
Dim obj As ValueType = DirectCast(emp, ValueType)
For Each i As Integer In New Integer() {3, 1, 5, 2, 6}
FileGet(file_num, obj, i)
emp = DirectCast(obj, Employee)
Console.WriteLine(emp.ToString())
Next i
' Close the file.
FileClose(file_num)
End Sub
End Class
Public Structure Employee
Public ID As Integer
<VBFixedString(15)> Public FirstName As String
<VBFixedString(15)> Public LastName As String
Public Sub New(ByVal new_id As Integer, ByVal first_name As String, _
ByVal last_name As String)
ID = new_id
FirstName = first_name
LastName = last_name
End Sub
Public Overrides Function ToString() As String
Return ID & ": " & FirstName & " " & LastName
End Function
End Structure
Related examples in the same category