Request garbage collection
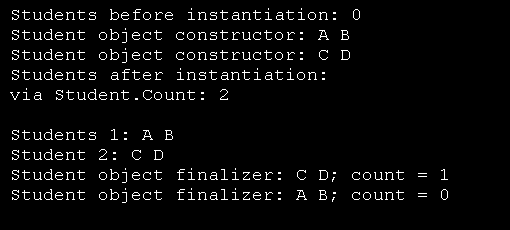
Imports System
Public Class MainClass
Shared Sub Main(ByVal args As String())
Console.WriteLine("Students before instantiation: " & Student.Count)
Dim student1 As Student = New Student("A", "B")
Dim student2 As Student = New Student("C", "D")
' output of student2 after instantiation
Console.WriteLine("Students after instantiation: " & vbCrLf & _
"via Student.Count: " & Student.Count)
' display name of first and second student
Console.WriteLine(vbCrLf & "Students 1: " & _
student1.FirstName & " " & student1.LastName & _
vbCrLf & "Student 2: " & student2.FirstName & " " & _
student2.LastName)
' mark student1 and student2 for garbage collection
student1 = Nothing
student2 = Nothing
System.GC.Collect() ' request garbage collection
End Sub
End Class
' Class Student uses Shared variable.
Class Student
Inherits Object
Private mFirstName As String
Private mLastName As String
' number of objects in memory
Private Shared mCount As Integer
' Student constructor
Public Sub New(ByVal firstNameValue As String, _
ByVal lastNameValue As String)
mFirstName = firstNameValue
mLastName = lastNameValue
mCount += 1 ' increment shared count of students
Console.WriteLine _
("Student object constructor: " & mFirstName & _
" " & mLastName)
End Sub ' New
' finalizer method decrements Shared count of students
Protected Overrides Sub Finalize()
mCount -= 1 ' decrement mCount, resulting in one fewer object
Console.WriteLine _
("Student object finalizer: " & mFirstName & _
" " & mLastName & "; count = " & mCount)
End Sub ' Finalize
' return first name
Public ReadOnly Property FirstName() As String
Get
Return mFirstName
End Get
End Property ' FirstName
' return last name
Public ReadOnly Property LastName() As String
Get
Return mLastName
End Get
End Property ' LastName
' property Count
Public Shared ReadOnly Property Count() As Integer
Get
Return mCount
End Get
End Property ' Count
End Class ' Student
Related examples in the same category