A strongly typed collection of your own Objects
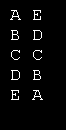
Imports System
Imports System.Collections
Imports System.Collections.Generic
Public Class MainClass
Shared Sub Main(ByVal args As String())
Dim student_list As New StudentCollection
student_list.Add(New Student("A", "E"))
student_list.Add(New Student("B", "D"))
student_list.Add(New Student("C", "C"))
student_list.Add(New Student("D", "B"))
student_list.Add(New Student("E", "A"))
For Each student As Student In student_list
Console.WriteLine(student.ToString)
Next student
End Sub
End Class
Public Class Student
Private m_FirstName As String
Private m_LastName As String
Public Sub New(ByVal first_name As String, ByVal last_name As String)
m_FirstName = first_name
m_LastName = last_name
End Sub
Public Overrides Function ToString() As String
Return m_FirstName & " " & m_LastName
End Function
End Class
' A strongly typed collection of Students.
Public Class StudentCollection
Inherits CollectionBase
' Add an Student.
Public Sub Add(ByVal value As Student)
List.Add(value)
End Sub
' Return True if the collection contains this employee.
Public Function Contains(ByVal value As Student) As Boolean
Return List.Contains(value)
End Function
' Return this Student's index.
Public Function IndexOf(ByVal value As Student) As Integer
Return List.IndexOf(value)
End Function
' Insert a new Student.
Public Sub Insert(ByVal index As Integer, ByVal value As Student)
List.Insert(index, value)
End Sub
' Return the Student at this position.
Default Public ReadOnly Property Item(ByVal index As Integer) As Student
Get
Return DirectCast(List.Item(index), Student)
End Get
End Property
' Remove an Student.
Public Sub Remove(ByVal value As Student)
List.Remove(value)
End Sub
End Class
Related examples in the same category