Array Sort Object who implements the IComparable
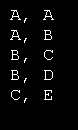
Imports System
Public Class MainClass
Shared Sub Main(ByVal args As String())
Dim student(4) As Student
student(0) = New Student("A", "A")
student(1) = New Student("B", "A")
student(2) = New Student("C", "B")
student(3) = New Student("D", "B")
student(4) = New Student("E", "C")
' Sort.
Array.Sort(student)
' Display the results.
Dim txt As String = ""
For i As Integer = 0 To student.GetUpperBound(0)
Console.WriteLine( student(i).ToString() )
Next i
End Sub
End Class
Public Class Student
Implements IComparable
Public FirstName As String
Public LastName As String
Public Sub New(ByVal first_name As String, ByVal last_name As String)
FirstName = first_name
LastName = last_name
End Sub
Public Overrides Function ToString() As String
Return LastName & ", " & FirstName
End Function
Public Function CompareTo(ByVal obj As Object) As Integer _
Implements System.IComparable.CompareTo
Dim other_Student As Student = DirectCast(obj, Student)
Return String.Compare(Me.ToString, other_Student.ToString)
End Function
End Class
Related examples in the same category