Class Inheritance Demo
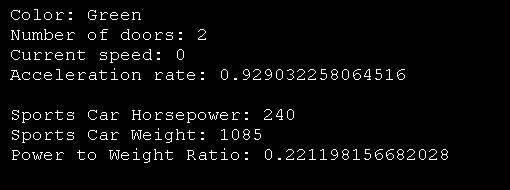
Imports System
Imports System.Collections
Imports System.ComponentModel
Imports System.Windows.Forms
Imports System.Data
Imports System.Configuration
Imports System.Resources
Imports System.Drawing
Imports System.Drawing.Drawing2D
Imports System.IO
Imports System.Drawing.Printing
Module Module1
Sub Main()
Using objCar As New SportsCar
'Set the horsepower and weight(kg)
objCar.HorsePower = 240
objCar.Weight = 1085
'Display the details of the car
DisplayCarDetails(objCar)
DisplaySportsCarDetails(objCar)
End Using
'Wait for input from the user
Console.ReadLine()
End Sub
'DisplayCarDetails - procedure that displays a car's details
Sub DisplayCarDetails(ByVal theCar As Car)
'Display the details of the car
Console.WriteLine("Color: " & theCar.Color)
Console.WriteLine("Number of doors: " & theCar.NumberOfDoors)
Console.WriteLine("Current speed: " & theCar.Speed)
Console.WriteLine("Acceleration rate: " & _
theCar.CalculateAccelerationRate)
End Sub
'DisplaySportsCarDetails - procedure that displays a sports car's details
Sub DisplaySportsCarDetails(ByVal theCar As SportsCar)
'Display the details of the sports car
Console.WriteLine()
Console.WriteLine("Sports Car Horsepower: " & theCar.HorsePower)
Console.WriteLine("Sports Car Weight: " & theCar.Weight)
Console.WriteLine("Power to Weight Ratio: " & theCar.GetPowerToWeightRatio)
End Sub
End Module
Public Class Car
Implements IDisposable
Public Color As String
Public HorsePower As Integer
Private _speed As Integer
Private _numberOfDoors As Integer
Public ReadOnly Property Speed() As Integer
Get
Return _speed
End Get
End Property
Public Sub Accelerate(ByVal accelerateBy As Integer)
_speed += accelerateBy
End Sub
Public Property NumberOfDoors() As Integer
Get
Return _numberOfDoors
End Get
Set(ByVal value As Integer)
If value >= 2 And value <= 5 Then
_numberOfDoors = value
End If
End Set
End Property
Public Function IsMoving() As Boolean
If Speed = 0 Then
Return False
Else
Return True
End If
End Function
Public Sub New()
Color = "White"
_speed = 0
_numberOfDoors = 5
End Sub
Public Overridable Function CalculateAccelerationRate() As Double
Return 4.2
End Function
Private disposed As Boolean = False
' IDisposable
Private Overloads Sub Dispose(ByVal disposing As Boolean)
If Not Me.disposed Then
If disposing Then
' TODO: put code to dispose managed resources
End If
' TODO: put code to free unmanaged resources here
End If
Me.disposed = True
End Sub
#Region " IDisposable Support "
' This code added by Visual Basic to correctly implement the disposable pattern.
Public Overloads Sub Dispose() Implements IDisposable.Dispose
' Do not change this code. Put cleanup code in Dispose(ByVal disposing As Boolean) above.
Dispose(True)
GC.SuppressFinalize(Me)
End Sub
Protected Overrides Sub Finalize()
' Do not change this code. Put cleanup code in Dispose(ByVal disposing As Boolean) above.
Dispose(False)
MyBase.Finalize()
End Sub
#End Region
End Class
Public Class SportsCar
Inherits Car
Public Weight As Integer
Public Function GetPowerToWeightRatio() As Double
Return CType(HorsePower, Double) / CType(Weight, Double)
End Function
Public Sub New()
'Change the default values
Color = "Green"
NumberOfDoors = 2
End Sub
Public Overrides Function CalculateAccelerationRate() As Double
'You'll assume the same 4.2 value, but you'll multiply it
'by the power/weight ratio
Return 4.2 * GetPowerToWeightRatio()
End Function
End Class
Related examples in the same category