Using different colors in Visual Basic
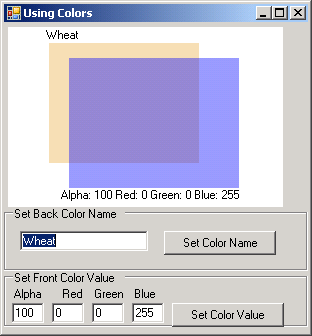
Imports System
Imports System.Drawing
Imports System.Windows.Forms
Public Class MainClass
Shared Sub Main()
Dim myform As Form = New FrmColorForm()
Application.Run(myform)
End Sub ' Main
End Class
Public Class FrmColorForm
Inherits System.Windows.Forms.Form
' input text boxes
Friend WithEvents txtColorName As TextBox
Friend WithEvents txtGreenBox As TextBox
Friend WithEvents txtRedBox As TextBox
Friend WithEvents txtAlphaBox As TextBox
Friend WithEvents txtBlueBox As TextBox
' set color command buttons
Friend WithEvents cmdColorName As Button
Friend WithEvents cmdColorValue As Button
' color labels
Friend WithEvents lblBlue As Label
Friend WithEvents lblGreen As Label
Friend WithEvents lblRed As Label
Friend WithEvents lblAlpha As Label
' group boxes
Friend WithEvents nameBox As GroupBox
Friend WithEvents colorValueGroup As GroupBox
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.Container
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.nameBox = New System.Windows.Forms.GroupBox()
Me.cmdColorName = New System.Windows.Forms.Button()
Me.txtColorName = New System.Windows.Forms.TextBox()
Me.colorValueGroup = New System.Windows.Forms.GroupBox()
Me.lblBlue = New System.Windows.Forms.Label()
Me.lblGreen = New System.Windows.Forms.Label()
Me.lblRed = New System.Windows.Forms.Label()
Me.lblAlpha = New System.Windows.Forms.Label()
Me.txtBlueBox = New System.Windows.Forms.TextBox()
Me.txtGreenBox = New System.Windows.Forms.TextBox()
Me.txtRedBox = New System.Windows.Forms.TextBox()
Me.txtAlphaBox = New System.Windows.Forms.TextBox()
Me.cmdColorValue = New System.Windows.Forms.Button()
Me.nameBox.SuspendLayout()
Me.colorValueGroup.SuspendLayout()
Me.SuspendLayout()
'
'nameBox
'
Me.nameBox.Controls.AddRange(New System.Windows.Forms.Control() {Me.cmdColorName, Me.txtColorName})
Me.nameBox.Location = New System.Drawing.Point(0, 184)
Me.nameBox.Name = "nameBox"
Me.nameBox.Size = New System.Drawing.Size(304, 64)
Me.nameBox.TabIndex = 0
Me.nameBox.TabStop = False
Me.nameBox.Text = "Set Back Color Name"
'
'cmdColorName
'
Me.cmdColorName.Location = New System.Drawing.Point(160, 24)
Me.cmdColorName.Name = "cmdColorName"
Me.cmdColorName.Size = New System.Drawing.Size(112, 24)
Me.cmdColorName.TabIndex = 1
Me.cmdColorName.Text = "Set Color Name"
'
'txtColorName
'
Me.txtColorName.Location = New System.Drawing.Point(16, 24)
Me.txtColorName.Name = "txtColorName"
Me.txtColorName.Size = New System.Drawing.Size(128, 20)
Me.txtColorName.TabIndex = 0
Me.txtColorName.Text = "Wheat"
'
'colorValueGroup
'
Me.colorValueGroup.Controls.AddRange(New System.Windows.Forms.Control() {Me.lblBlue, Me.lblGreen, Me.lblRed, Me.lblAlpha, Me.txtBlueBox, Me.txtGreenBox, Me.txtRedBox, Me.txtAlphaBox, Me.cmdColorValue})
Me.colorValueGroup.Location = New System.Drawing.Point(0, 248)
Me.colorValueGroup.Name = "colorValueGroup"
Me.colorValueGroup.Size = New System.Drawing.Size(304, 56)
Me.colorValueGroup.TabIndex = 1
Me.colorValueGroup.TabStop = False
Me.colorValueGroup.Text = "Set Front Color Value"
'
'lblBlue
'
Me.lblBlue.Location = New System.Drawing.Point(128, 16)
Me.lblBlue.Name = "lblBlue"
Me.lblBlue.Size = New System.Drawing.Size(40, 16)
Me.lblBlue.TabIndex = 7
Me.lblBlue.Text = "Blue"
'
'lblGreen
'
Me.lblGreen.Location = New System.Drawing.Point(88, 16)
Me.lblGreen.Name = "lblGreen"
Me.lblGreen.Size = New System.Drawing.Size(40, 16)
Me.lblGreen.TabIndex = 6
Me.lblGreen.Text = "Green"
'
'lblRed
'
Me.lblRed.Location = New System.Drawing.Point(56, 16)
Me.lblRed.Name = "lblRed"
Me.lblRed.Size = New System.Drawing.Size(32, 16)
Me.lblRed.TabIndex = 5
Me.lblRed.Text = "Red"
'
'lblAlpha
'
Me.lblAlpha.Location = New System.Drawing.Point(8, 16)
Me.lblAlpha.Name = "lblAlpha"
Me.lblAlpha.Size = New System.Drawing.Size(40, 16)
Me.lblAlpha.TabIndex = 4
Me.lblAlpha.Text = "Alpha"
'
'txtBlueBox
'
Me.txtBlueBox.Location = New System.Drawing.Point(128, 32)
Me.txtBlueBox.Name = "txtBlueBox"
Me.txtBlueBox.Size = New System.Drawing.Size(32, 20)
Me.txtBlueBox.TabIndex = 3
Me.txtBlueBox.Text = "255"
'
'txtGreenBox
'
Me.txtGreenBox.Location = New System.Drawing.Point(88, 32)
Me.txtGreenBox.Name = "txtGreenBox"
Me.txtGreenBox.Size = New System.Drawing.Size(32, 20)
Me.txtGreenBox.TabIndex = 2
Me.txtGreenBox.Text = "0"
'
'txtRedBox
'
Me.txtRedBox.Location = New System.Drawing.Point(48, 32)
Me.txtRedBox.Name = "txtRedBox"
Me.txtRedBox.Size = New System.Drawing.Size(32, 20)
Me.txtRedBox.TabIndex = 1
Me.txtRedBox.Text = "0"
'
'txtAlphaBox
'
Me.txtAlphaBox.Location = New System.Drawing.Point(8, 32)
Me.txtAlphaBox.Name = "txtAlphaBox"
Me.txtAlphaBox.Size = New System.Drawing.Size(32, 20)
Me.txtAlphaBox.TabIndex = 0
Me.txtAlphaBox.Text = "100"
'
'cmdColorValue
'
Me.cmdColorValue.Location = New System.Drawing.Point(168, 32)
Me.cmdColorValue.Name = "cmdColorValue"
Me.cmdColorValue.Size = New System.Drawing.Size(112, 24)
Me.cmdColorValue.TabIndex = 3
Me.cmdColorValue.Text = "Set Color Value"
'
'FrmColorForm
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(304, 309)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.colorValueGroup, Me.nameBox})
Me.Name = "FrmColorForm"
Me.Text = "Using Colors"
Me.nameBox.ResumeLayout(False)
Me.colorValueGroup.ResumeLayout(False)
Me.ResumeLayout(False)
End Sub
#End Region
' color for back rectangle
Private mBehindColor As Color = Color.Wheat
' color for front rectangle
Private mFrontColor As Color = Color.FromArgb(100, 0, 0, 255)
' overrides Form OnPaint method
Protected Overrides Sub OnPaint(ByVal e As PaintEventArgs)
Dim graphicsObject As Graphics = e.Graphics ' get graphics
Dim textBrush As SolidBrush = New SolidBrush(Color.Black)
Dim brush As SolidBrush = New SolidBrush(Color.White)
graphicsObject.FillRectangle(brush, 4, 4, 275, 180)
graphicsObject.DrawString(mBehindColor.Name, Me.Font, _
textBrush, 40, 5)
brush.Color = mBehindColor
graphicsObject.FillRectangle(brush, 45, 20, 150, 120)
graphicsObject.DrawString("Alpha: " & mFrontColor.A & _
" Red: " & mFrontColor.R & " Green: " & mFrontColor.G _
& " Blue: " & mFrontColor.B, Me.Font, textBrush, _
55, 165)
brush.Color = mFrontColor
graphicsObject.FillRectangle(brush, 65, 35, 170, 130)
End Sub
Private Sub cmdColorValue_Click(ByVal sender As _
System.Object, ByVal e As System.EventArgs) _
Handles cmdColorValue.Click
mFrontColor = Color.FromArgb(txtAlphaBox.Text, _
txtRedBox.Text, txtGreenBox.Text, txtBlueBox.Text)
Invalidate()
End Sub
Private Sub cmdColorName_Click(ByVal sender As _
System.Object, ByVal e As System.EventArgs) _
Handles cmdColorName.Click
mBehindColor = Color.FromName(txtColorName.Text)
Invalidate()
End Sub
End Class
Related examples in the same category