Property Table: Use JTable to display and edit properties
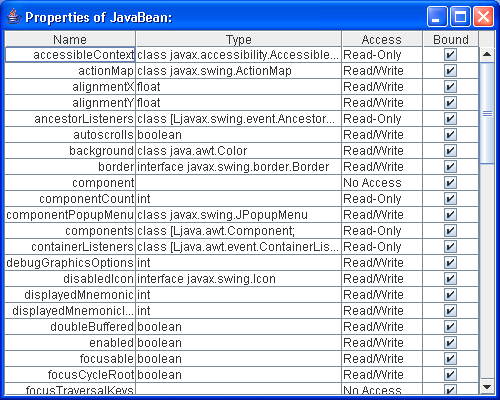
/*
* Copyright (c) 2000 David Flanagan. All rights reserved.
* This code is from the book Java Examples in a Nutshell, 2nd Edition.
* It is provided AS-IS, WITHOUT ANY WARRANTY either expressed or implied.
* You may study, use, and modify it for any non-commercial purpose.
* You may distribute it non-commercially as long as you retain this notice.
* For a commercial use license, or to purchase the book (recommended),
* visit http://www.davidflanagan.com/javaexamples2.
*/
import java.beans.BeanInfo;
import java.beans.IntrospectionException;
import java.beans.Introspector;
import java.beans.PropertyDescriptor;
import java.util.Arrays;
import java.util.Comparator;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.SwingConstants;
import javax.swing.table.AbstractTableModel;
import javax.swing.table.DefaultTableCellRenderer;
import javax.swing.table.TableColumn;
import javax.swing.table.TableColumnModel;
/**
* This class is a JTable subclass that displays a table of the JavaBeans
* properties of any specified class.
*/
public class PropertyTable extends JTable {
/** This main method allows the class to be demonstrated standalone */
public static void main(String[] args) {
// Specify the name of the class as a command-line argument
Class beanClass = null;
try {
// Use reflection to get the Class from the classname
beanClass = Class.forName("javax.swing.JLabel");
} catch (Exception e) { // Report errors
System.out.println("Can't find specified class: " + e.getMessage());
System.out.println("Usage: java TableDemo <JavaBean class name>");
System.exit(0);
}
// Create a table to display the properties of the specified class
JTable table = new PropertyTable(beanClass);
// Then put the table in a scrolling window, put the scrolling
// window into a frame, and pop it all up on to the screen
JScrollPane scrollpane = new JScrollPane(table);
JFrame frame = new JFrame("Properties of JavaBean: ");
frame.getContentPane().add(scrollpane);
frame.setSize(500, 400);
frame.setVisible(true);
}
/**
* This constructor method specifies what data the table will display (the
* table model) and uses the TableColumnModel to customize the way that the
* table displays it. The hard work is done by the TableModel implementation
* below.
*/
public PropertyTable(Class beanClass) {
// Set the data model for this table
try {
setModel(new JavaBeanPropertyTableModel(beanClass));
} catch (IntrospectionException e) {
System.err.println("WARNING: can't introspect: " + beanClass);
}
// Tweak the appearance of the table by manipulating its column model
TableColumnModel colmodel = getColumnModel();
// Set column widths
colmodel.getColumn(0).setPreferredWidth(125);
colmodel.getColumn(1).setPreferredWidth(200);
colmodel.getColumn(2).setPreferredWidth(75);
colmodel.getColumn(3).setPreferredWidth(50);
// Right justify the text in the first column
TableColumn namecol = colmodel.getColumn(0);
DefaultTableCellRenderer renderer = new DefaultTableCellRenderer();
renderer.setHorizontalAlignment(SwingConstants.RIGHT);
namecol.setCellRenderer(renderer);
}
/**
* This class implements TableModel and represents JavaBeans property data
* in a way that the JTable component can display. If you've got some type
* of tabular data to display, implement a TableModel class to describe that
* data, and the JTable component will be able to display it.
*/
static class JavaBeanPropertyTableModel extends AbstractTableModel {
PropertyDescriptor[] properties; // The properties to display
/**
* The constructor: use the JavaBeans introspector mechanism to get
* information about all the properties of a bean. Once we've got this
* information, the other methods will interpret it for JTable.
*/
public JavaBeanPropertyTableModel(Class beanClass)
throws java.beans.IntrospectionException {
// Use the introspector class to get "bean info" about the class.
BeanInfo beaninfo = Introspector.getBeanInfo(beanClass);
// Get the property descriptors from that BeanInfo class
properties = beaninfo.getPropertyDescriptors();
// Now do a case-insensitive sort by property name
// The anonymous Comparator implementation specifies how to
// sort PropertyDescriptor objects by name
Arrays.sort(properties, new Comparator() {
public int compare(Object p, Object q) {
PropertyDescriptor a = (PropertyDescriptor) p;
PropertyDescriptor b = (PropertyDescriptor) q;
return a.getName().compareToIgnoreCase(b.getName());
}
public boolean equals(Object o) {
return o == this;
}
});
}
// These are the names of the columns represented by this TableModel
static final String[] columnNames = new String[] { "Name", "Type",
"Access", "Bound" };
// These are the types of the columns represented by this TableModel
static final Class[] columnTypes = new Class[] { String.class,
Class.class, String.class, Boolean.class };
// These simple methods return basic information about the table
public int getColumnCount() {
return columnNames.length;
}
public int getRowCount() {
return properties.length;
}
public String getColumnName(int column) {
return columnNames[column];
}
public Class getColumnClass(int column) {
return columnTypes[column];
}
/**
* This method returns the value that appears at the specified row and
* column of the table
*/
public Object getValueAt(int row, int column) {
PropertyDescriptor prop = properties[row];
switch (column) {
case 0:
return prop.getName();
case 1:
return prop.getPropertyType();
case 2:
return getAccessType(prop);
case 3:
return new Boolean(prop.isBound());
default:
return null;
}
}
// A helper method called from getValueAt() above
String getAccessType(PropertyDescriptor prop) {
java.lang.reflect.Method reader = prop.getReadMethod();
java.lang.reflect.Method writer = prop.getWriteMethod();
if ((reader != null) && (writer != null))
return "Read/Write";
else if (reader != null)
return "Read-Only";
else if (writer != null)
return "Write-Only";
else
return "No Access"; // should never happen
}
}
}
Related examples in the same category
1. | Creating a JTable Component | | |
2. | Creates tables that allow rows and columns to be added or deleted | | |
3. | Build a table from list data and column names | | |
4. | Getting the Number of Rows and Columns in a JTable Component | | |
5. | Appending a Row to a JTable Component | | |
6. | Add columns to a table through DefaultTableModel | | |
7. | Insert a row to a table through DefaultTableModel | | |
8. | Insert a row to a table through DefaultTableModel at specified row | | |
9. | Scroll Table Sample | | 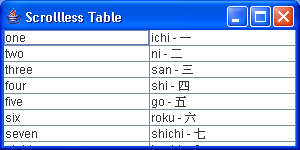 |
10. | Simple demonstration of JTable | | 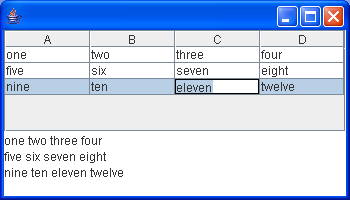 |
11. | Create a table with two dimensional array | | 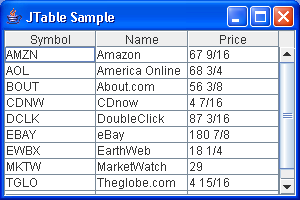 |
12. | Create table with Unicode data | | 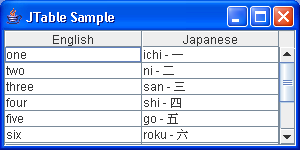 |
13. | Resize Table | |  |
14. | Expense Table | | 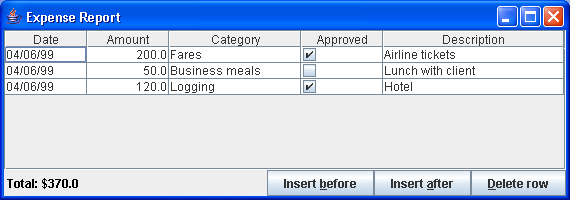 |
15. | StockTable 6: Action and Dynamic Display | |  |
16. | JTable selection events | | 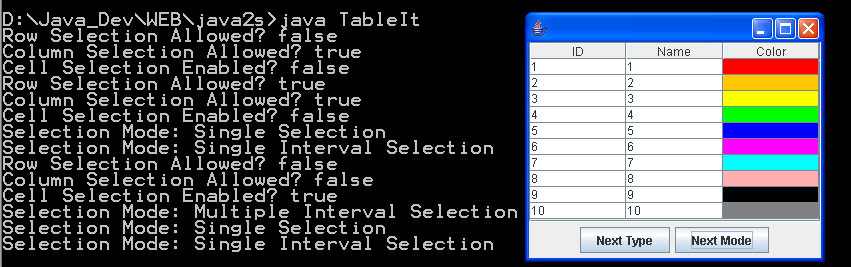 |
17. | A table with the ability to play with row and column selections | | 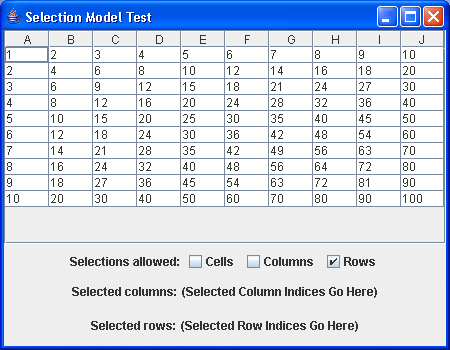 |
18. | Table Selection mode | | 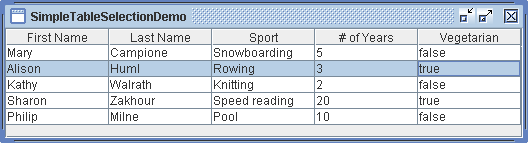 |
19. | Display ResultSet in Table (JTable) | | |
20. | JTable(Table) with JDBC and ResultSet | | 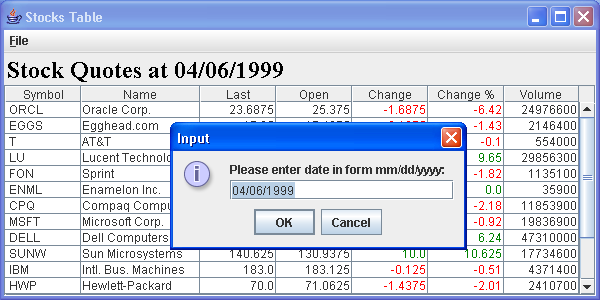 |
21. | List UI Properties in a JTable and sortable | | 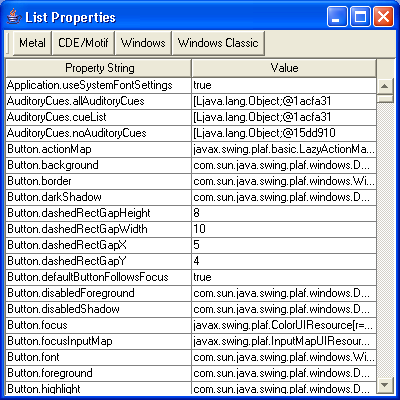 |
22. | Table with tool tips for both cells and column headers | | 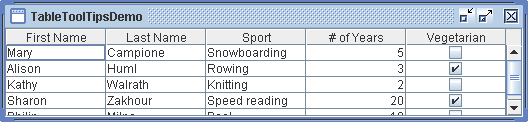 |
23. | StockTable 4: Table Sorter | |  |
24. | JTable sorter:click the table header to sort a column and a table | | 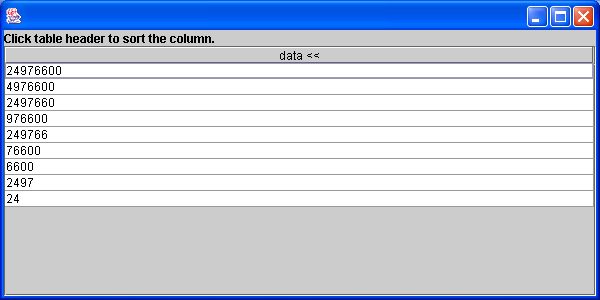 |
25. | Creating image out of a JTable | | |
26. | JTable.setSelectionMode(ListSelectionModel.MULTIPLE_INTERVAL_SELECTION); | | |
27. | JTable.setSelectionMode(ListSelectionModel.SINGLE_SELECTION); | | |
28. | JTable.setColumnSelectionAllowed(boolean b); | | |
29. | JTable.setRowSelectionAllowed(boolean b); | | |
30. | JTable.setCellSelectionEnabled(boolean b); | | |
31. | Handle selection and model change events for a JTable. | | |
32. | ListSelectionModel rowSelMod = JTable.getSelectionModel(); | | |
33. | ListSelectionModel colSelMod = JTable.getColumnModel().getSelectionModel(); | | |
34. | JTree.getModel().addTreeModelListener(new TreeModelListener()) | | |
35. | Move the last visible column so it becomes the first visible column | | |
36. | the last column is moved to the first position | | |
37. | Allowing the User to Resize a Column in a JTable Component | | |
38. | Retrieve the value in the visible cell (1,2) in a JTable | | |
39. | Retrieve the value in cell (1,2) from the model | | |
40. | Change a cell in the 2nd visible column | | |
41. | Change a cell in the 3rd column in the model | | |
42. | Showing the Table Header in a Non-Scrollable JTable Component | | |
43. | Changing the Name of a Column in a JTable Component | | |
44. | Displaying an Icon in a Column Head of a JTable Component | | |
45. | Implementing Variable-Height Column Headers in a JTable Component | | |
46. | Removing the Column Headers from a Scrollable in a JTable Component | | |
47. | Creating a Custom Column Header Renderer in a JTable Component | | |
48. | Setting Column Header Tool Tips in a JTable Components | | |
49. | Setting Tool Tips on Cells in a JTable Component | | |
50. | Enable row selection (default) in a JTable | | |
51. | Enable column selection in a JTable | | |
52. | Enable cell selection in a JTable | | |
53. | Get default selection mode:MULTIPLE_INTERVAL_SELECTION | | |
54. | Allow only single a selection | | |
55. | Allow selection to span one contiguous set of rows, visible columns, or block of cells | | |
56. | Allow multiple selections of rows, visible columns, or cell blocks (default) | | |
57. | Select a column - column 0 in a JTable | | |
58. | Select an additional range of columns - columns 1 to 2 | | |
59. | Deselect a range of columns - columns 0 to 1 | | |
60. | Select a row - row 0 | | |
61. | Select an additional range of rows - rows 1 to 2 | | |
62. | Deselect a range of rows - rows 0 to 1 | | |
63. | Select a cell: cell (2,1) | | |
64. | Extend the selection to include all cells (5,3) | | |
65. | Deselect a cell: cell (3,2), All cells in the row and column containing (3,2) are deselected. | | |
66. | Toggles the selection state, if it were called again, it exactly reverses the first call. | | |
67. | Select all cells | | |
68. | Deselect all cells | | |
69. | Column selection is enabled, get the indices of the selected columns | | |
70. | Row selection is enabled, Get the indices of the selected rows | | |
71. | Get selected row and selected index | | |
72. | Get the min and max ranges of selected cells | | |
73. | Check each cell in the min and max ranges of selected cells | | |
74. | Disabling Selections in a JTable Component | | |
75. | Getting the Anchor Cell in a JTable Component | | |
76. | Creating a Scrollable JTable Component | | |
77. | Disable auto resizing to make the table horizontal scrollable | | |
78. | Determining If a Cell Is Visible in a JTable Component | | |
79. | Making a Cell Visible in a JTable Component | | |
80. | Scrolling a Cell to the Center of a JTable Component | | |
81. | Show both horizontal and vertical grid lines (the default) | | |
82. | Don't show any grid lines | | |
83. | Show only vertical grid lines | | |
84. | Show only horizontal grid lines | | |
85. | Set the grid color | | |
86. | Getting the Gap Size Between Cells in a JTable Component | | |
87. | Add 5 spaces to the left and right sides of a cell. | | |
88. | Increase the row height | | |
89. | Programmatically Starting Cell Editing in a JTable Component | | |
90. | Save the current value in the cell being edited and stops the editing process | | |
91. | Discard any changes made by the user and stops the editing process | | |
92. | Disabling User Edits in a JTable | | |
93. | Disabling User Edits in a JTable with DefaultTableModel | | |
94. | Sorting the Rows in a JTable Component Based on a Column | | |
95. | Sorting a Column in a JTable Component | | |
96. | Listening for Selection Events in a JTable Component | | |
97. | Listening for Changes to the Rows and Columns of a JTable Component | | |
98. | Listening for Column-Related Changes in a JTable Component | | |
99. | Listening for Clicks on a Column Header in a JTable Component | | |
100. | Sorting and Filtering Tables | | |
101. | Use a regexFilter to filter table content | | |
102. | Creating a JTable with rows of variable height | | |
103. | Scroll JTable To Center | | |