OptionPane Sample: simple dialog
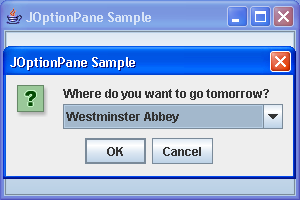
import java.awt.BorderLayout;
import java.awt.Component;
import java.awt.Container;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
public class OptionPaneSample {
public static void main(String args[]) {
JFrame f = new JFrame("JOptionPane Sample");
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Container content = f.getContentPane();
JButton button = new JButton("Ask");
ActionListener actionListener = new ActionListener() {
public void actionPerformed(ActionEvent actionEvent) {
Component source = (Component) actionEvent.getSource();
Object response = JOptionPane.showInputDialog(source,
"Where do you want to go tomorrow?",
"JOptionPane Sample", JOptionPane.QUESTION_MESSAGE,
null, new String[] { "A","B", "C","D","E" },"E");
System.out.println("Response: " + response);
}
};
button.addActionListener(actionListener);
content.add(button, BorderLayout.CENTER);
f.setSize(300, 200);
f.setVisible(true);
}
}
Related examples in the same category