Controlling the scrollbars in a JScrollPane
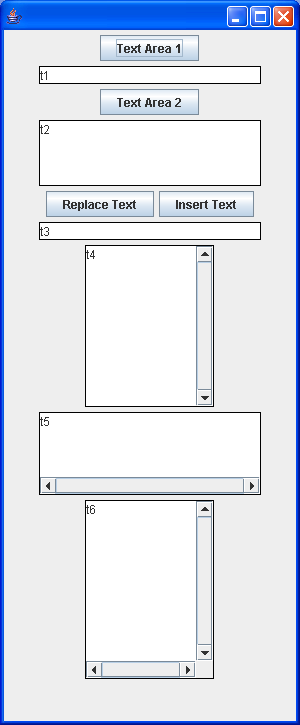
// : c14:JScrollPanes.java
// Controlling the scrollbars in a JScrollPane.
// <applet code=JScrollPanes width=300 height=725></applet>
// From 'Thinking in Java, 3rd ed.' (c) Bruce Eckel 2002
// www.BruceEckel.com. See copyright notice in CopyRight.txt.
import java.awt.Color;
import java.awt.Container;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.BorderFactory;
import javax.swing.JApplet;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.border.Border;
public class JScrollPanes extends JApplet {
private JButton b1 = new JButton("Text Area 1"), b2 = new JButton(
"Text Area 2"), b3 = new JButton("Replace Text"), b4 = new JButton(
"Insert Text");
private JTextArea t1 = new JTextArea("t1", 1, 20), t2 = new JTextArea("t2",
4, 20), t3 = new JTextArea("t3", 1, 20), t4 = new JTextArea("t4",
10, 10), t5 = new JTextArea("t5", 4, 20), t6 = new JTextArea("t6",
10, 10);
private JScrollPane sp3 = new JScrollPane(t3,
JScrollPane.VERTICAL_SCROLLBAR_NEVER,
JScrollPane.HORIZONTAL_SCROLLBAR_NEVER), sp4 = new JScrollPane(t4,
JScrollPane.VERTICAL_SCROLLBAR_ALWAYS,
JScrollPane.HORIZONTAL_SCROLLBAR_NEVER), sp5 = new JScrollPane(t5,
JScrollPane.VERTICAL_SCROLLBAR_NEVER,
JScrollPane.HORIZONTAL_SCROLLBAR_ALWAYS), sp6 = new JScrollPane(t6,
JScrollPane.VERTICAL_SCROLLBAR_ALWAYS,
JScrollPane.HORIZONTAL_SCROLLBAR_ALWAYS);
class B1L implements ActionListener {
public void actionPerformed(ActionEvent e) {
t5.append(t1.getText() + "\n");
}
}
class B2L implements ActionListener {
public void actionPerformed(ActionEvent e) {
t2.setText("Inserted by Button 2");
t2.append(": " + t1.getText());
t5.append(t2.getText() + "\n");
}
}
class B3L implements ActionListener {
public void actionPerformed(ActionEvent e) {
String s = " Replacement ";
t2.replaceRange(s, 3, 3 + s.length());
}
}
class B4L implements ActionListener {
public void actionPerformed(ActionEvent e) {
t2.insert(" Inserted ", 10);
}
}
public void init() {
Container cp = getContentPane();
cp.setLayout(new FlowLayout());
// Create Borders for components:
Border brd = BorderFactory.createMatteBorder(1, 1, 1, 1, Color.BLACK);
t1.setBorder(brd);
t2.setBorder(brd);
sp3.setBorder(brd);
sp4.setBorder(brd);
sp5.setBorder(brd);
sp6.setBorder(brd);
// Initialize listeners and add components:
b1.addActionListener(new B1L());
cp.add(b1);
cp.add(t1);
b2.addActionListener(new B2L());
cp.add(b2);
cp.add(t2);
b3.addActionListener(new B3L());
cp.add(b3);
b4.addActionListener(new B4L());
cp.add(b4);
cp.add(sp3);
cp.add(sp4);
cp.add(sp5);
cp.add(sp6);
}
public static void main(String[] args) {
run(new JScrollPanes(), 300, 725);
}
public static void run(JApplet applet, int width, int height) {
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().add(applet);
frame.setSize(width, height);
applet.init();
applet.start();
frame.setVisible(true);
}
} ///:~
Related examples in the same category