Small Cell Combobox Example
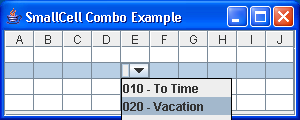
// Example from http://www.crionics.com/products/opensource/faq/swing_ex/SwingExamples.html
/* (swing1.1) */
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.Rectangle;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.util.Vector;
import javax.swing.ComboBoxModel;
import javax.swing.DefaultCellEditor;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.UIManager;
import javax.swing.event.ListDataEvent;
import javax.swing.plaf.basic.BasicComboPopup;
import javax.swing.plaf.basic.ComboPopup;
import javax.swing.plaf.metal.MetalComboBoxUI;
import javax.swing.table.DefaultTableModel;
/**
* @version 1.0 3/06/99
*/
public class SmallCellComboExample extends JFrame {
public SmallCellComboExample() {
super("SmallCell Combo Example");
DefaultTableModel dm = new DefaultTableModel(4, 10) {
public void setValueAt(Object obj, int row, int col) {
if (obj != null) {
String str;
if (obj instanceof String) {
str = ((String) obj).substring(0, 2);
} else {
str = obj.toString();
}
super.setValueAt(str, row, col);
}
}
};
JTable table = new JTable(dm);
String[] str = { "010 - To Time", "020 - Vacation", "030 - Feel Bad" };
SteppedComboBox combo = new SteppedComboBox(str) {
public void contentsChanged(ListDataEvent e) {
selectedItemReminder = null;
super.contentsChanged(e);
}
};
Dimension d = combo.getPreferredSize();
combo.setPopupWidth(d.width);
DefaultCellEditor editor = new DefaultCellEditor(combo);
table.setDefaultEditor(Object.class, editor);
JScrollPane scroll = new JScrollPane(table);
getContentPane().add(scroll, BorderLayout.CENTER);
}
public static void main(String[] args) {
SmallCellComboExample frame = new SmallCellComboExample();
frame.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
frame.setSize(300, 120);
frame.setVisible(true);
}
}
/**
* @version 1.0 12/12/98
*/
class SteppedComboBoxUI extends MetalComboBoxUI {
protected ComboPopup createPopup() {
BasicComboPopup popup = new BasicComboPopup(comboBox) {
public void show() {
Dimension popupSize = ((SteppedComboBox) comboBox)
.getPopupSize();
popupSize
.setSize(popupSize.width,
getPopupHeightForRowCount(comboBox
.getMaximumRowCount()));
Rectangle popupBounds = computePopupBounds(0, comboBox
.getBounds().height, popupSize.width, popupSize.height);
scroller.setMaximumSize(popupBounds.getSize());
scroller.setPreferredSize(popupBounds.getSize());
scroller.setMinimumSize(popupBounds.getSize());
list.invalidate();
int selectedIndex = comboBox.getSelectedIndex();
if (selectedIndex == -1) {
list.clearSelection();
} else {
list.setSelectedIndex(selectedIndex);
}
list.ensureIndexIsVisible(list.getSelectedIndex());
setLightWeightPopupEnabled(comboBox.isLightWeightPopupEnabled());
show(comboBox, popupBounds.x, popupBounds.y);
}
};
popup.getAccessibleContext().setAccessibleParent(comboBox);
return popup;
}
}
class SteppedComboBox extends JComboBox {
protected int popupWidth;
public SteppedComboBox(ComboBoxModel aModel) {
super(aModel);
setUI(new SteppedComboBoxUI());
popupWidth = 0;
}
public SteppedComboBox(final Object[] items) {
super(items);
setUI(new SteppedComboBoxUI());
popupWidth = 0;
}
public SteppedComboBox(Vector items) {
super(items);
setUI(new SteppedComboBoxUI());
popupWidth = 0;
}
public void setPopupWidth(int width) {
popupWidth = width;
}
public Dimension getPopupSize() {
Dimension size = getSize();
if (popupWidth < 1)
popupWidth = size.width;
return new Dimension(popupWidth, size.height);
}
}
Related examples in the same category