Demonstrates the functionality of DatagramConnection framework.
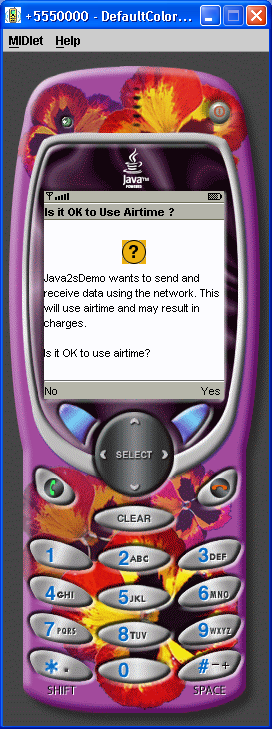
/*** Chapter 5 Sample Code for Datagram functionality ***/
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
import javax.microedition.io.*;
import java.util.*;
public class DatagramTest extends MIDlet {
// Port 9001 is used for datagram communication
static final int receiveport = 9001;
Receive receiveThread = new Receive();
public DatagramTest() {
}
public void startApp() {
// Start the listening thread
receiveThread.start();
// Send message Hello World!
sendMessage("Hello World!");
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
// This function sends a datagram message on port 9001.
void sendMessage(String msg) {
String destAddr = "datagram://localhost:" + receiveport;
DatagramConnection dc = null;
Datagram dgram;
byte[] bMsg = msg.getBytes();
try {
dc = (DatagramConnection)Connector.open(destAddr);
// Create a datagram socket and send
dgram= dc.newDatagram(bMsg,bMsg.length,destAddr);
dc.send(dgram);
System.out.println("Sending Packet:" + msg);
dc.close();
}
catch (Exception e) {
System.out.println("Exception Connecting: " + e.getMessage());
}
finally {
if (dc != null) {
try {
dc.close();
}
catch (Exception e) {
System.out.println("Exception Closing: " + e.getMessage());
}
}
}
}
// This function is a listener. It waits to receive datagram packets on 9001 port
class Receive extends Thread {
public void run() {
doReceive();
}
void doReceive() {
DatagramConnection dc = null;
Datagram dgram;
try {
// Open Server side datagram connection
dc = (DatagramConnection)Connector.open("datagram://:"+receiveport);
String receivedMsg;
while (true) {
dgram = dc.newDatagram(dc.getMaximumLength());
try {
dc.receive(dgram);
} catch (Exception e) {
System.out.println("Exception in receiving message:" + e.getMessage());
}
receivedMsg = new String(dgram.getData(), 0,dgram.getLength());
System.out.println("Received Message: " + receivedMsg);
try {
Thread.sleep(500);
}
catch (Exception e) {
System.out.println("Exception doReceive(): " + e.getMessage());
}
}
}
catch (Exception e) {
System.out.println("Exception doReceive(): " + e.getMessage());
}
finally {
if (dc != null) {
try {
dc.close();
}
catch (Exception e) {
System.out.println("Exception Closing: " + e.getMessage());
}
}
}
}
}
}
Related examples in the same category