Encode and Decode
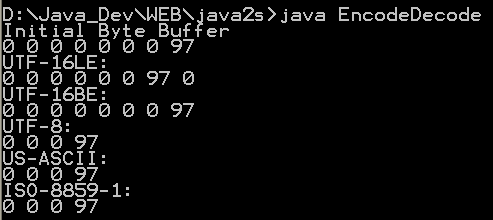
// : c12:EncodeDecode.java
// From 'Thinking in Java, 3rd ed.' (c) Bruce Eckel 2002
// www.BruceEckel.com. See copyright notice in CopyRight.txt.
import java.nio.ByteBuffer;
import java.nio.charset.Charset;
public class EncodeDecode {
public static void print(ByteBuffer bb) {
while (bb.hasRemaining())
System.out.print(bb.get() + " ");
System.out.println();
bb.rewind();
}
public static void main(String[] args) {
ByteBuffer bb = ByteBuffer.wrap(new byte[] { 0, 0, 0, 0, 0, 0, 0,
(byte) 'a' });
System.out.println("Initial Byte Buffer");
print(bb);
Charset[] csets = { Charset.forName("UTF-16LE"),
Charset.forName("UTF-16BE"), Charset.forName("UTF-8"),
Charset.forName("US-ASCII"), Charset.forName("ISO-8859-1") };
for (int i = 0; i < csets.length; i++) {
System.out.println(csets[i].name() + ":");
print(csets[i].encode(bb.asCharBuffer()));
csets[i].decode(bb);
bb.rewind();
}
}
} ///:~
Related examples in the same category