Set drag types (Smart GWT)
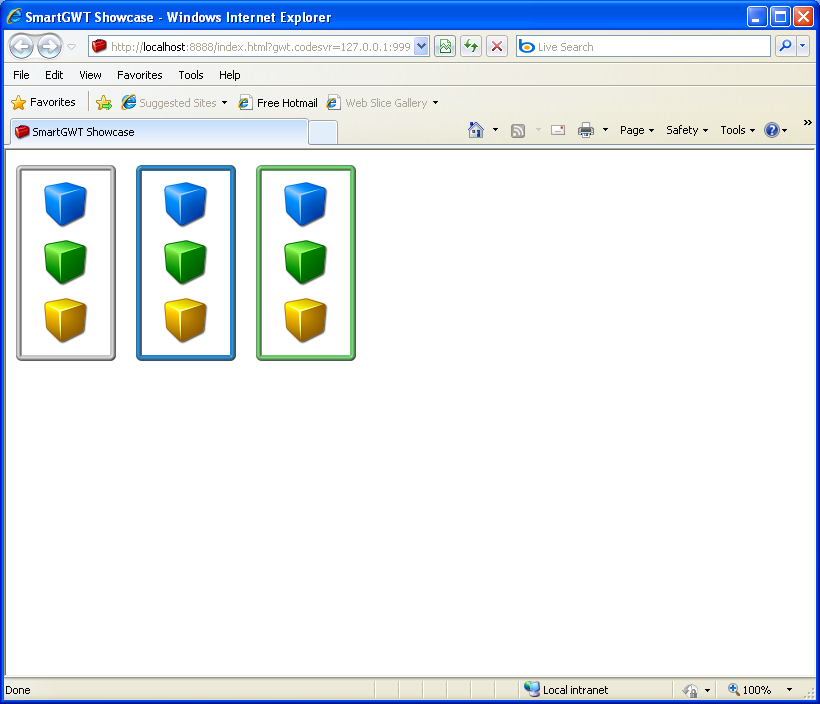
/*
* SmartGWT (GWT for SmartClient)
* Copyright 2008 and beyond, Isomorphic Software, Inc.
*
* SmartGWT is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License version 3
* as published by the Free Software Foundation. SmartGWT is also
* available under typical commercial license terms - see
* http://smartclient.com/license
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package com.smartgwt.sample.showcase.client;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.user.client.ui.RootPanel;
import com.smartgwt.client.types.Alignment;
import com.smartgwt.client.types.DragAppearance;
import com.smartgwt.client.widgets.Canvas;
import com.smartgwt.client.widgets.Img;
import com.smartgwt.client.widgets.layout.HStack;
import com.smartgwt.client.widgets.layout.VStack;
public class Showcase implements EntryPoint {
public void onModuleLoad() {
RootPanel.get().add(getViewPanel());
}
public Canvas getViewPanel() {
DropBox gray = new DropBox("edges/gray/6.png");
gray.addMember(new DragPiece("cube_blue.png", "b"));
gray.addMember(new DragPiece("cube_green.png", "g"));
gray.addMember(new DragPiece("cube_yellow.png", "y"));
gray.setDropTypes("b", "g", "y");
DropBox blue = new DropBox("edges/blue/6.png");
blue.addMember(new DragPiece("cube_blue.png", "b"));
blue.addMember(new DragPiece("cube_green.png", "g"));
blue.addMember(new DragPiece("cube_yellow.png", "y"));
blue.setDropTypes("b");
DropBox green = new DropBox("edges/green/6.png");
green.addMember(new DragPiece("cube_blue.png", "b"));
green.addMember(new DragPiece("cube_green.png", "g"));
green.addMember(new DragPiece("cube_yellow.png", "y"));
green.setDropTypes("g");
HStack hStack = new HStack(20);
hStack.addMember(gray);
hStack.addMember(blue);
hStack.addMember(green);
return hStack;
}
private class DropBox extends VStack {
public DropBox() {
setShowEdges(true);
setMembersMargin(10);
setLayoutMargin(10);
setCanAcceptDrop(true);
setAnimateMembers(true);
setDropLineThickness(4);
setAutoHeight();
}
public DropBox(String edgeImage) {
this();
setEdgeImage(edgeImage);
}
}
private class DragPiece extends Img {
public DragPiece() {
setWidth(48);
setHeight(48);
setLayoutAlign(Alignment.CENTER);
setCanDragReposition(true);
setCanDrop(true);
setDragAppearance(DragAppearance.TARGET);
setAppImgDir("pieces/48/");
}
public DragPiece(String src, String dragType) {
this();
setSrc(src);
setDragType(dragType);
}
}
}
SmartGWT.zip( 9,880 k)Related examples in the same category