Grid Dependent Selects Sample (Smart GWT)
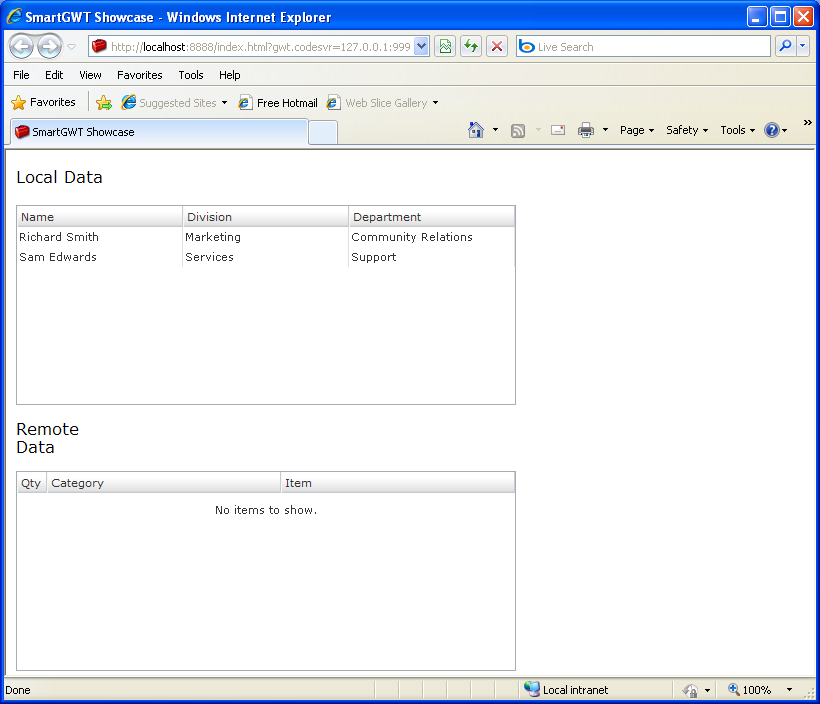
/*
* SmartGWT (GWT for SmartClient)
* Copyright 2008 and beyond, Isomorphic Software, Inc.
*
* SmartGWT is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License version 3
* as published by the Free Software Foundation. SmartGWT is also
* available under typical commercial license terms - see
* http://smartclient.com/license
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package com.smartgwt.sample.showcase.client;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Map;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.user.client.ui.RootPanel;
import com.smartgwt.client.data.Criteria;
import com.smartgwt.client.data.DataSource;
import com.smartgwt.client.data.fields.DataSourceBooleanField;
import com.smartgwt.client.data.fields.DataSourceDateField;
import com.smartgwt.client.data.fields.DataSourceEnumField;
import com.smartgwt.client.data.fields.DataSourceFloatField;
import com.smartgwt.client.data.fields.DataSourceIntegerField;
import com.smartgwt.client.data.fields.DataSourceLinkField;
import com.smartgwt.client.data.fields.DataSourceTextField;
import com.smartgwt.client.types.ListGridFieldType;
import com.smartgwt.client.widgets.Canvas;
import com.smartgwt.client.widgets.IButton;
import com.smartgwt.client.widgets.Label;
import com.smartgwt.client.widgets.events.ClickEvent;
import com.smartgwt.client.widgets.events.ClickHandler;
import com.smartgwt.client.widgets.form.fields.FilterCriteriaFunction;
import com.smartgwt.client.widgets.form.fields.SelectItem;
import com.smartgwt.client.widgets.form.fields.events.ChangedEvent;
import com.smartgwt.client.widgets.form.fields.events.ChangedHandler;
import com.smartgwt.client.widgets.form.validator.FloatPrecisionValidator;
import com.smartgwt.client.widgets.form.validator.FloatRangeValidator;
import com.smartgwt.client.widgets.grid.EditorValueMapFunction;
import com.smartgwt.client.widgets.grid.ListGrid;
import com.smartgwt.client.widgets.grid.ListGridField;
import com.smartgwt.client.widgets.grid.ListGridRecord;
import com.smartgwt.client.widgets.layout.VLayout;
public class Showcase implements EntryPoint {
public void onModuleLoad() {
RootPanel.get().add(getViewPanel());
}
public Canvas getViewPanel() {
VLayout layout = new VLayout(15);
Label localDataLabel = new Label("Local Data");
localDataLabel.setWidth("90%");
localDataLabel.setHeight(25);
localDataLabel.setBaseStyle("exampleSeparator");
layout.addMember(localDataLabel);
final ListGrid localDataGrid = new ListGrid();
localDataGrid.setWidth(500);
localDataGrid.setHeight(200);
localDataGrid.setCanEdit(true);
localDataGrid.setData(EmployeeData.getRecords());
ListGridField employeeField = new ListGridField("employee", "Name");
employeeField.setCanEdit(false);
ListGridField divisionField = new ListGridField("division", "Division");
SelectItem divisionSelectItem = new SelectItem();
divisionSelectItem.setValueMap("Marketing", "Sales", "Services");
divisionSelectItem.addChangedHandler(new ChangedHandler() {
public void onChanged(ChangedEvent event) {
// calling 'setValueMap()' will force the 'getEditorValueMap' method to
// be re-evaluated for the department field
localDataGrid.setValueMap("department", new LinkedHashMap());
}
});
divisionField.setEditorType(divisionSelectItem);
ListGridField departmentField = new ListGridField("department", "Department");
final Map<String, String[]> departments = new HashMap<String, String[]>();
departments.put("Marketing", new String[] { "Advertising", "Community Relations" });
departments.put("Sales", new String[] { "Channed Sales", "Direct Sales" });
departments.put("Manufacturing", new String[] { "Design", "Development", "QA" });
departments.put("Services", new String[] { "Support", "Consulting" });
SelectItem departmentSelectItem = new SelectItem();
departmentSelectItem.setAddUnknownValues(false);
departmentField.setEditorValueMapFunction(new EditorValueMapFunction() {
public Map getEditorValueMap(Map values, ListGridField field, ListGrid grid) {
String division = (String) values.get("division");
String[] divisions = departments.get(division);
// convert divisions into ValueMap. In this case we simply create a Map
// with same key -> value since
// stored value is the same as user displayable value
Map<String, String> valueMap = new HashMap<String, String>();
for (int i = 0; i < divisions.length; i++) {
String val = divisions[i];
valueMap.put(val, val);
}
return valueMap;
}
});
departmentField.setEditorType(departmentSelectItem);
localDataGrid.setFields(employeeField, divisionField, departmentField);
layout.addMember(localDataGrid);
// remote dependent selects sample
Label remoteDataLabel = new Label("Remote Data");
remoteDataLabel.setWidth("90%");
remoteDataLabel.setHeight(25);
remoteDataLabel.setBaseStyle("exampleSeparator");
layout.addMember(remoteDataLabel);
final ListGrid remoteDataGrid = new ListGrid();
remoteDataGrid.setWidth(500);
remoteDataGrid.setHeight(200);
remoteDataGrid.setCanEdit(true);
ListGridField quantityField = new ListGridField("quantity", "Qty");
quantityField.setType(ListGridFieldType.INTEGER);
quantityField.setWidth(30);
ListGridField categoryField = new ListGridField("categoryName", "Category");
DataSource supplyCategoryDS = SupplyCategoryXmlDS.getInstance();
DataSource supplyItemDS = ItemSupplyXmlDS.getInstance();
SelectItem categorySelectItem = new SelectItem();
categorySelectItem.setOptionDataSource(supplyCategoryDS);
categoryField.setEditorType(categorySelectItem);
categoryField.addChangedHandler(new com.smartgwt.client.widgets.grid.events.ChangedHandler() {
public void onChanged(com.smartgwt.client.widgets.grid.events.ChangedEvent event) {
remoteDataGrid.clearEditValue(event.getRowNum(), "itemName");
}
});
ListGridField itemField = new ListGridField("itemName", "Item");
SelectItem itemEditor = new SelectItem();
itemEditor.setPickListFilterCriteriaFunction(new FilterCriteriaFunction() {
public Criteria getCriteria() {
String category = (String) remoteDataGrid.getEditedCell(remoteDataGrid.getEditRow(),
"categoryName");
return new Criteria("category", category);
}
});
itemEditor.setOptionDataSource(supplyItemDS);
itemField.setEditorType(itemEditor);
remoteDataGrid.setFields(quantityField, categoryField, itemField);
layout.addMember(remoteDataGrid);
IButton newOrderButton = new IButton("Order New Item");
newOrderButton.setWidth(110);
newOrderButton.addClickHandler(new ClickHandler() {
public void onClick(ClickEvent event) {
Map defaultValues = new HashMap();
defaultValues.put("quantity", 1);
remoteDataGrid.startEditingNew(defaultValues);
}
});
layout.addMember(newOrderButton);
return layout;
}
}
class EmployeeRecord extends ListGridRecord {
public EmployeeRecord() {
}
public EmployeeRecord(String employee, String division, String department) {
setEmployee(employee);
setDivision(division);
setDepartment(department);
}
public String getEmployee() {
return getAttribute("employee");
}
public void setEmployee(String employee) {
setAttribute("employee", employee);
}
public String getDivision() {
return getAttribute("division");
}
public void setDivision(String division) {
setAttribute("division", division);
}
public String getDepartment() {
return getAttribute("department");
}
public void setDepartment(String department) {
setAttribute("department", department);
}
}
class EmployeeData {
private static EmployeeRecord[] records;
public static EmployeeRecord[] getRecords() {
if (records == null) {
records = getNewRecords();
}
return records;
}
public static EmployeeRecord[] getNewRecords() {
return new EmployeeRecord[] {
new EmployeeRecord("Richard Smith", "Marketing", "Community Relations"),
new EmployeeRecord("Sam Edwards", "Services", "Support") };
}
}
class SupplyCategoryXmlDS extends DataSource {
private static SupplyCategoryXmlDS instance = null;
public static SupplyCategoryXmlDS getInstance() {
if (instance == null) {
instance = new SupplyCategoryXmlDS("supplyCategoryDS");
}
return instance;
}
public SupplyCategoryXmlDS(String id) {
setID(id);
setRecordXPath("/List/supplyCategory");
DataSourceTextField itemNameField = new DataSourceTextField("categoryName", "Item", 128, true);
itemNameField.setPrimaryKey(true);
DataSourceTextField parentField = new DataSourceTextField("parentID", null);
parentField.setHidden(true);
parentField.setRequired(true);
parentField.setRootValue("root");
parentField.setForeignKey("supplyCategoryDS.categoryName");
setFields(itemNameField, parentField);
setDataURL("ds/test_data/supplyCategory.data.xml");
setClientOnly(true);
}
}
class ItemSupplyXmlDS extends DataSource {
private static ItemSupplyXmlDS instance = null;
public static ItemSupplyXmlDS getInstance() {
if (instance == null) {
instance = new ItemSupplyXmlDS("supplyItemDS");
}
return instance;
}
public ItemSupplyXmlDS(String id) {
setID(id);
setRecordXPath("/List/supplyItem");
DataSourceIntegerField pkField = new DataSourceIntegerField("itemID");
pkField.setHidden(true);
pkField.setPrimaryKey(true);
DataSourceTextField itemNameField = new DataSourceTextField("itemName", "Item Name", 128, true);
DataSourceTextField skuField = new DataSourceTextField("SKU", "SKU", 10, true);
DataSourceTextField descriptionField = new DataSourceTextField("description", "Description",
2000);
DataSourceTextField categoryField = new DataSourceTextField("category", "Category", 128, true);
categoryField.setForeignKey("supplyCategoryDS.categoryName");
DataSourceEnumField unitsField = new DataSourceEnumField("units", "Units", 5);
unitsField.setValueMap("Roll", "Ea", "Pkt", "Set", "Tube", "Pad", "Ream", "Tin", "Bag", "Ctn",
"Box");
DataSourceFloatField unitCostField = new DataSourceFloatField("unitCost", "Unit Cost", 5);
FloatRangeValidator rangeValidator = new FloatRangeValidator();
rangeValidator.setMin(0);
rangeValidator.setErrorMessage("Please enter a valid (positive) cost");
FloatPrecisionValidator precisionValidator = new FloatPrecisionValidator();
precisionValidator.setPrecision(2);
precisionValidator.setErrorMessage("The maximum allowed precision is 2");
unitCostField.setValidators(rangeValidator, precisionValidator);
DataSourceBooleanField inStockField = new DataSourceBooleanField("inStock", "In Stock");
DataSourceDateField nextShipmentField = new DataSourceDateField("nextShipment", "Next Shipment");
setFields(pkField, itemNameField, skuField, descriptionField, categoryField, unitsField,
unitCostField, inStockField, nextShipmentField);
setDataURL("ds/test_data/supplyItem.data.xml");
setClientOnly(true);
}
}
class CountryXmlDS extends DataSource {
private static CountryXmlDS instance = null;
public static CountryXmlDS getInstance() {
if (instance == null) {
instance = new CountryXmlDS("countryDS");
}
return instance;
}
public CountryXmlDS(String id) {
setID(id);
setRecordXPath("/List/country");
DataSourceIntegerField pkField = new DataSourceIntegerField("pk");
pkField.setHidden(true);
pkField.setPrimaryKey(true);
DataSourceTextField countryCodeField = new DataSourceTextField("countryCode", "Code");
countryCodeField.setRequired(true);
DataSourceTextField countryNameField = new DataSourceTextField("countryName", "Country");
countryNameField.setRequired(true);
DataSourceTextField capitalField = new DataSourceTextField("capital", "Capital");
DataSourceTextField governmentField = new DataSourceTextField("government", "Government", 500);
DataSourceBooleanField memberG8Field = new DataSourceBooleanField("member_g8", "G8");
DataSourceTextField continentField = new DataSourceTextField("continent", "Continent");
continentField.setValueMap("Europe", "Asia", "North America", "Australia/Oceania",
"South America", "Africa");
DataSourceDateField independenceField = new DataSourceDateField("independence", "Nationhood");
DataSourceFloatField areaField = new DataSourceFloatField("area", "Area (km&sup2;)");
DataSourceIntegerField populationField = new DataSourceIntegerField("population", "Population");
DataSourceFloatField gdpField = new DataSourceFloatField("gdp", "GDP ($M)");
DataSourceLinkField articleField = new DataSourceLinkField("article", "Info");
setFields(pkField, countryCodeField, countryNameField, capitalField, governmentField,
memberG8Field, continentField, independenceField, areaField, populationField, gdpField,
articleField);
setDataURL("ds/test_data/country.data.xml");
setClientOnly(true);
}
}
SmartGWT.zip( 9,880 k)Related examples in the same category