Auto-complete ComboBox (Ext GWT)
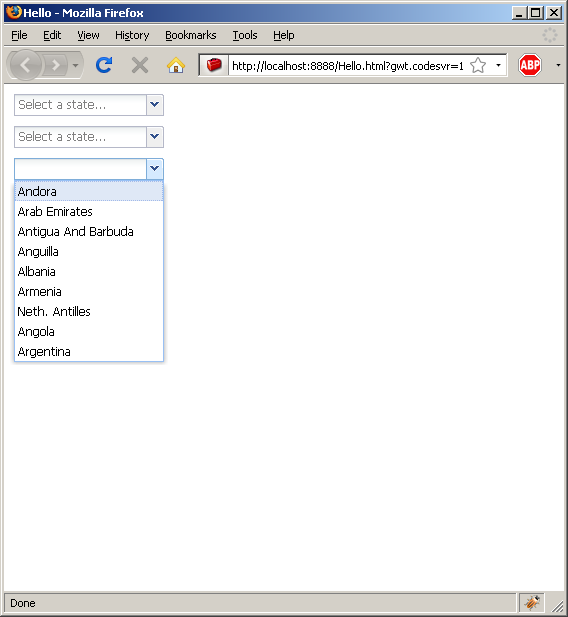
/*
* Ext GWT - Ext for GWT
* Copyright(c) 2007-2009, Ext JS, LLC.
* licensing@extjs.com
*
* http://extjs.com/license
*/
package com.google.gwt.sample.hello.client;
import java.util.ArrayList;
import java.util.List;
import com.extjs.gxt.ui.client.data.BaseModelData;
import com.extjs.gxt.ui.client.store.ListStore;
import com.extjs.gxt.ui.client.widget.LayoutContainer;
import com.extjs.gxt.ui.client.widget.VerticalPanel;
import com.extjs.gxt.ui.client.widget.form.ComboBox;
import com.extjs.gxt.ui.client.widget.form.ComboBox.TriggerAction;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.user.client.Element;
import com.google.gwt.user.client.Random;
import com.google.gwt.user.client.ui.RootPanel;
public class Hello implements EntryPoint {
public void onModuleLoad() {
RootPanel.get().add(new ComboBoxExample());
}
}
class ComboBoxExample extends LayoutContainer {
@Override
protected void onRender(Element parent, int index) {
super.onRender(parent, index);
VerticalPanel vp = new VerticalPanel();
vp.setSpacing(10);
ListStore<State> states = new ListStore<State>();
states.add(getStates());
ComboBox<State> combo = new ComboBox<State>();
combo.setEmptyText("Select a state...");
combo.setDisplayField("name");
combo.setWidth(150);
combo.setStore(states);
combo.setTypeAhead(true);
combo.setTriggerAction(TriggerAction.ALL);
vp.add(combo);
states = new ListStore<State>();
states.add(getStates());
combo = new ComboBox<State>();
combo.setEmptyText("Select a state...");
combo.setDisplayField("name");
combo.setTemplate(getTemplate());
combo.setWidth(150);
combo.setStore(states);
combo.setTypeAhead(true);
combo.setTriggerAction(TriggerAction.ALL);
vp.add(combo);
ListStore<Country> countries = new ListStore<Country>();
countries.add(getCountries());
ComboBox<Country> combo2 = new ComboBox<Country>();
combo2.setWidth(150);
combo2.setStore(countries);
//combo2.setTemplate(getFlagTemplate(Examples.isExplorer() ? "" : "../../"));
combo2.setDisplayField("name");
combo2.setTypeAhead(true);
combo2.setTriggerAction(TriggerAction.ALL);
vp.add(combo2);
add(vp);
}
public static List<State> getStates() {
List<State> states = new ArrayList<State>();
states.add(new State("AL", "Alabama", "The Heart of Dixie"));
states.add(new State("AK", "Alaska", "The Land of the Midnight Sun"));
states.add(new State("AZ", "Arizona", "The Grand Canyon State"));
states.add(new State("AR", "Arkansas", "The Natural State"));
states.add(new State("CA", "California", "The Golden State"));
states.add(new State("CO", "Colorado", "The Mountain State"));
states.add(new State("CT", "Connecticut", "The Constitution State"));
states.add(new State("DE", "Delaware", "The First State"));
states.add(new State("DC", "District of Columbia", "The Nations Capital"));
states.add(new State("FL", "Florida", "The Sunshine State"));
states.add(new State("GA", "Georgia", "The Peach State"));
states.add(new State("HI", "Hawaii", "The Aloha State"));
states.add(new State("ID", "Idaho", "Famous Potatoes"));
states.add(new State("IL", "Illinois", "The Prairie State"));
states.add(new State("IN", "Indiana", "The Hospitality State"));
states.add(new State("IA", "Iowa", "The Corn State"));
states.add(new State("KS", "Kansas", "The Sunflower State"));
states.add(new State("KY", "Kentucky", "The Bluegrass State"));
states.add(new State("LA", "Louisiana", "The Bayou State"));
states.add(new State("ME", "Maine", "The Pine Tree State"));
states.add(new State("MD", "Maryland", "Chesapeake State"));
states.add(new State("MA", "Massachusetts", "The Spirit of America"));
states.add(new State("MI", "Michigan", "Great Lakes State"));
states.add(new State("MN", "Minnesota", "North Star State"));
states.add(new State("MS", "Mississippi", "Magnolia State"));
states.add(new State("MO", "Missouri", "Show Me State"));
states.add(new State("MT", "Montana", "Big Sky Country"));
states.add(new State("NE", "Nebraska", "Beef State"));
states.add(new State("NV", "Nevada", "Silver State"));
states.add(new State("NH", "New Hampshire", "Granite State"));
states.add(new State("NJ", "New Jersey", "Garden State"));
states.add(new State("NM", "New Mexico", "Land of Enchantment"));
states.add(new State("NY", "New York", "Empire State"));
states.add(new State("NC", "North Carolina", "First in Freedom"));
states.add(new State("ND", "North Dakota", "Peace Garden State"));
states.add(new State("OH", "Ohio", "The Heart of it All"));
states.add(new State("OK", "Oklahoma", "Oklahoma is OK"));
states.add(new State("OR", "Oregon", "Pacific Wonderland"));
states.add(new State("PA", "Pennsylvania", "Keystone State"));
states.add(new State("RI", "Rhode Island", "Ocean State"));
states.add(new State("SC", "South Carolina", "Nothing Could be Finer"));
states.add(new State("SD", "South Dakota", "Great Faces, Great Places"));
states.add(new State("TN", "Tennessee", "Volunteer State"));
states.add(new State("TX", "Texas", "Lone Star State"));
states.add(new State("UT", "Utah", "Salt Lake State"));
states.add(new State("VT", "Vermont", "Green Mountain State"));
states.add(new State("VA", "Virginia", "Mother of States"));
states.add(new State("WA", "Washington", "Green Tree State"));
states.add(new State("WV", "West Virginia", "Mountain State"));
states.add(new State("WI", "Wisconsin", "Americas Dairyland"));
states.add(new State("WY", "Wyoming", "Like No Place on Earth"));
return states;
}
public static List<Country> getCountries() {
List<Country> countries = new ArrayList<Country>();
countries.add(new Country("ad", "Andora", 100 + (Random.nextInt(110) * 100)));
countries.add(new Country("ae", "Arab Emirates", 100 + (Random.nextInt(110) * 100)));
countries.add(new Country("ag", "Antigua And Barbuda",
100 + (Random.nextInt(110) * 100)));
countries.add(new Country("ai", "Anguilla", 100 + (Random.nextInt(110) * 100)));
countries.add(new Country("al", "Albania", 100 + (Random.nextInt(110) * 100)));
countries.add(new Country("am", "Armenia", 100 + (Random.nextInt(110) * 100)));
countries.add(new Country("an", "Neth. Antilles", 100 + (Random.nextInt(110) * 100)));
countries.add(new Country("ao", "Angola", 100 + (Random.nextInt(110) * 100)));
countries.add(new Country("ar", "Argentina", 100 + (Random.nextInt(110) * 100)));
return countries;
}
private native String getTemplate() /*-{
return [
'<tpl for=".">',
'<div class="x-combo-list-item" qtip="{slogan}" qtitle="State Slogan">{name}</div>',
'</tpl>'
].join("");
}-*/;
private native String getFlagTemplate(String base) /*-{
return [
'<tpl for=".">',
'<div class="x-combo-list-item"><img width="16px" height="11px" src="' + base + 'samples/images/icons/fam/flags/{[values.abbr]}.png"> {[values.name]}</div>',
'</tpl>'
].join("");
}-*/;
}
class Country extends BaseModelData {
public Country() {
}
public Country(String abbr, String name, int value) {
setAbbr(abbr);
setName(name);
set("value", value);
}
public String getName() {
return get("name");
}
public void setName(String name) {
set("name", name);
}
public String getAbbr() {
return get("abbr");
}
public void setAbbr(String abbr) {
set("abbr", abbr);
}
}
class State extends BaseModelData {
public State() {
}
public State(String abbr, String name, String slogan) {
setAbbr(abbr);
setName(name);
setSlogan(slogan);
}
public String getSlogan() {
return get("slogan");
}
public void setSlogan(String slogan) {
set("slogan", slogan);
}
public String getAbbr() {
return get("abbr");
}
public void setAbbr(String abbr) {
set("abbr", abbr);
}
public String getName() {
return get("name");
}
public void setName(String name) {
set("name", name);
}
}
Ext-GWT.zip( 4,297 k)Related examples in the same category