EventListenerList enabled Secret Label
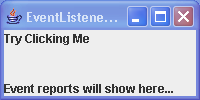
/*
Java Swing, 2nd Edition
By Marc Loy, Robert Eckstein, Dave Wood, James Elliott, Brian Cole
ISBN: 0-596-00408-7
Publisher: O'Reilly
*/
// SecretTest.java
//A demonstration framework for the EventListenerList-enabled SecretLabel class
//
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import javax.swing.JFrame;
import javax.swing.JLabel;
public class SecretTest extends JFrame {
public SecretTest() {
super("EventListenerList Demo");
setSize(200, 100);
setDefaultCloseOperation(EXIT_ON_CLOSE);
SecretLabel secret = new SecretLabel("Try Clicking Me");
final JLabel reporter = new JLabel("Event reports will show here...");
secret.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent ae) {
reporter.setText("Got it: " + ae.getActionCommand());
}
});
getContentPane().add(secret, BorderLayout.NORTH);
getContentPane().add(reporter, BorderLayout.SOUTH);
}
public static void main(String args[]) {
SecretTest st = new SecretTest();
st.setVisible(true);
}
}
//SecretLabel.java
//An extension of the JLabel class that listens to mouse clicks and converts
//them to ActionEvents, which in turn are reported via an EventListenersList
//object
//
class SecretLabel extends JLabel {
public SecretLabel(String msg) {
super(msg);
addMouseListener(new MouseAdapter() {
public void mouseClicked(MouseEvent me) {
fireActionPerformed(new ActionEvent(SecretLabel.this,
ActionEvent.ACTION_PERFORMED, "SecretMessage"));
}
});
}
public void addActionListener(ActionListener l) {
// We'll just use the listenerList we inherit from JComponent.
listenerList.add(ActionListener.class, l);
}
public void removeActionListener(ActionListener l) {
listenerList.remove(ActionListener.class, l);
}
protected void fireActionPerformed(ActionEvent ae) {
Object[] listeners = listenerList.getListeners(ActionListener.class);
for (int i = 0; i < listeners.length; i++) {
((ActionListener) listeners[i]).actionPerformed(ae);
}
}
}
Related examples in the same category