Use printf() to create a time stamp.
import java.io.FileWriter;
import java.io.PrintWriter;
import java.util.Calendar;
public class Main {
static void timeStamp(String msg, PrintWriter pw) {
Calendar cal = Calendar.getInstance();
pw.printf("%s %tc\n", msg, cal);
}
public static void main(String args[]) throws Exception {
PrintWriter pw = new PrintWriter(new FileWriter("logfile.txt", true));
timeStamp("File opened", pw);
pw.close();
if (pw.checkError())
System.out.println("I/O error occurred.");
}
}
Related examples in the same category
1. | give a known Locale (with the right decimal separator) to bypass the current Locale | | |
2. | Two decimal digits | | |
3. | Group separators | | |
4. | Align string output | | |
5. | Align float numbers | | |
6. | Display current time | | |
7. | Display short and long date and time formats. | | |
8. | "%d %(d %+d %05d | | |
9. | System.out.printf("a %1$s is a %1$s is a %1$s...", "AAA") | | |
10. | System.out.printf( "%-10.10s %s\n", word, word.length() ) | | |
11. | System.out.printf("num is %s\n", 5) | | |
12. | System.out.printf("The DATE is %tz\n", new Date() ) | | |
13. | System.out.printf("%1$s://%2$s/%3$s\n", "http", "host", "path") | | |
14. | System.out.printf("num is %.2f\n", Math.PI ) | | |
15. | System.out.printf("String is '%-5s'\n", "A") | | |
16. | System.out.printf(Locale.CHINA, "The date is %tc\n", new Date()) | | |
17. | System.out.printf("The DATE is %tr\n", new Date() ) | | |
18. | System.out.printf("The DATE is %Tc\n", new Date() ) | | |
19. | System.out.printf("%s://%s/%s\n", "http", "host", "path") | | |
20. | System.out.printf("num is %03d\n", 5 ) | | |
21. | System.out.printf("num is {%07.3f}\n", 3.14 ) | | |
22. | System.out.printf("1.0 4f num is %4f\n", 1.0 ) | | |
23. | System.out.printf("sci not num is %e\n", 3000000.0 ) | | |
24. | System.out.printf("boolean value is %1$b, %1$B\n", true ) | | |
25. | System.out.printf("hex value is %1$#x, %1$#X\n", 0xCAFE ) | | |
26. | System.out.printf( Locale.ITALIAN, "value: %f\n", 3.14 ) | | |
27. | System.out.printf("float is %-20f!\n", 1.23456789) | | |
28. | Use printf(): Two decimal digits | | |
29. | Use printf(): group separators | | |
30. | Use printf(): %10s %10s %10s | | |
31. | Use printf(): align float numbers | | |
32. | Default floating-point format: %f | | |
33. | Floating-point with commas: %,f | | |
34. | Negative floating-point default: %,f | | |
35. | Negative floating-point option: %,(f | | |
36. | Line up positive and negative values | | |
37. | System.out.format("%f, %1$+020.10f %n", Math.PI) | | |
38. | System.out.printf("%b %n %c %n %s %n %s %n %d %n",..) | | |
39. | Basic Math with System.out.printf | | 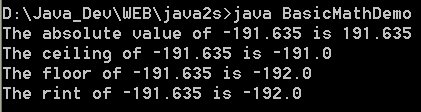 |
40. | Display a file in hex. | | |