SimpleAttributeSet Example
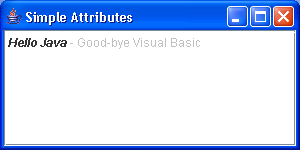
/*
Definitive Guide to Swing for Java 2, Second Edition
By John Zukowski
ISBN: 1-893115-78-X
Publisher: APress
*/
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Container;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTextPane;
import javax.swing.text.BadLocationException;
import javax.swing.text.DefaultStyledDocument;
import javax.swing.text.SimpleAttributeSet;
import javax.swing.text.StyleConstants;
import javax.swing.text.StyledDocument;
public class SimpleAttributeSample {
public static void main(String args[]) {
JFrame frame = new JFrame("Simple Attributes");
Container content = frame.getContentPane();
StyledDocument document = new DefaultStyledDocument();
SimpleAttributeSet attributes = new SimpleAttributeSet();
attributes.addAttribute(StyleConstants.CharacterConstants.Bold,
Boolean.TRUE);
attributes.addAttribute(StyleConstants.CharacterConstants.Italic,
Boolean.TRUE);
// Insert content
try {
document.insertString(document.getLength(), "Hello Java",
attributes);
} catch (BadLocationException badLocationException) {
System.err.println("Oops");
}
attributes = new SimpleAttributeSet();
attributes.addAttribute(StyleConstants.CharacterConstants.Bold,
Boolean.FALSE);
attributes.addAttribute(StyleConstants.CharacterConstants.Italic,
Boolean.FALSE);
attributes.addAttribute(StyleConstants.CharacterConstants.Foreground,
Color.lightGray);
// Insert content
try {
document.insertString(document.getLength(),
" - Good-bye Visual Basic", attributes);
} catch (BadLocationException badLocationException) {
System.err.println("Oops");
}
JTextPane textPane = new JTextPane(document);
textPane.setEditable(false);
JScrollPane scrollPane = new JScrollPane(textPane);
content.add(scrollPane, BorderLayout.CENTER);
frame.setSize(300, 150);
frame.setVisible(true);
}
}
Related examples in the same category