A frame that restores position and size from a properties file and updates the properties upon exit
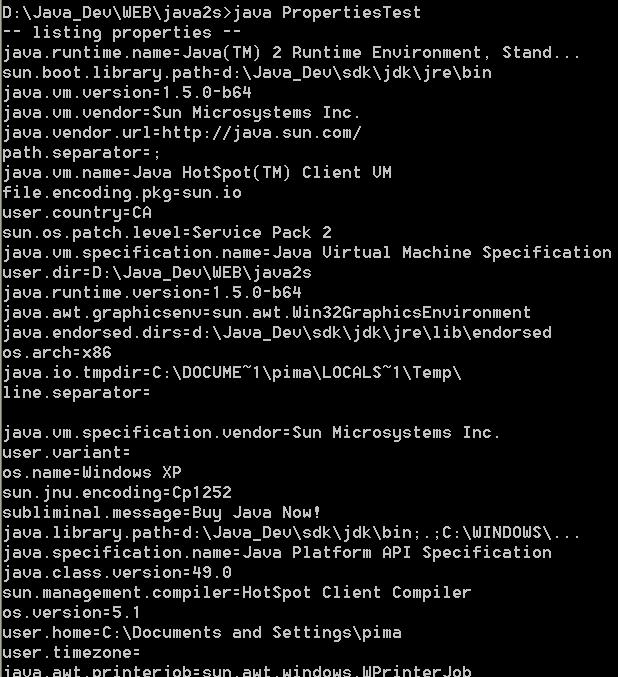
/*
This program is a part of the companion code for Core Java 8th ed.
(http://horstmann.com/corejava)
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
import java.awt.EventQueue;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.Properties;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
/**
* A program to test properties. The program remembers the frame position, size, and title.
* @version 1.00 2007-04-29
* @author Cay Horstmann
*/
public class PropertiesTest
{
public static void main(String[] args)
{
EventQueue.invokeLater(new Runnable()
{
public void run()
{
PropertiesFrame frame = new PropertiesFrame();
frame.setVisible(true);
}
});
}
}
/**
* A frame that restores position and size from a properties file and updates the properties upon
* exit.
*/
class PropertiesFrame extends JFrame
{
public PropertiesFrame()
{
// get position, size, title from properties
String userDir = System.getProperty("user.home");
File propertiesDir = new File(userDir, ".corejava");
if (!propertiesDir.exists()) propertiesDir.mkdir();
propertiesFile = new File(propertiesDir, "program.properties");
Properties defaultSettings = new Properties();
defaultSettings.put("left", "0");
defaultSettings.put("top", "0");
defaultSettings.put("width", "" + DEFAULT_WIDTH);
defaultSettings.put("height", "" + DEFAULT_HEIGHT);
defaultSettings.put("title", "");
settings = new Properties(defaultSettings);
if (propertiesFile.exists()) try
{
FileInputStream in = new FileInputStream(propertiesFile);
settings.load(in);
}
catch (IOException ex)
{
ex.printStackTrace();
}
int left = Integer.parseInt(settings.getProperty("left"));
int top = Integer.parseInt(settings.getProperty("top"));
int width = Integer.parseInt(settings.getProperty("width"));
int height = Integer.parseInt(settings.getProperty("height"));
setBounds(left, top, width, height);
// if no title given, ask user
String title = settings.getProperty("title");
if (title.equals("")) title = JOptionPane.showInputDialog("Please supply a frame title:");
if (title == null) title = "";
setTitle(title);
addWindowListener(new WindowAdapter()
{
public void windowClosing(WindowEvent event)
{
settings.put("left", "" + getX());
settings.put("top", "" + getY());
settings.put("width", "" + getWidth());
settings.put("height", "" + getHeight());
settings.put("title", getTitle());
try
{
FileOutputStream out = new FileOutputStream(propertiesFile);
settings.store(out, "Program Properties");
}
catch (IOException ex)
{
ex.printStackTrace();
}
System.exit(0);
}
});
}
private File propertiesFile;
private Properties settings;
public static final int DEFAULT_WIDTH = 300;
public static final int DEFAULT_HEIGHT = 200;
}
Related examples in the same category