Finds the first index within a String, handling null.
/**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
* <p>Operations on {@link java.lang.String} that are
* <code>null</code> safe.</p>
*
* @see java.lang.String
* @author <a href="http://jakarta.apache.org/turbine/">Apache Jakarta Turbine</a>
* @author <a href="mailto:jon@latchkey.com">Jon S. Stevens</a>
* @author Daniel L. Rall
* @author <a href="mailto:gcoladonato@yahoo.com">Greg Coladonato</a>
* @author <a href="mailto:ed@apache.org">Ed Korthof</a>
* @author <a href="mailto:rand_mcneely@yahoo.com">Rand McNeely</a>
* @author Stephen Colebourne
* @author <a href="mailto:fredrik@westermarck.com">Fredrik Westermarck</a>
* @author Holger Krauth
* @author <a href="mailto:alex@purpletech.com">Alexander Day Chaffee</a>
* @author <a href="mailto:hps@intermeta.de">Henning P. Schmiedehausen</a>
* @author Arun Mammen Thomas
* @author Gary Gregory
* @author Phil Steitz
* @author Al Chou
* @author Michael Davey
* @author Reuben Sivan
* @author Chris Hyzer
* @author Scott Johnson
* @since 1.0
* @version $Id: StringUtils.java 635447 2008-03-10 06:27:09Z bayard $
*/
public class Main {
/**
* <p>Finds the first index within a String, handling <code>null</code>.
* This method uses {@link String#indexOf(String, int)}.</p>
*
* <p>A <code>null</code> String will return <code>-1</code>.
* A negative start position is treated as zero.
* An empty ("") search String always matches.
* A start position greater than the string length only matches
* an empty search String.</p>
*
* <pre>
* StringUtils.indexOf(null, *, *) = -1
* StringUtils.indexOf(*, null, *) = -1
* StringUtils.indexOf("", "", 0) = 0
* StringUtils.indexOf("aabaabaa", "a", 0) = 0
* StringUtils.indexOf("aabaabaa", "b", 0) = 2
* StringUtils.indexOf("aabaabaa", "ab", 0) = 1
* StringUtils.indexOf("aabaabaa", "b", 3) = 5
* StringUtils.indexOf("aabaabaa", "b", 9) = -1
* StringUtils.indexOf("aabaabaa", "b", -1) = 2
* StringUtils.indexOf("aabaabaa", "", 2) = 2
* StringUtils.indexOf("abc", "", 9) = 3
* </pre>
*
* @param str the String to check, may be null
* @param searchStr the String to find, may be null
* @param startPos the start position, negative treated as zero
* @return the first index of the search String,
* -1 if no match or <code>null</code> string input
* @since 2.0
*/
public static int indexOf(String str, String searchStr, int startPos) {
if (str == null || searchStr == null) {
return -1;
}
// JDK1.2/JDK1.3 have a bug, when startPos > str.length for "", hence
if (searchStr.length() == 0 && startPos >= str.length()) {
return str.length();
}
return str.indexOf(searchStr, startPos);
}
}
Related examples in the same category
1. | String Region Match Demo | |  |
2. | Palindrome | | 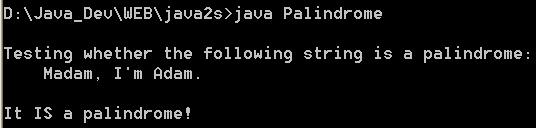 |
3. | Look for particular sequences in sentences | | 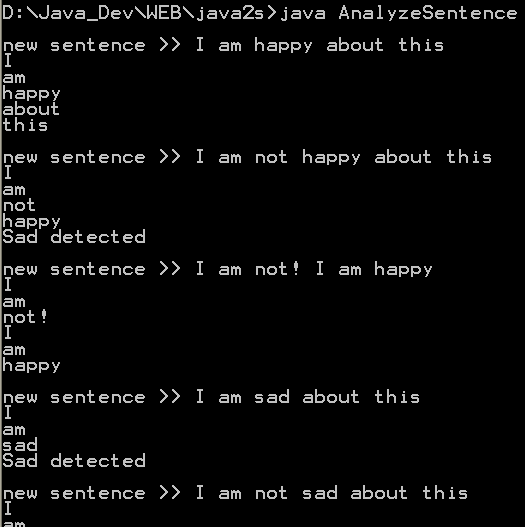 |
4. | Strings -- extract printable strings from binary file | | |
5. | Java Search String | | |
6. | Java String endsWith | | |
7. | Java String startsWith | | |
8. | Search a substring Anywhere | | |
9. | Starts with, ignore case( regular expressions ) | | |
10. | Ends with, ignore case( regular expressions ) | | |
11. | Anywhere, ignore case( regular expressions ) | | |
12. | Searching a String for a Character or a Substring | | |
13. | Not found returns -1 | | |
14. | If a string contains a specific word | | |
15. | Not found | | |
16. | if a String starts with a digit or uppercase letter | | |
17. | Search a String to find the first index of any character in the given set of characters. | | |
18. | Search a String to find the first index of any character not in the given set of characters. | | |
19. | Searches a String for substrings delimited by a start and end tag, returning all matching substrings in an array. | | |
20. | Helper functions to query a strings end portion. The comparison is case insensitive. | | |
21. | Helper functions to query a strings start portion. The comparison is case insensitive. | | |
22. | Wrapper for arrays of ordered strings. This verifies the arrays and supports efficient lookups. | | |
23. | Returns an index into arra (or -1) where the character is not in the charset byte array. | | |
24. | Returns an int[] array of length segments containing the distribution count of the elements in unsorted int[] array with values between min and max (range). | | |
25. | Returns the next index of a character from the chars string | | |
26. | Finds the last index within a String from a start position, handling null. | | |
27. | Finds the n-th index within a String, handling null. | | |
28. | Case insensitive check if a String ends with a specified suffix. | | |
29. | Case insensitive check if a String starts with a specified prefix. | | |
30. | Case insensitive removal of a substring if it is at the begining of a source string, otherwise returns the source string. | | |
31. | Case insensitive removal of a substring if it is at the end of a source string, otherwise returns the source string. | | |
32. | Check if a String ends with a specified suffix. | | |
33. | Check if a String starts with a specified prefix. | | |
34. | Determine if a String is contained in a String Collection | | |
35. | Determine if a String is contained in a String Collection, ignoring case | | |
36. | Determine if a String is contained in a String [], ignoring case | | |
37. | Determine if a String is contained in a String [], ignoring case or not as specified | | |
38. | Determine if a String is contained in a String[] | | |
39. | Determines if the specified string contains only Unicode letters or digits as defined by Character#isLetterOrDigit(char) | | |
40. | Determining the validity of various XML names | | |
41. | Return the nth index of the given token occurring in the given string | | |
42. | Find the earliest index of any of a set of potential substrings. | | |
43. | Find the latest index of any of a set of potential substrings. | | |
44. | Fast String Search | | |
45. | Performs continuous matching of a pattern in a given string. | | |
46. | Count match | | |