This builds a red sphere using the Sphere utility class and adds lights
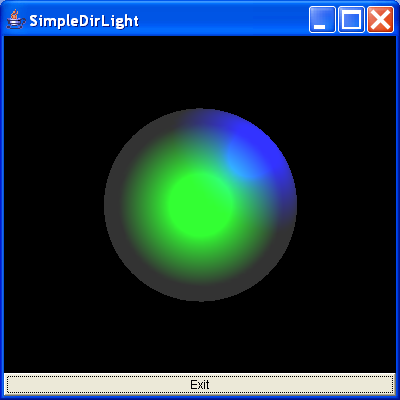
/*
Essential Java 3D Fast
Ian Palmer
Publisher: Springer-Verlag
ISBN: 1-85233-394-4
*/
import java.awt.BorderLayout;
import java.awt.Button;
import java.awt.Frame;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.media.j3d.AmbientLight;
import javax.media.j3d.Appearance;
import javax.media.j3d.BoundingSphere;
import javax.media.j3d.BranchGroup;
import javax.media.j3d.Canvas3D;
import javax.media.j3d.Locale;
import javax.media.j3d.Material;
import javax.media.j3d.PhysicalBody;
import javax.media.j3d.PhysicalEnvironment;
import javax.media.j3d.SpotLight;
import javax.media.j3d.Transform3D;
import javax.media.j3d.TransformGroup;
import javax.media.j3d.View;
import javax.media.j3d.ViewPlatform;
import javax.media.j3d.VirtualUniverse;
import javax.vecmath.AxisAngle4d;
import javax.vecmath.Color3f;
import javax.vecmath.Point3d;
import javax.vecmath.Point3f;
import javax.vecmath.Vector3f;
import com.sun.j3d.utils.geometry.Sphere;
/**
* This builds a red sphere using the Sphere utility class and adds lights so
* that you can see it shape. It creates a material for the sphere, creates an
* ambient light and a two spot lights of different colours.
*
* @author I.J.Palmer
* @version 1.0
*/
public class SimpleSpotLights extends Frame implements ActionListener {
protected Canvas3D myCanvas3D = new Canvas3D(null);
protected Button myButton = new Button("Exit");
/**
* This function builds the view branch of the scene graph. It creates a
* branch group and then creates the necessary view elements to give a
* useful view of our content.
*
* @param c
* Canvas3D that will display the view
* @return BranchGroup that is the root of the view elements
*/
protected BranchGroup buildViewBranch(Canvas3D c) {
BranchGroup viewBranch = new BranchGroup();
Transform3D viewXfm = new Transform3D();
viewXfm.set(new Vector3f(0.0f, 0.0f, 10.0f));
TransformGroup viewXfmGroup = new TransformGroup(viewXfm);
ViewPlatform myViewPlatform = new ViewPlatform();
PhysicalBody myBody = new PhysicalBody();
PhysicalEnvironment myEnvironment = new PhysicalEnvironment();
viewXfmGroup.addChild(myViewPlatform);
viewBranch.addChild(viewXfmGroup);
View myView = new View();
myView.addCanvas3D(c);
myView.attachViewPlatform(myViewPlatform);
myView.setPhysicalBody(myBody);
myView.setPhysicalEnvironment(myEnvironment);
return viewBranch;
}
/**
* This creates some lights and adds them to the BranchGroup.
*
* @param b
* BranchGroup that the lights are added to.
*/
protected void addLights(BranchGroup b) {
// Create a bounds for the lights
BoundingSphere bounds = new BoundingSphere(new Point3d(0.0, 0.0, 0.0),
100.0);
//Set up the ambient light
Color3f ambientColour = new Color3f(0.2f, 0.2f, 0.2f);
AmbientLight ambientLight = new AmbientLight(ambientColour);
ambientLight.setInfluencingBounds(bounds);
Color3f blueColour = new Color3f(0.0f, 0.0f, 1.0f);
Point3f bluePosition = new Point3f(1.8f, 1.8f, 1.8f);
Point3f blueAtten = new Point3f(1.0f, 0.0f, 0.0f);
Vector3f blueDir = new Vector3f(-1.0f, -1.0f, -1.0f);
SpotLight blueLight = new SpotLight(blueColour, bluePosition,
blueAtten, blueDir, (float) (Math.PI / 2.0), 0.0f);
blueLight.setInfluencingBounds(bounds);
Color3f greenColour = new Color3f(0.0f, 1.0f, 0.0f);
Point3f greenPosition = new Point3f(0.0f, 0.0f, 3.0f);
Point3f greenAtten = new Point3f(1.0f, 0.0f, 0.0f);
Vector3f greenDir = new Vector3f(0.0f, 0.0f, -1.0f);
SpotLight greenLight = new SpotLight(greenColour, greenPosition,
greenAtten, greenDir, (float) (Math.PI / 2.0), 0.0f);
greenLight.setInfluencingBounds(bounds);
//Add the lights to the BranchGroup
b.addChild(ambientLight);
b.addChild(greenLight);
b.addChild(blueLight);
}
/**
* This build the content branch of our scene graph. It creates a transform
* group so that the shape is slightly tilted to reveal its 3D shape.
*
* @param shape
* Node that represents the geometry for the content
* @return BranchGroup that is the root of the content branch
*/
protected BranchGroup buildContentBranch() {
BranchGroup contentBranch = new BranchGroup();
Transform3D rotateCube = new Transform3D();
rotateCube.set(new AxisAngle4d(1.0, 1.0, 0.0, Math.PI / 4.0));
TransformGroup rotationGroup = new TransformGroup(rotateCube);
contentBranch.addChild(rotationGroup);
//Create a new appearance
Appearance app = new Appearance();
//Create the colours for the material
Color3f ambientColour = new Color3f(1.0f, 1.0f, 1.0f);
Color3f diffuseColour = new Color3f(1.0f, 1.0f, 1.0f);
Color3f specularColour = new Color3f(1.0f, 1.0f, 1.0f);
Color3f emissiveColour = new Color3f(0.0f, 0.0f, 0.0f);
//Define the shininess
float shininess = 20.0f;
//Set the material of the appearance
app.setMaterial(new Material(ambientColour, emissiveColour,
diffuseColour, specularColour, shininess));
//Create and add a new sphere using the appearance
rotationGroup.addChild(new Sphere(2.0f, Sphere.GENERATE_NORMALS, 120,
app));
//Use the addLights function to add the lights to the branch
addLights(contentBranch);
//Return the root of the content branch
return contentBranch;
}
/**
* Handles the exit button action to quit the program.
*/
public void actionPerformed(ActionEvent e) {
dispose();
System.exit(0);
}
/**
* This creates a default universe and locale, creates a window and uses the
* functions defined in this class to build the view and content branches of
* the scene graph.
*/
public SimpleSpotLights() {
VirtualUniverse myUniverse = new VirtualUniverse();
Locale myLocale = new Locale(myUniverse);
myLocale.addBranchGraph(buildViewBranch(myCanvas3D));
myLocale.addBranchGraph(buildContentBranch());
setTitle("SimpleDirLight");
setSize(400, 400);
setLayout(new BorderLayout());
add("Center", myCanvas3D);
add("South", myButton);
myButton.addActionListener(this);
setVisible(true);
}
public static void main(String[] args) {
SimpleSpotLights spl = new SimpleSpotLights();
}
}
Related examples in the same category