Overload the -- relative to MyClass class using a friend.
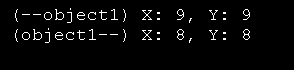
#include <iostream>
using namespace std;
class MyClass {
int x, y;
public:
MyClass() {
x=0;
y=0;
}
MyClass(int i, int j) {
x=i;
y=j;
}
void getXY(int &i, int &j) {
i=x;
j=y;
}
friend MyClass operator--(MyClass &ob); // prefix
friend MyClass operator--(MyClass &ob, int notused); // postfix
};
// Overload -- (prefix) for MyClass class using a friend.
MyClass operator--(MyClass &ob)
{
ob.x--;
ob.y--;
return ob;
}
// Overload -- (postfix) for MyClass class using a friend.
MyClass operator--(MyClass &ob, int notused)
{
ob.x--;
ob.y--;
return ob;
}
int main()
{
MyClass object1(10, 10);
int x, y;
--object1; // decrement object1 an object
object1.getXY(x, y);
cout << "(--object1) X: " << x << ", Y: " << y << endl;
object1--; // decrement object1 an object
object1.getXY(x, y);
cout << "(object1--) X: " << x << ", Y: " << y << endl;
return 0;
}
Related examples in the same category