Namespace Demo: define a namespace
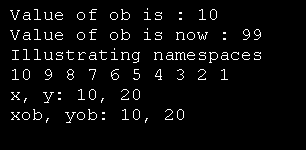
#include <iostream>
using namespace std;
namespace MyNameSpace {
class demo {
int i;
public:
demo(int x) {
i = x;
}
void seti(int x) {
i = x;
}
int geti() {
return i;
}
};
char str[] = "Illustrating namespaces\n";
int counter;
}
namespace SecondNamespace {
int x, y;
}
int main()
{
MyNameSpace::demo ob(10); // use scope resolution
cout << "Value of ob is : " << ob.geti();
cout << endl;
ob.seti(99);
cout << "Value of ob is now : " << ob.geti();
cout << endl;
using MyNameSpace::str; // bring str into current scope
cout << str;
using namespace MyNameSpace; // bring all of MyNameSpace into current scope
for(counter = 10; counter; counter--)
cout << counter << " ";
cout << endl;
SecondNamespace::x = 10; // use SecondNamespace namespace
SecondNamespace::y = 20;
cout << "x, y: " << SecondNamespace:: x;
cout << ", " << SecondNamespace::y << endl;
using namespace SecondNamespace; // bring another namespace into view
demo xob(x), yob(y);
cout << "xob, yob: " << xob.geti() << ", ";
cout << yob.geti() << endl;
return 0;
}
Related examples in the same category