Use lock to synchronize access to an object
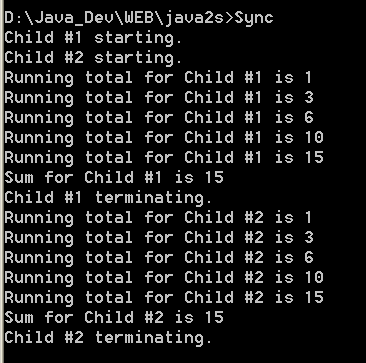
/*
C#: The Complete Reference
by Herbert Schildt
Publisher: Osborne/McGraw-Hill (March 8, 2002)
ISBN: 0072134852
*/
// Use lock to synchronize access to an object.
using System;
using System.Threading;
class SumArray {
int sum;
public int sumIt(int[] nums) {
lock(this) { // lock the entire method
sum = 0; // reset sum
for(int i=0; i < nums.Length; i++) {
sum += nums[i];
Console.WriteLine("Running total for " +
Thread.CurrentThread.Name +
" is " + sum);
Thread.Sleep(10); // allow task-switch
}
return sum;
}
}
}
class MyThread {
public Thread thrd;
int[] a;
int answer;
/* Create one SumArray object for all
instances of MyThread. */
static SumArray sa = new SumArray();
// Construct a new thread.
public MyThread(string name, int[] nums) {
a = nums;
thrd = new Thread(new ThreadStart(this.run));
thrd.Name = name;
thrd.Start(); // start the thread
}
// Begin execution of new thread.
void run() {
Console.WriteLine(thrd.Name + " starting.");
answer = sa.sumIt(a);
Console.WriteLine("Sum for " + thrd.Name +
" is " + answer);
Console.WriteLine(thrd.Name + " terminating.");
}
}
public class Sync {
public static void Main() {
int[] a = {1, 2, 3, 4, 5};
MyThread mt1 = new MyThread("Child #1", a);
MyThread mt2 = new MyThread("Child #2", a);
mt1.thrd.Join();
mt2.thrd.Join();
}
}
Related examples in the same category