Directory Services Change Password
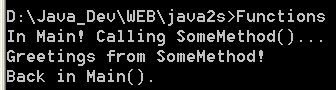
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.DirectoryServices;
using System.DirectoryServices.ActiveDirectory;
using System.Runtime.InteropServices;
namespace Homelink.AD.Utility
{
public class Functions
{
public static void ChangePassword(string userID, string oldPassword, string newPassword)
{
DirectoryEntry userEntry = Functions.GetUserEntry(userID);
if (userEntry != null)
{
try
{
// Check old password
// string userName = userEntry.Properties["CN"][0].ToString();
// DirectoryEntry en = new DirectoryEntry(userEntry.Path, userName, oldPassword, AuthenticationTypes.Secure);
// string cn = en.Properties["CN"][0].ToString();
userEntry.UsePropertyCache = true;
userEntry.Invoke("ChangePassword", new object[] { oldPassword, newPassword });
// userEntry.Invoke("SetPassword", new object[] { oldPassword });
userEntry.CommitChanges();
userEntry.Close();
}
catch (Exception e)
{
Exception baseException = e.GetBaseException();
if (baseException is COMException)
{
COMException comException = baseException as COMException;
switch (comException.ErrorCode)
{
case -2147024810:
throw new Exception("The current password does not match our records. Please try again.");
case -2147022651:
throw new Exception("indicating password does not meet security requirements of the domain.");
case -2147023570:
throw new Exception("invalidate user or password.");
case -2147016657:
throw new Exception("invalidate user or password. Please try again. ");
default:
throw e;
}
}
}
}
else
{
throw new Exception("UserID = '" + userID + "' not found");
}
}
public static DirectoryEntry GetUserEntry(string userID)
{
DirectoryEntry entry = Environment.GetDirectoryEntry();
string filter = "(&(objectClass=user)(Pager=" + userID + "))";
DirectorySearcher search = new DirectorySearcher(entry, filter);
search.SearchScope = SearchScope.Subtree;
SearchResult result = search.FindOne();
if (result != null)
{
DirectoryEntry userEntry = new DirectoryEntry(result.Path, Environment.AD_AdminAccountName, Environment.AD_AdminPassword, AuthenticationTypes.Secure);
return userEntry;
}
else
{
return null;
}
}
}
}
Related examples in the same category