Regular expressions: Match
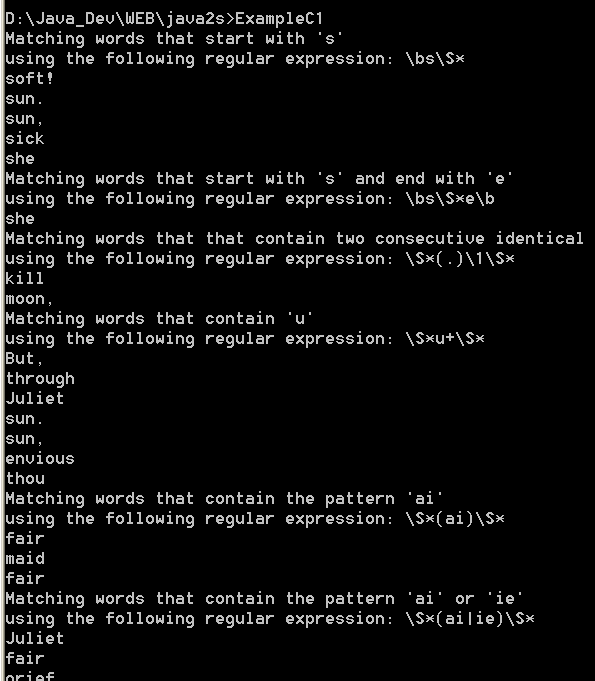
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
ExampleC_1.cs illustrates the use of regular expressions
*/
using System;
using System.Text.RegularExpressions;
public class ExampleC_1 {
private static void DisplayMatches(string text,
string regularExpressionString ) {
Console.WriteLine("using the following regular expression: " +
regularExpressionString);
// create a MatchCollection object to store the words that
// match the regular expression
MatchCollection myMatchCollection =
Regex.Matches(text, regularExpressionString);
// use a foreach loop to iterate over the Match objects in
// the MatchCollection object
foreach (Match myMatch in myMatchCollection)
{
Console.WriteLine(myMatch);
}
}
public static void Main()
{
string text =
"But, soft! what light through yonder window breaks?\n" +
"It is the east, and Juliet is the sun.\n" +
"Arise, fair sun, and kill the envious moon,\n" +
"Who is already sick and pale with grief,\n" +
"That thou her maid art far more fair than she";
// match words that start with 's'
Console.WriteLine("Matching words that start with 's'");
DisplayMatches(text, @"\bs\S*");
// match words that start with 's' and end with 'e'
Console.WriteLine("Matching words that start with 's' and end with 'e'");
DisplayMatches(text, @"\bs\S*e\b");
// match words that contain two consecutive identical characters
Console.WriteLine("Matching words that that contain two consecutive identical characters");
DisplayMatches(text, @"\S*(.)\1\S*");
// match words that contain 'u'
Console.WriteLine("Matching words that contain 'u'");
DisplayMatches(text, @"\S*u+\S*");
// match words that contain the pattern 'ai'
Console.WriteLine("Matching words that contain the pattern 'ai'");
DisplayMatches(text, @"\S*(ai)\S*");
// match words that contain the pattern 'ai' or 'ie'
Console.WriteLine("Matching words that contain the pattern 'ai' or 'ie'");
DisplayMatches(text, @"\S*(ai|ie)\S*");
// match words that contain 'k' or 'f'
Console.WriteLine("Matching words that contain 'k' or 'f'");
DisplayMatches(text, @"\S*[kf]\S*");
// match words that contain any letters in the range 'b' through 'd'
Console.WriteLine("Matching words that contain any letters in the range 'b' through 'd'");
DisplayMatches(text, @"\S*[b-d]\S*");
}
}
Related examples in the same category