1. | Use Uri | | 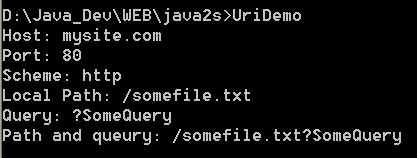 |
2. | Build the hash table of HTML entity references and encode Url | | |
3. | Is Relative Url | | |
4. | Is Rooted Url | | |
5. | Correctly encode a name for a URL. | | |
6. | Essentially creates a query string. | | |
7. | Uri Class Provides an object representation of a uniform resource identifier (URI) and easy access to the parts of the URI. | | |
8. | Initializes a new instance of the Uri class with the specified URI. | | |
9. | Initializes a new instance of the Uri class based on the specified base URI and relative URI string. | | |
10. | Gets the absolute path of the URI. | | |
11. | Gets the absolute URI. | | |
12. | Gets the Domain Name System (DNS) host name or IP address and the port number for a server. | | |
13. | Determines whether the specified host name is a valid DNS name. | | |
14. | Determines whether the specified scheme name is valid. | | |
15. | Gets an unescaped host name that is safe to use for DNS resolution. | | |
16. | Gets the escaped URI fragment. | | |
17. | Gets the decimal value of a hexadecimal digit. | | |
18. | Gets the hash code for the URI. | | |
19. | Gets the specified portion of a Uri instance. | | |
20. | Converts a specified character into its hexadecimal equivalent. | | |
21. | Gets the host component of this instance. | | |
22. | Gets the type of the host name specified in the URI. | | |
23. | Gets whether the port value of the URI is the default for this scheme. | | |
24. | Gets a value indicating whether the specified Uri is a file URI. | | |
25. | Determines whether a specified character is a valid hexadecimal digit. | | |
26. | Gets whether the specified Uri references the local host. | | |
27. | Gets whether the specified Uri is a universal naming convention (UNC) path. | | |
28. | Gets a local operating-system representation of a file name. | | |
29. | Determines the difference between two Uri instances. | | |
30. | Gets the original URI string that was passed to the Uri constructor. | | |
31. | Gets the AbsolutePath and Query properties separated by a question mark (?). | | |
32. | Gets the port number of this URI. | | |
33. | Gets any query information included in the specified URI. | | |
34. | Gets the scheme name for this URI. | | |
35. | Gets an array containing the path segments that make up the specified URI. | | |
36. | Gets a canonical string representation for the specified Uri instance. | | |
37. | Specifies that the URI is a pointer to a file. | | |
38. | Specifies that the URI is accessed through the File Transfer Protocol (FTP). | | |
39. | Specifies that the URI is accessed through the Gopher protocol. | | |
40. | Specifies that the URI is accessed through the Hypertext Transfer Protocol (HTTP). | | |
41. | Specifies that the URI is accessed through the Secure Hypertext Transfer Protocol (HTTPS). | | |
42. | Specifies that the URI is an e-mail address and is accessed through the Simple Mail Transport Protocol (SMTP). | | |
43. | Specifies that the URI is an Internet news group and is accessed through the Network News Transport Protocol (NNTP). | | |
44. | Uri.UriSchemeNntp | | |
45. | Indicates that the URI string was completely escaped before the Uri instance was created. | | |
46. | Gets the user name, password, or other user-specific information associated with the specified URI. | | |
47. | Download text data from the specified URI | | |
48. | Expand Uri | | |
49. | Expand Relative Uri | | |
50. | Parse the query string in the URI into a KeyValuePair | | |
51. | Assembles a series of key=value pairs as a URI-escaped query-string. | | |
52. | Get Absolute Url For Local File | | |
53. | Get Post ID From URL | | |
54. | Combine URL | | |
55. | Url Encode | | |
56. | Url encoding (2) | | |
57. | Url Encode 2 | | |
58. | Url Encode 3 | | |
59. | Retrieves the subdomain from the specified URL. | | |
60. | Try to parse the url, similar to int.TryParse | | |
61. | Is Link Valid | | |