Several catch branches
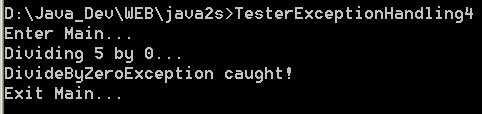
/*
Learning C#
by Jesse Liberty
Publisher: O'Reilly
ISBN: 0596003765
*/
using System;
namespace ExceptionHandling
{
public class TesterExceptionHandling4
{
public void Run()
{
try
{
double a = 5;
double b = 0;
Console.WriteLine("Dividing {0} by {1}...",a,b);
Console.WriteLine ("{0} / {1} = {2}",
a, b, DoDivide(a,b));
}
// most derived exception type first
catch (System.DivideByZeroException)
{
Console.WriteLine(
"DivideByZeroException caught!");
}
catch (System.ArithmeticException)
{
Console.WriteLine(
"ArithmeticException caught!");
}
// generic exception type last
catch
{
Console.WriteLine(
"Unknown exception caught");
}
}
// do the division if legal
public double DoDivide(double a, double b)
{
if (b == 0)
throw new System.DivideByZeroException();
if (a == 0)
throw new System.ArithmeticException();
return a/b;
}
static void Main()
{
Console.WriteLine("Enter Main...");
TesterExceptionHandling4 t = new TesterExceptionHandling4();
t.Run();
Console.WriteLine("Exit Main...");
}
}
}
Related examples in the same category