A class that displays the binary representation of a value
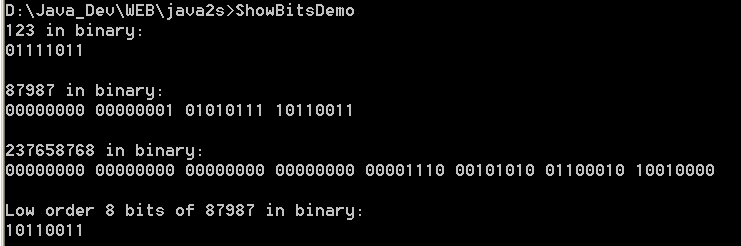
/*
C# A Beginner's Guide
By Schildt
Publisher: Osborne McGraw-Hill
ISBN: 0072133295
*/
/*
Project 5-3
A class that displays the binary representation of a value.
*/
using System;
class ShowBits {
public int numbits;
public ShowBits(int n) {
numbits = n;
}
public void show(ulong val) {
ulong mask = 1;
// left-shift a 1 into the proper position
mask <<= numbits-1;
int spacer = 0;
for(; mask != 0; mask >>= 1) {
if((val & mask) != 0) Console.Write("1");
else Console.Write("0");
spacer++;
if((spacer % 8) == 0) {
Console.Write(" ");
spacer = 0;
}
}
Console.WriteLine();
}
}
// Demonstrate ShowBits.
public class ShowBitsDemo {
public static void Main() {
ShowBits b = new ShowBits(8);
ShowBits i = new ShowBits(32);
ShowBits li = new ShowBits(64);
Console.WriteLine("123 in binary: ");
b.show(123);
Console.WriteLine("\n87987 in binary: ");
i.show(87987);
Console.WriteLine("\n237658768 in binary: ");
li.show(237658768);
// you can also show low-order bits of any integer
Console.WriteLine("\nLow order 8 bits of 87987 in binary: ");
b.show(87987);
}
}
Related examples in the same category