Transparent Forms: holes
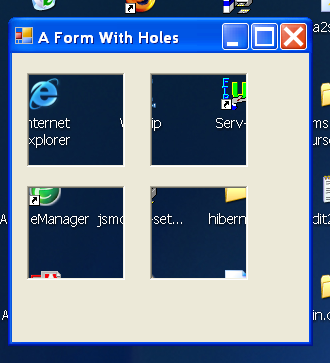
/*
User Interfaces in C#: Windows Forms and Custom Controls
by Matthew MacDonald
Publisher: Apress
ISBN: 1590590457
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace TransparentForms
{
/// <summary>
/// Summary description for Form1.
/// </summary>
public class Holes : System.Windows.Forms.Form
{
internal System.Windows.Forms.PictureBox PictureBox4;
internal System.Windows.Forms.PictureBox PictureBox2;
internal System.Windows.Forms.PictureBox PictureBox1;
internal System.Windows.Forms.PictureBox PictureBox3;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public Holes()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.PictureBox4 = new System.Windows.Forms.PictureBox();
this.PictureBox2 = new System.Windows.Forms.PictureBox();
this.PictureBox1 = new System.Windows.Forms.PictureBox();
this.PictureBox3 = new System.Windows.Forms.PictureBox();
this.SuspendLayout();
//
// PictureBox4
//
this.PictureBox4.BackColor = System.Drawing.Color.FromArgb(((System.Byte)(255)), ((System.Byte)(128)), ((System.Byte)(128)));
this.PictureBox4.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D;
this.PictureBox4.Location = new System.Drawing.Point(108, 108);
this.PictureBox4.Name = "PictureBox4";
this.PictureBox4.Size = new System.Drawing.Size(76, 76);
this.PictureBox4.TabIndex = 10;
this.PictureBox4.TabStop = false;
//
// PictureBox2
//
this.PictureBox2.BackColor = System.Drawing.Color.FromArgb(((System.Byte)(255)), ((System.Byte)(128)), ((System.Byte)(128)));
this.PictureBox2.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D;
this.PictureBox2.Location = new System.Drawing.Point(12, 108);
this.PictureBox2.Name = "PictureBox2";
this.PictureBox2.Size = new System.Drawing.Size(76, 76);
this.PictureBox2.TabIndex = 9;
this.PictureBox2.TabStop = false;
//
// PictureBox1
//
this.PictureBox1.BackColor = System.Drawing.Color.FromArgb(((System.Byte)(255)), ((System.Byte)(128)), ((System.Byte)(128)));
this.PictureBox1.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D;
this.PictureBox1.Location = new System.Drawing.Point(108, 16);
this.PictureBox1.Name = "PictureBox1";
this.PictureBox1.Size = new System.Drawing.Size(76, 76);
this.PictureBox1.TabIndex = 8;
this.PictureBox1.TabStop = false;
//
// PictureBox3
//
this.PictureBox3.BackColor = System.Drawing.Color.FromArgb(((System.Byte)(255)), ((System.Byte)(128)), ((System.Byte)(128)));
this.PictureBox3.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D;
this.PictureBox3.Location = new System.Drawing.Point(12, 16);
this.PictureBox3.Name = "PictureBox3";
this.PictureBox3.Size = new System.Drawing.Size(76, 76);
this.PictureBox3.TabIndex = 7;
this.PictureBox3.TabStop = false;
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(232, 234);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.PictureBox4,
this.PictureBox2,
this.PictureBox1,
this.PictureBox3});
this.Name = "Form1";
this.Text = "A Form With Holes";
this.TransparencyKey = System.Drawing.Color.FromArgb(((System.Byte)(255)), ((System.Byte)(128)), ((System.Byte)(128)));
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Holes());
}
}
}
Related examples in the same category