Popup Menu action
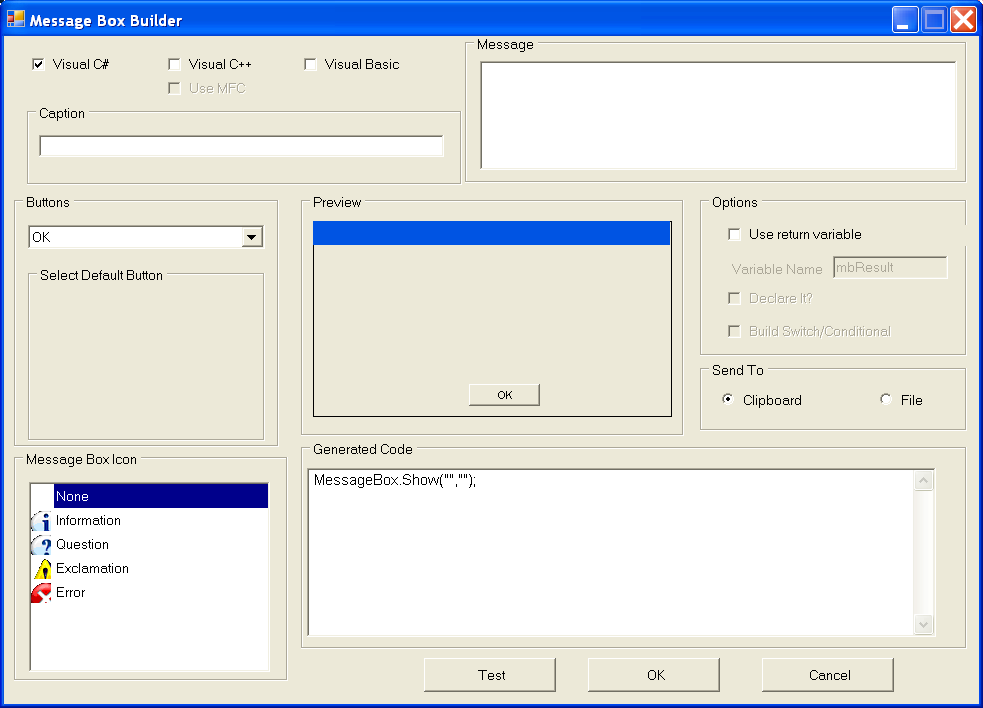
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class MainForm : System.Windows.Forms.Form
{
private int currFontSize = 20;
private ContextMenu popUpMenu;
private MenuItem currentCheckedItem;
private MenuItem checkedHuge;
private MenuItem checkedNormal;
private MenuItem checkedTiny;
public MainForm()
{
InitializeComponent();
Text = "PopUp Menu";
CenterToScreen();
popUpMenu = new ContextMenu();
popUpMenu.MenuItems.Add("Huge", new EventHandler(PopUp_Clicked));
popUpMenu.MenuItems.Add("Normal", new EventHandler(PopUp_Clicked));
popUpMenu.MenuItems.Add("Tiny", new EventHandler(PopUp_Clicked));
this.ContextMenu = popUpMenu;
checkedHuge = this.ContextMenu.MenuItems[0];
checkedNormal = this.ContextMenu.MenuItems[1];
checkedTiny = this.ContextMenu.MenuItems[2];
currentCheckedItem = checkedNormal;
currentCheckedItem.Checked = true;
this.Resize += new System.EventHandler(this.MainForm_Resize);
this.Paint += new System.Windows.Forms.PaintEventHandler(this.MainForm_Paint);
}
private void InitializeComponent()
{
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 273);
this.Text = "Form1";
}
static void Main()
{
Application.Run(new MainForm());
}
private void PopUp_Clicked(object sender, EventArgs e)
{
currentCheckedItem.Checked = false;
MenuItem miClicked = (MenuItem)sender;
string item = miClicked.Text;
if(item == "Huge")
{
currFontSize = 30;
currentCheckedItem = checkedHuge;
}
if(item == "Normal")
{
currFontSize = 20;
currentCheckedItem = checkedNormal;
}
if(item == "Tiny")
{
currFontSize = 8;
currentCheckedItem = checkedTiny;
}
currentCheckedItem.Checked = true;
Invalidate();
}
private void MainForm_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
Graphics g = e.Graphics;
g.DrawString("Right click your mouse",
new Font("Times New Roman", (float)currFontSize),
new SolidBrush(Color.Black),
this.DisplayRectangle);
}
private void MainForm_Resize(object sender, System.EventArgs e)
{
Invalidate();
}
}
Related examples in the same category