Owner Drawn Menu Control
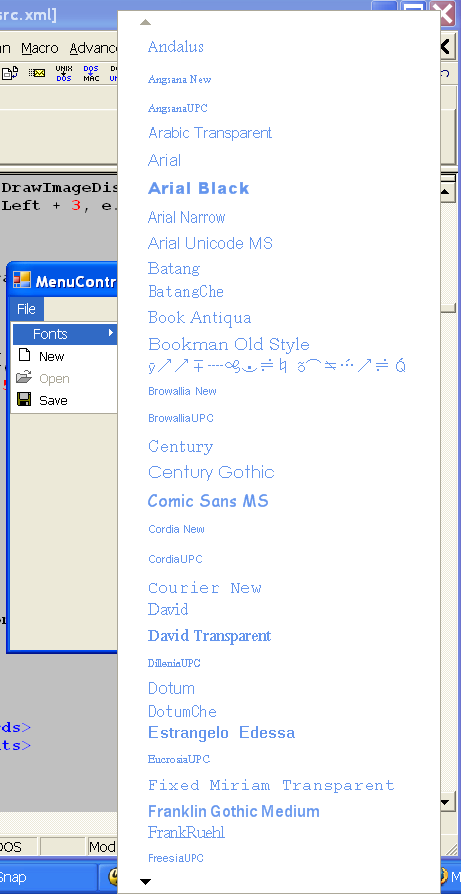
/*
User Interfaces in C#: Windows Forms and Custom Controls
by Matthew MacDonald
Publisher: Apress
ISBN: 1590590457
*/
using System.Drawing;
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Drawing.Text;
namespace OwnerDrawnMenuControl
{
/// <summary>
/// Summary description for OwnerDrawnMenuControl.
/// </summary>
public class OwnerDrawnMenuControl : System.Windows.Forms.Form
{
internal System.Windows.Forms.MainMenu mainMenu1;
internal System.Windows.Forms.MenuItem mnuFile;
internal System.Windows.Forms.MenuItem mnuFonts;
internal System.Windows.Forms.ImageList imgMenu;
private System.ComponentModel.IContainer components;
public OwnerDrawnMenuControl()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
System.Resources.ResourceManager resources = new System.Resources.ResourceManager(typeof(OwnerDrawnMenuControl));
this.mainMenu1 = new System.Windows.Forms.MainMenu();
this.mnuFile = new System.Windows.Forms.MenuItem();
this.mnuFonts = new System.Windows.Forms.MenuItem();
this.imgMenu = new System.Windows.Forms.ImageList(this.components);
//
// mainMenu1
//
this.mainMenu1.MenuItems.AddRange(new System.Windows.Forms.MenuItem[] {
this.mnuFile});
//
// mnuFile
//
this.mnuFile.Index = 0;
this.mnuFile.MenuItems.AddRange(new System.Windows.Forms.MenuItem[] {
this.mnuFonts});
this.mnuFile.Text = "File";
//
// mnuFonts
//
this.mnuFonts.Index = 0;
this.mnuFonts.Text = "Fonts";
//
// imgMenu
//
this.imgMenu.ColorDepth = System.Windows.Forms.ColorDepth.Depth8Bit;
this.imgMenu.ImageSize = new System.Drawing.Size(16, 16);
this.imgMenu.ImageStream = ((System.Windows.Forms.ImageListStreamer)(resources.GetObject("imgMenu.ImageStream")));
this.imgMenu.TransparentColor = System.Drawing.Color.Transparent;
//
// OwnerDrawnMenuControl
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 266);
this.Menu = this.mainMenu1;
this.Name = "OwnerDrawnMenuControl";
this.Text = "MenuControlClient";
this.Load += new System.EventHandler(this.OwnerDrawnMenuControl_Load);
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new OwnerDrawnMenuControl());
}
private void OwnerDrawnMenuControl_Load(object sender, System.EventArgs e)
{
mnuFile.MenuItems.Add(new ImageMenuItem("New", imgMenu.Images[0]));
mnuFile.MenuItems.Add(new ImageMenuItem("Open", imgMenu.Images[1]));
mnuFile.MenuItems.Add(new ImageMenuItem("Save", imgMenu.Images[2]));
InstalledFontCollection fonts = new InstalledFontCollection();
foreach (FontFamily family in fonts.Families)
{
try
{
mnuFonts.MenuItems.Add(new ImageMenuItem(family.Name,
new Font(family, 10), null, Color.CornflowerBlue));
}
catch
{
// Catch invalid fonts/styles and ignore them.
}
}
}
}
public class ImageMenuItem : MenuItem
{
private Font font;
private Color foreColor;
private Image image;
public Font Font
{
get
{
return font;
}
set
{
font = value;
}
}
public Image Image
{
get
{
return image;
}
set
{
image = value;
}
}
public Color ForeColor
{
get
{
return foreColor;
}
set
{
foreColor = value;
}
}
public ImageMenuItem(string text, Font font, Image image, Color foreColor) : base(text)
{
this.Font = font;
this.Image = image;
this.ForeColor = foreColor;
this.OwnerDraw = true;
}
public ImageMenuItem(string text, Image image) : base(text)
{
// Choose a suitable default color and font.
this.Font = new Font("Tahoma", 8);
this.Image = image;
this.ForeColor = SystemColors.MenuText;
this.OwnerDraw = true;
}
protected override void OnMeasureItem(System.Windows.Forms.MeasureItemEventArgs e)
{
base.OnMeasureItem(e);
// Measure size needed to display text.
e.ItemHeight = (int)e.Graphics.MeasureString(this.Text, this.Font).Height + 5;
e.ItemWidth = (int)e.Graphics.MeasureString(this.Text, this.Font).Width + 30;
}
protected override void OnDrawItem(System.Windows.Forms.DrawItemEventArgs e)
{
base.OnDrawItem(e);
// Determine whether disabled text is needed.
Color textColor;
if (this.Enabled == false)
{
textColor = SystemColors.GrayText;
}
else
{
e.DrawBackground();
if ((e.State & DrawItemState.Selected) == DrawItemState.Selected)
{
textColor = SystemColors.HighlightText;
}
else
{
textColor = this.ForeColor;
}
}
// Draw the image.
if (Image != null)
{
if (this.Enabled == false)
{
ControlPaint.DrawImageDisabled(e.Graphics, Image,
e.Bounds.Left + 3, e.Bounds.Top + 2, SystemColors.Menu);
}
else
{
e.Graphics.DrawImage(Image, e.Bounds.Left + 3, e.Bounds.Top + 2);
}
}
// Draw the text with the supplied colors and in the set region.
e.Graphics.DrawString(this.Text, this.Font, new SolidBrush(textColor),
e.Bounds.Left + 25, e.Bounds.Top + 3);
}
}
}
OwnerDrawnMenuControl.zip( 35 k)Related examples in the same category