File.ReadLines Method
using System;
using System.IO;
using System.Linq;
class Program
{
static void Main(string[] args)
{
var files = from file in Directory.EnumerateFiles(@"c:\","*.txt", SearchOption.AllDirectories)
from line in File.ReadLines(file)
where line.Contains("a")
select new
{
File = file,
Line = line
};
foreach (var f in files)
{
Console.WriteLine("{0}\t{1}", f.File, f.Line);
}
Console.WriteLine("{0} files found.",
files.Count().ToString());
}
}
Related examples in the same category
1. | Get file Creation Time | | 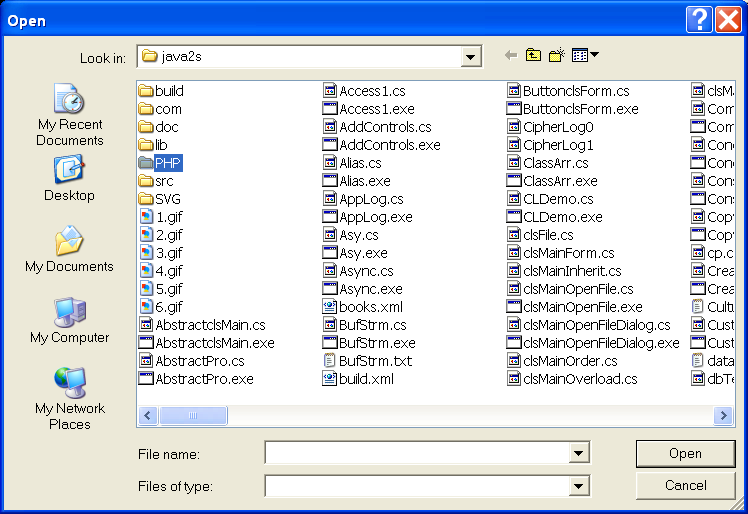 |
2. | Delete a file | | |
3. | Get File Access Control | | |
4. | illustrates the FileAttributes enumeration | | 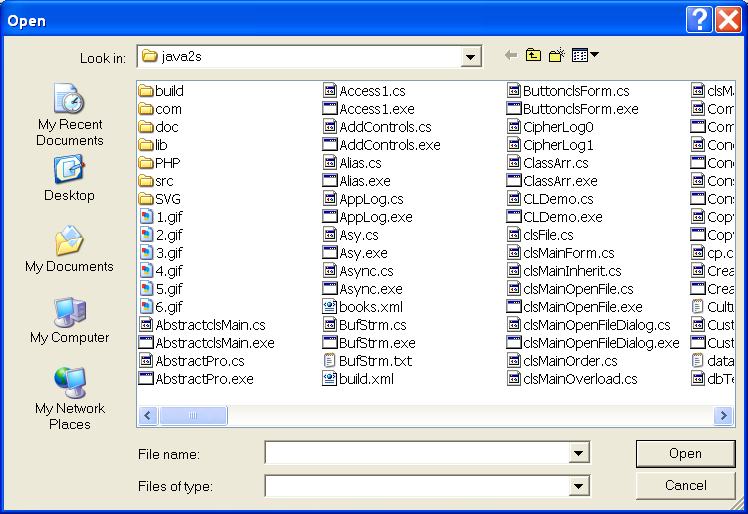 |
5. | illustrates the FileInfo class | | 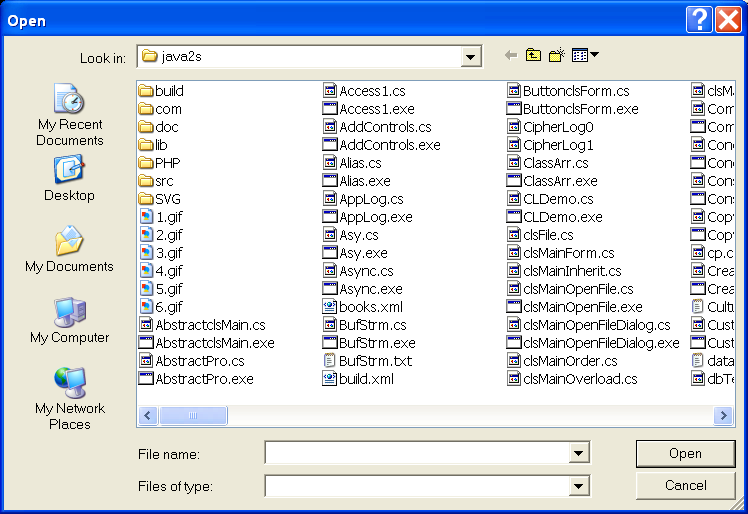 |
6. | illustrates the FileSystemWatcher class | | 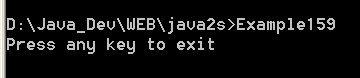 |
7. | File class to check whether a file exists, open and read | | |
8. | Uses methods in the File class to check whether a file exists | | |
9. | Uses methods in the File class to check the status of a file | | |
10. | File Class | | |
11. | File.AppendAllLines Method Appends lines to a file, and then closes the file. | | |
12. | File.AppendAllText Opens a file, appends the specified string to the file | | |
13. | File.AppendAllText Method Appends the string to the file, creating the file if it does not exist. | | |
14. | File.AppendText Creates a StreamWriter that appends UTF-8 encoded text to an existing file. | | |
15. | File.Copy Method Copies file to a new file | | |
16. | File.Create Creates or overwrites a file in the specified path. | | |
17. | File.Create Method (String, Int32) Creates or overwrites the specified file. | | |
18. | File.CreateText Method Creates or opens a file for writing UTF-8 encoded text. | | |
19. | File.Decrypt Decrypts a file | | |
20. | File.Exists Determines whether the specified file exists. | | |
21. | File.GetAccessControl Gets a FileSecurity object that encapsulates the access control list | | |
22. | File.GetAttributes Method Gets the FileAttributes of the file on the path. | | |
23. | File.GetCreationTime Method Returns the creation date and time of the specified file or directory. | | |
24. | File.GetLastAccessTime Method Returns the date and time the specified file or directory was last accessed. | | |
25. | File.GetLastWriteTime Returns the date and time the specified file or directory was last written to. | | |
26. | File.Move Moves a file to a new location | | |
27. | File.Open Opens a FileStream on the specified path with read/write access. | | |
28. | File.Open Method (String, FileMode, FileAccess) | | |
29. | File.Open Method (String, FileMode, FileAccess, FileShare) | | |
30. | File.OpenRead Method Opens an existing file for reading. | | |
31. | File.OpenText Method Opens an existing UTF-8 encoded text file for reading. | | |
32. | File.OpenWrite Method Opens an existing file or creates a new file for writing. | | |
33. | File.ReadAllLines Method Opens a text file, reads all lines of the file, and then closes the file. | | |
34. | File.ReadAllLines Method (String, Encoding) | | |
35. | File.Replace Replaces contents of file | | |
36. | File.SetLastAccessTime Sets the date and time the specified file was last accessed. | | |
37. | File.SetLastWriteTime | | |